ParCompMark::Host Class Reference
#include <PCMHost.h>
Inheritance diagram for ParCompMark::Host:
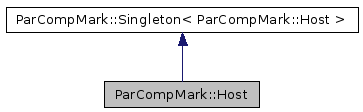
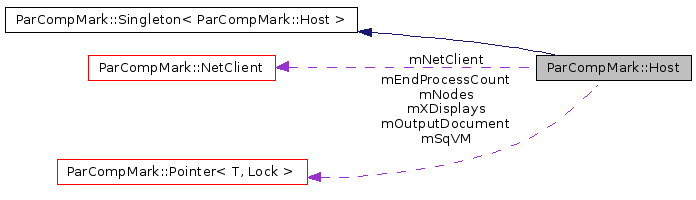
Detailed Description
Class for describe a hosts.
Definition at line 77 of file PCMHost.h.
Public Types | |
typedef ParCompMark::Pointer< int, Mutex > | IntPointer |
Type for pointer to an int. | |
Public Member Functions | |
Constructors & destructor | |
Host (const std::string &name="(unknown host)", NetClient *netClient=NULL) | |
Creates a host with a specified name. | |
virtual | ~Host () |
The destructor. | |
Getters & setters | |
const bool & | getWait () const |
Getter of mWait. | |
const std::string & | getName () const |
Getter of mName. | |
void | setName (const std::string &name) |
Setter of mName. | |
const std::string & | getLowLevelScript () const |
Getter of mLowLevelScript. | |
void | setLowLevelScript (const std::string &lowlevelscript) |
Setter of mLowLevelScript. | |
Container< Node, Mutex >::Pointer & | getNodes () |
Getter of mNodes. | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
OutputNode::Pointer & | getOutputDocument () |
Getter of mOutputDocument. | |
const PCid & | getSessionID () const |
Getter of mSessionID. | |
NetClient * | getNetClient () const |
Getter of mNetClient. | |
void | setNetClient (const NetClient *netclient) |
Setter of mNetClient. | |
const Host::IntPointer & | getEndProcessCount () const |
Getter of mEndProcessCount. | |
void | setEndProcessCount (const Host::IntPointer &endprocesscount) |
Setter of mEndProcessCount. | |
const int & | getProcessCount () const |
Getter of mProcessCount. | |
const int & | getID () const |
Getter of mID. | |
void | setID (const int &id) |
Setter of mID. | |
const Real & | getMessageSendingTime () const |
Getter of mMessageSendingTime. | |
void | setMessageSendingTime (const Real &messagesendingtime) |
Setter of mMessageSendingTime. | |
Methods | |
virtual XDisplay::Pointer | openXDisplay (const std::string &displayName="") |
Open an X display. | |
virtual XDisplay::Pointer | getFirstXDisplay () |
Return the first X Display on this host. | |
virtual void | openXDisplays () |
Automatically scan available X displays on this host and open them. | |
virtual void | closeXDisplays () |
Close all opened X displays. | |
virtual void | initialize () |
Initialize host from low level script. | |
virtual void | finalize () |
Finalize the host. | |
virtual Node * | createNode (const char *nodeName) |
Create node on this host. | |
virtual void | start () |
Start the nodes. | |
virtual u32 | stop () |
Stop the nodes. | |
virtual void | collectData () |
Collect data from nodes. | |
virtual void | setFrameID (const u32 &frameID) |
Set the ending frameID of processes. | |
Static Public Member Functions | |
Scripting binding | |
static void | squirrelGlue () |
Static glue code method for Squirrel-C++ binding. | |
Class methods | |
static Host * | getInstance () |
Gets the instance of the singleton class. | |
Static Public Attributes | |
Class constants | |
static const std::string | HOSTINITNUT = "scripts/framework/host-init.nut" |
Host initializer Squirrel script file (relative to the data directory). | |
Protected Attributes | |
Variables | |
bool | mWait |
Node wait?. | |
std::string | mName |
Name of the host (IP address). | |
SqVM::Pointer | mSqVM |
Squirrel virtual machine. | |
std::string | mLowLevelScript |
Squirrel low level script for initialization. | |
Container< XDisplay, Mutex >::Pointer | mXDisplays |
X Displays on this host. | |
Container< Node, Mutex >::Pointer | mNodes |
Nodes on this host. | |
bool | mInitialized |
The host is initialized. | |
OutputNode::Pointer | mOutputDocument |
Root of the output document on this host. | |
PCid | mSessionID |
PC session ID. | |
NetClient * | mNetClient |
Pointer to the netclietn class. | |
IntPointer | mEndProcessCount |
Count how many process end the rendering/compositing. | |
int | mProcessCount |
Count how many processes are on the host. | |
int | mID |
ID for the host. | |
Real | mMessageSendingTime |
Network message sendign time. |
Member Typedef Documentation
typedef ParCompMark::Pointer< int, Mutex > ParCompMark::Host::IntPointer |
Constructor & Destructor Documentation
ParCompMark::Host::Host | ( | const std::string & | name = "(unknown host)" , |
|
NetClient * | netClient = NULL | |||
) |
Creates a host with a specified name.
Definition at line 51 of file PCMHost.cpp.
References ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::getPtr(), mEndProcessCount, mInitialized, mLowLevelScript, mName, mNetClient, mProcessCount, mSessionID, mXDisplays, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::Host::~Host | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 90 of file PCMHost.cpp.
References finalize(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::kill(), mInitialized, mName, mXDisplays, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::Host::closeXDisplays | ( | ) | [virtual] |
Close all opened X displays.
Definition at line 171 of file PCMHost.cpp.
References mXDisplays.
Referenced by ParCompMark::Application::finalizeSoldier().
void ParCompMark::Host::collectData | ( | ) | [virtual] |
Collect data from nodes.
Definition at line 339 of file PCMHost.cpp.
References mNodes, and mOutputDocument.
Referenced by ParCompMark::Client::handleMessage().
Node * ParCompMark::Host::createNode | ( | const char * | nodeName | ) | [virtual] |
Create node on this host.
- Parameters:
-
[in] nodeName Name of the node (C string for squirrel compatibility).
- Returns:
- Pointer to the created node.
Definition at line 292 of file PCMHost.cpp.
References ParCompMark::Pointer< T, Lock >::getPtr(), ParCompMark::Pointer< T, Lock >::lock(), mNodes, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by squirrelGlue().
Here is the call graph for this function:

void ParCompMark::Host::finalize | ( | ) | [virtual] |
Finalize the host.
Definition at line 256 of file PCMHost.cpp.
References Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::isNotNull(), ParCompMark::Pointer< T, Lock >::kill(), mInitialized, mName, mNodes, mOutputDocument, mProcessCount, and mSqVM.
Referenced by initialize(), and ~Host().
Here is the call graph for this function:

const Host::IntPointer & ParCompMark::Host::getEndProcessCount | ( | ) | const [inline] |
Getter of mEndProcessCount.
Returns value of mEndProcessCount.
- Returns:
- The value of mEndProcessCount
Definition at line 616 of file PCMHost.h.
Referenced by ParCompMark::Process::threadFinalize().
XDisplay::Pointer ParCompMark::Host::getFirstXDisplay | ( | ) | [virtual] |
Return the first X Display on this host.
- Returns:
- The first display on this host, on null if there are no opened displays.
Definition at line 152 of file PCMHost.cpp.
References mXDisplays.
Referenced by ParCompMark::Application::setEnvironmentVariablesToSquirrel().
const int & ParCompMark::Host::getID | ( | ) | const [inline] |
const bool & ParCompMark::Host::getInitialized | ( | ) | const [inline] |
Host * ParCompMark::Host::getInstance | ( | ) | [static] |
Gets the instance of the singleton class.
Reimplemented for squirrel usage.
- Returns:
- Instance of the class
Reimplemented from ParCompMark::Singleton< T >.
Definition at line 108 of file PCMHost.cpp.
Referenced by ParCompMark::Application::finalizeSoldier(), ParCompMark::Client::handleMessage(), ParCompMark::Application::setEnvironmentVariablesToSquirrel(), ParCompMark::Application::soldierOperation(), squirrelGlue(), and ParCompMark::Process::threadFinalize().
const std::string & ParCompMark::Host::getLowLevelScript | ( | ) | const [inline] |
const Real & ParCompMark::Host::getMessageSendingTime | ( | ) | const [inline] |
const std::string & ParCompMark::Host::getName | ( | ) | const [inline] |
NetClient * ParCompMark::Host::getNetClient | ( | ) | const [inline] |
OutputNode::Pointer & ParCompMark::Host::getOutputDocument | ( | ) | [inline] |
Getter of mOutputDocument.
Returns value of mOutputDocument.
- Returns:
- The value of mOutputDocument
Definition at line 588 of file PCMHost.h.
Referenced by ParCompMark::Client::handleMessage().
const int & ParCompMark::Host::getProcessCount | ( | ) | const [inline] |
Getter of mProcessCount.
Returns value of mProcessCount.
- Returns:
- The value of mProcessCount
Definition at line 630 of file PCMHost.h.
Referenced by ParCompMark::Process::threadFinalize().
const PCid & ParCompMark::Host::getSessionID | ( | ) | const [inline] |
const bool & ParCompMark::Host::getWait | ( | ) | const [inline] |
void ParCompMark::Host::initialize | ( | ) | [virtual] |
Initialize host from low level script.
Definition at line 181 of file PCMHost.cpp.
References ParCompMark::Logger::DEBUG, finalize(), ParCompMark::FileSystemManager::getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::getPtr(), HOSTINITNUT, ParCompMark::OutputNode::INFORMATION, ParCompMark::Pointer< T, Lock >::lock(), mEndProcessCount, mInitialized, mLowLevelScript, mName, mNodes, mOutputDocument, mProcessCount, mSessionID, mSqVM, ParCompMark::SqVM::NOMAINMETHOD, ParCompMark::Application::PARCOMPMARKNUT, ParCompMark::Application::PCAPINUT, ParCompMark::Timer::setTimeCorrection(), ParCompMark::StringConverter::toString(), ParCompMark::Pointer< T, Lock >::unlock(), and ParCompMark::Application::UTILSNUT.
Referenced by ParCompMark::Client::handleMessage().
Here is the call graph for this function:
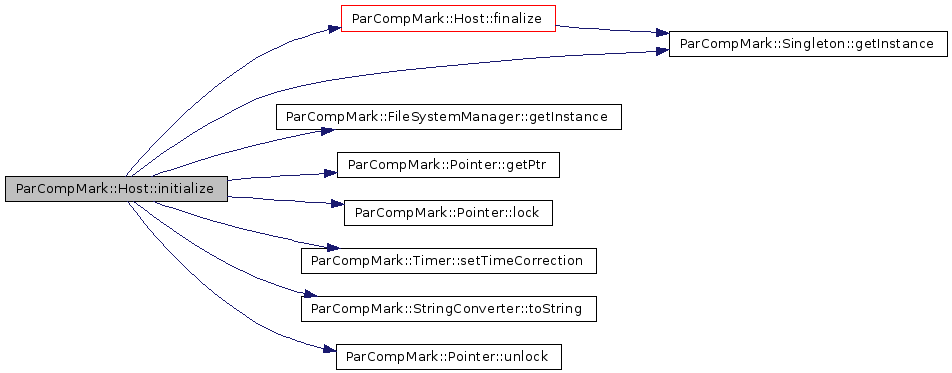
XDisplay::Pointer ParCompMark::Host::openXDisplay | ( | const std::string & | displayName = "" |
) | [virtual] |
Open an X display.
An X display can be opened several times, and does not have to be closed. The host will close it when it is destructed. When no parameter is set, the default display will be opened.
- Parameters:
-
[in] displayName X display name
- Returns:
- Opened X Display
Definition at line 119 of file PCMHost.cpp.
References ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::lock(), mName, mXDisplays, ParCompMark::Logger::NOTICE, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by openXDisplays().
Here is the call graph for this function:
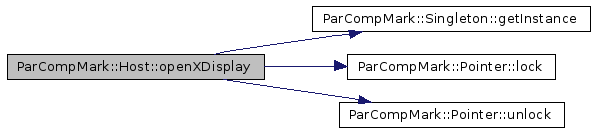
void ParCompMark::Host::openXDisplays | ( | ) | [virtual] |
Automatically scan available X displays on this host and open them.
Definition at line 163 of file PCMHost.cpp.
References openXDisplay().
Referenced by ParCompMark::Application::soldierOperation().
Here is the call graph for this function:

void ParCompMark::Host::setEndProcessCount | ( | const Host::IntPointer & | endprocesscount | ) | [inline] |
void ParCompMark::Host::setFrameID | ( | const u32 & | frameID | ) | [virtual] |
Set the ending frameID of processes.
- Parameters:
-
[in] frameID StopID.
Definition at line 350 of file PCMHost.cpp.
References mNodes.
Referenced by ParCompMark::Client::handleMessage().
void ParCompMark::Host::setID | ( | const int & | id | ) | [inline] |
Setter of mID.
Sets value of mID.
- Parameters:
-
[in] id The value of mID
Definition at line 644 of file PCMHost.h.
Referenced by ParCompMark::Client::handleMessage().
void ParCompMark::Host::setLowLevelScript | ( | const std::string & | lowlevelscript | ) | [inline] |
Setter of mLowLevelScript.
Sets value of mLowLevelScript.
- Parameters:
-
[in] lowlevelscript The value of mLowLevelScript
Definition at line 566 of file PCMHost.h.
Referenced by ParCompMark::Client::handleMessage().
void ParCompMark::Host::setMessageSendingTime | ( | const Real & | messagesendingtime | ) | [inline] |
Setter of mMessageSendingTime.
Sets value of mMessageSendingTime.
- Parameters:
-
[in] messagesendingtime The value of mMessageSendingTime
Definition at line 658 of file PCMHost.h.
Referenced by ParCompMark::Client::handleMessage().
void ParCompMark::Host::setName | ( | const std::string & | name | ) | [inline] |
Setter of mName.
Sets value of mName.
- Parameters:
-
[in] name The value of mName
Definition at line 552 of file PCMHost.h.
Referenced by ParCompMark::Client::handleMessage().
void ParCompMark::Host::setNetClient | ( | const NetClient * | netclient | ) | [inline] |
Setter of mNetClient.
Sets value of mNetClient.
- Parameters:
-
[in] netclient The value of mNetClient
Definition at line 609 of file PCMHost.h.
Referenced by ParCompMark::Application::soldierOperation().
static void ParCompMark::Host::squirrelGlue | ( | ) | [inline, static] |
Static glue code method for Squirrel-C++ binding.
This method should be call from each Squirrel virtual machines to generate the proper Squirrel glue code. To content of this method is fully autogenerated in the header implementation file, you should not modify it.
Definition at line 101 of file PCMHost.h.
References createNode(), and getInstance().
Referenced by ParCompMark::squirrelClassBindings().
Here is the call graph for this function:

void ParCompMark::Host::start | ( | ) | [virtual] |
Start the nodes.
Definition at line 307 of file PCMHost.cpp.
References ParCompMark::Singleton< T >::getInstance(), mName, mNodes, and ParCompMark::Logger::NOTICE.
Referenced by ParCompMark::Client::handleMessage().
Here is the call graph for this function:

u32 ParCompMark::Host::stop | ( | ) | [virtual] |
Stop the nodes.
- Returns:
- frameID
Definition at line 318 of file PCMHost.cpp.
References ParCompMark::Singleton< T >::getInstance(), mName, mNodes, and ParCompMark::Logger::NOTICE.
Referenced by ParCompMark::Client::handleMessage().
Here is the call graph for this function:

Member Data Documentation
const std::string ParCompMark::Host::HOSTINITNUT = "scripts/framework/host-init.nut" [static] |
Host initializer Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 134 of file PCMHost.h.
Referenced by initialize().
IntPointer ParCompMark::Host::mEndProcessCount [protected] |
Count how many process end the rendering/compositing.
- Remarks:
- This is own attribute of this class.
Definition at line 222 of file PCMHost.h.
Referenced by Host(), and initialize().
int ParCompMark::Host::mID [protected] |
bool ParCompMark::Host::mInitialized [protected] |
The host is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 194 of file PCMHost.h.
Referenced by finalize(), Host(), initialize(), and ~Host().
std::string ParCompMark::Host::mLowLevelScript [protected] |
Squirrel low level script for initialization.
- Remarks:
- This is own attribute of this class.
Definition at line 173 of file PCMHost.h.
Referenced by Host(), and initialize().
Real ParCompMark::Host::mMessageSendingTime [protected] |
std::string ParCompMark::Host::mName [protected] |
Name of the host (IP address).
- Remarks:
- This is own attribute of this class.
Definition at line 159 of file PCMHost.h.
Referenced by finalize(), Host(), initialize(), openXDisplay(), start(), stop(), and ~Host().
NetClient* ParCompMark::Host::mNetClient [protected] |
Container< Node, Mutex >::Pointer ParCompMark::Host::mNodes [protected] |
Nodes on this host.
- Remarks:
- This is own attribute of this class.
Definition at line 187 of file PCMHost.h.
Referenced by collectData(), createNode(), finalize(), initialize(), setFrameID(), start(), and stop().
Root of the output document on this host.
- Remarks:
- This is own attribute of this class.
Definition at line 201 of file PCMHost.h.
Referenced by collectData(), finalize(), and initialize().
int ParCompMark::Host::mProcessCount [protected] |
Count how many processes are on the host.
- Remarks:
- This is own attribute of this class.
Definition at line 229 of file PCMHost.h.
Referenced by finalize(), Host(), and initialize().
PCid ParCompMark::Host::mSessionID [protected] |
PC session ID.
- Remarks:
- This is own attribute of this class.
Definition at line 208 of file PCMHost.h.
Referenced by Host(), and initialize().
SqVM::Pointer ParCompMark::Host::mSqVM [protected] |
Squirrel virtual machine.
- Remarks:
- This is own attribute of this class.
Definition at line 166 of file PCMHost.h.
Referenced by finalize(), and initialize().
bool ParCompMark::Host::mWait [protected] |
Container< XDisplay, Mutex >::Pointer ParCompMark::Host::mXDisplays [protected] |
X Displays on this host.
- Remarks:
- This is own attribute of this class.
Definition at line 180 of file PCMHost.h.
Referenced by closeXDisplays(), getFirstXDisplay(), Host(), openXDisplay(), and ~Host().
The documentation for this class was generated from the following files: