ParCompMark::OutputNode Class Reference
#include <PCMOutputNode.h>
Inheritance diagram for ParCompMark::OutputNode:
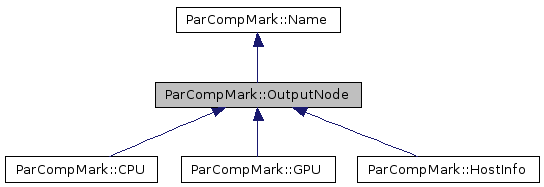
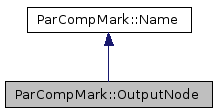
Detailed Description
Node of the output document.
Definition at line 62 of file PCMOutputNode.h.
Getters & setters | |
const OutputNode::NodeType & | getType () const |
Getter of mType. | |
const std::string & | getText () const |
Getter of mText. | |
void | setText (const std::string &text) |
Setter of mText. | |
static const std::string & | getEXTNODENAME () |
Getter of TEXTNODENAME. | |
Class methods | |
static OutputNode::Pointer | parseFromXML (std::string &xmlDocument) |
Parse XML document and construct its OutputNode equivalent. | |
static bool | _testXMLName (const std::string &name) |
Test name if its a correct xml name. | |
static std::string | _convertSpecialChars (const std::string &string) |
Convert special characters to XML entites. | |
Public Types | |
typedef Pointer< OutputNode, Mutex > | Pointer |
Type for pointer on this class. | |
typedef std::list< OutputNode::Pointer > | ChildNodeList |
Type definition for list of child nodes. | |
typedef ChildNodeList::iterator | ChildNodeListIterator |
Type definition for iterator on list of child nodes. | |
typedef std::map< std::string, std::string > | AttributeMap |
Type definition for map of attributes. | |
typedef AttributeMap::const_iterator | AttributeMapIterator |
Type definition for iterator on map of attributes. | |
DEFINITION | |
Define something (cluster, host, node, etc. | |
INFORMATION | |
Information about the hardware or software element. | |
REFERENCE | |
A reference, new element cannot be defined, only referencing an existing element is possible. | |
STATISTICS | |
Statistics, it cannot be defined, referenced, or determined from the hardware directly. | |
TEXT | |
The node is a text field. | |
CDATA | |
The node is a CDATA text field. | |
enum | NodeType { DEFINITION, INFORMATION, REFERENCE, STATISTICS, TEXT, CDATA } |
Node type definitions. More... | |
Public Member Functions | |
Constructors & destructor | |
OutputNode (const std::string &name, const NodeType &type) | |
Creates output node. | |
OutputNode (const std::string &text) | |
Creates text field. | |
virtual | ~OutputNode () |
The destructor. | |
Methods | |
virtual OutputNode::Pointer | createChildNode (const std::string &name, const NodeType &type) |
Create nontextual child node. | |
virtual OutputNode::Pointer | createChildNode (const std::string &text) |
Create textual child node. | |
virtual void | addChildNode (OutputNode::Pointer child) |
Add child node. | |
virtual u32 | getChildCount () |
Return the number of child nodes. | |
virtual OutputNode::Pointer | getFirstChildNode () |
Return first child node. | |
virtual bool | hasAttribute (const std::string &attribute) const |
Return true if the node has attribute with the specified name. | |
virtual const std::string & | getAttribute (const std::string &attribute) const |
Get an attribute by name. | |
virtual void | setAttribute (const std::string &attribute, const std::string &value) |
Set attribute, also create if does not exists, overwrite otherwise. | |
virtual std::ostringstream & | serialize2XML (std::ostringstream &osstr) |
Serialize the node to XML (this is a recursive method, calls the same methods of the children too). | |
virtual std::string | serialize2XML () |
Serialize the node to XML (this is a recursive method, calls the same methods of the children too). | |
virtual void | refreshData () |
Refresh datas. | |
virtual void | clean () |
Clean outputnode. | |
Protected Attributes | |
Variables | |
NodeType | mType |
Type of the node. | |
AttributeMap | mAttributes |
Attributes of the node. | |
ChildNodeList | mChildren |
Child nodes. | |
std::string | mText |
Text data. | |
Static Protected Attributes | |
Class constants | |
static const std::string | TEXTNODENAME = "#text" |
String constant for node name for text data nodes. |
Member Typedef Documentation
typedef std::map< std::string, std::string > ParCompMark::OutputNode::AttributeMap |
typedef AttributeMap::const_iterator ParCompMark::OutputNode::AttributeMapIterator |
typedef std::list< OutputNode::Pointer > ParCompMark::OutputNode::ChildNodeList |
typedef ChildNodeList::iterator ParCompMark::OutputNode::ChildNodeListIterator |
Type definition for iterator on list of child nodes.
Definition at line 115 of file PCMOutputNode.h.
typedef Pointer< OutputNode, Mutex > ParCompMark::OutputNode::Pointer |
Type for pointer on this class.
Reimplemented in ParCompMark::CPU, ParCompMark::GPU, and ParCompMark::HostInfo.
Definition at line 109 of file PCMOutputNode.h.
Member Enumeration Documentation
Node type definitions.
- Enumerator:
Definition at line 79 of file PCMOutputNode.h.
Constructor & Destructor Documentation
ParCompMark::OutputNode::OutputNode | ( | const std::string & | name, | |
const NodeType & | type | |||
) |
Creates output node.
- Parameters:
-
[in] name Name of the node. [in] type Type of the node.
Definition at line 50 of file PCMOutputNode.cpp.
References _testXMLName(), and Assert.
Referenced by createChildNode().
Here is the call graph for this function:

ParCompMark::OutputNode::OutputNode | ( | const std::string & | text | ) |
Creates text field.
- Parameters:
-
[in] text Text data.
Definition at line 70 of file PCMOutputNode.cpp.
ParCompMark::OutputNode::~OutputNode | ( | ) | [virtual] |
Member Function Documentation
std::string ParCompMark::OutputNode::_convertSpecialChars | ( | const std::string & | string | ) | [static, protected] |
Convert special characters to XML entites.
- Parameters:
-
[in] string String to convert.
- Returns:
- Converted string
Definition at line 125 of file PCMOutputNode.cpp.
Referenced by serialize2XML().
bool ParCompMark::OutputNode::_testXMLName | ( | const std::string & | name | ) | [static, protected] |
Test name if its a correct xml name.
- Parameters:
-
[in] name Name to test.
- Returns:
- True if the specified name is a correct XML name
Definition at line 116 of file PCMOutputNode.cpp.
Referenced by OutputNode(), and setAttribute().
void ParCompMark::OutputNode::addChildNode | ( | OutputNode::Pointer | child | ) | [virtual] |
Add child node.
- Parameters:
-
[in] child Child node.
Definition at line 166 of file PCMOutputNode.cpp.
References Assert, CDATA, ParCompMark::Pointer< T, Lock >::getPtr(), mChildren, mType, and TEXT.
Here is the call graph for this function:

void ParCompMark::OutputNode::clean | ( | ) | [virtual] |
OutputNode::Pointer ParCompMark::OutputNode::createChildNode | ( | const std::string & | text | ) | [virtual] |
Create textual child node.
- Parameters:
-
[in] text Text data.
- Returns:
- Created child node.
Definition at line 152 of file PCMOutputNode.cpp.
References Assert, CDATA, mChildren, mType, OutputNode(), and TEXT.
Here is the call graph for this function:

OutputNode::Pointer ParCompMark::OutputNode::createChildNode | ( | const std::string & | name, | |
const NodeType & | type | |||
) | [virtual] |
Create nontextual child node.
- Parameters:
-
[in] name Name of the child node. [in] type Type of the child node.
- Returns:
- Created child node.
Definition at line 138 of file PCMOutputNode.cpp.
References Assert, CDATA, mChildren, mType, OutputNode(), and TEXT.
Referenced by ParCompMark::HostInfo::refreshData(), and ParCompMark::GPU::refreshData().
Here is the call graph for this function:

const std::string & ParCompMark::OutputNode::getAttribute | ( | const std::string & | attribute | ) | const [virtual] |
Get an attribute by name.
- Parameters:
-
[in] attribute Name of the attribute.
- Returns:
- Attribute value.
Definition at line 203 of file PCMOutputNode.cpp.
References Assert, CDATA, mAttributes, mType, and TEXT.
u32 ParCompMark::OutputNode::getChildCount | ( | ) | [virtual] |
Return the number of child nodes.
- Returns:
- The number of child nodes.
Definition at line 178 of file PCMOutputNode.cpp.
References mChildren.
const std::string & ParCompMark::OutputNode::getEXTNODENAME | ( | ) | [inline, static] |
Getter of TEXTNODENAME.
Returns value of TEXTNODENAME.
- Returns:
- The value of TEXTNODENAME
Definition at line 408 of file PCMOutputNode.h.
OutputNode::Pointer ParCompMark::OutputNode::getFirstChildNode | ( | ) | [virtual] |
const std::string & ParCompMark::OutputNode::getText | ( | ) | const [inline] |
Getter of mText.
Returns value of mText.
- Returns:
- The value of mText
Definition at line 422 of file PCMOutputNode.h.
const OutputNode::NodeType & ParCompMark::OutputNode::getType | ( | ) | const [inline] |
Getter of mType.
Returns value of mType.
- Returns:
- The value of mType
Definition at line 415 of file PCMOutputNode.h.
bool ParCompMark::OutputNode::hasAttribute | ( | const std::string & | attribute | ) | const [virtual] |
Return true if the node has attribute with the specified name.
- Parameters:
-
[in] attribute Name of the attribute.
- Returns:
- The node has the specified attribute.
Definition at line 194 of file PCMOutputNode.cpp.
References Assert, CDATA, mAttributes, mType, and TEXT.
OutputNode::Pointer ParCompMark::OutputNode::parseFromXML | ( | std::string & | xmlDocument | ) | [static] |
Parse XML document and construct its OutputNode equivalent.
- Parameters:
-
[out] xmlDocument XML document or fragment to parse.
- Returns:
- Root node of the parsed document
Definition at line 102 of file PCMOutputNode.cpp.
void ParCompMark::OutputNode::refreshData | ( | ) | [virtual] |
Refresh datas.
Reimplemented in ParCompMark::CPU, ParCompMark::GPU, and ParCompMark::HostInfo.
Definition at line 302 of file PCMOutputNode.cpp.
std::string ParCompMark::OutputNode::serialize2XML | ( | ) | [virtual] |
Serialize the node to XML (this is a recursive method, calls the same methods of the children too).
Use the other version of this method with parameter std::ostringstream if it is possible for memory saving.
- Returns:
- Serialized node.
Definition at line 294 of file PCMOutputNode.cpp.
std::ostringstream & ParCompMark::OutputNode::serialize2XML | ( | std::ostringstream & | osstr | ) | [virtual] |
Serialize the node to XML (this is a recursive method, calls the same methods of the children too).
- Parameters:
-
[out] osstr Output string stream.
- Returns:
- Serialized node (added to osstr).
Definition at line 228 of file PCMOutputNode.cpp.
References _convertSpecialChars(), Assert, CDATA, DEFINITION, INFORMATION, mAttributes, mChildren, ParCompMark::Name::mName, mText, mType, REFERENCE, STATISTICS, and TEXT.
Here is the call graph for this function:

void ParCompMark::OutputNode::setAttribute | ( | const std::string & | attribute, | |
const std::string & | value | |||
) | [virtual] |
Set attribute, also create if does not exists, overwrite otherwise.
- Parameters:
-
[in] attribute Name of the attribute. [in] value Value of the attribute.
Definition at line 217 of file PCMOutputNode.cpp.
References _testXMLName(), Assert, CDATA, mAttributes, mType, and TEXT.
Referenced by ParCompMark::HostInfo::HostInfo().
Here is the call graph for this function:

void ParCompMark::OutputNode::setText | ( | const std::string & | text | ) | [inline] |
Setter of mText.
Sets value of mText.
- Parameters:
-
[in] text The value of mText
Definition at line 429 of file PCMOutputNode.h.
Member Data Documentation
AttributeMap ParCompMark::OutputNode::mAttributes [protected] |
Attributes of the node.
- Remarks:
- This is own attribute of this class.
Definition at line 163 of file PCMOutputNode.h.
Referenced by getAttribute(), hasAttribute(), serialize2XML(), and setAttribute().
ChildNodeList ParCompMark::OutputNode::mChildren [protected] |
Child nodes.
- Remarks:
- This is own attribute of this class.
Definition at line 170 of file PCMOutputNode.h.
Referenced by addChildNode(), clean(), createChildNode(), getChildCount(), getFirstChildNode(), and serialize2XML().
std::string ParCompMark::OutputNode::mText [protected] |
Text data.
- Remarks:
- This is own attribute of this class.
Definition at line 177 of file PCMOutputNode.h.
Referenced by serialize2XML(), ParCompMarkTest::TestOutputNode::test_constructor_cstd__string(), and ParCompMarkTest::TestOutputNode::test_constructor_cstd__string_cNodeType().
NodeType ParCompMark::OutputNode::mType [protected] |
Type of the node.
- Remarks:
- This is own attribute of this class.
Definition at line 156 of file PCMOutputNode.h.
Referenced by addChildNode(), createChildNode(), getAttribute(), getFirstChildNode(), hasAttribute(), serialize2XML(), setAttribute(), ParCompMarkTest::TestOutputNode::test_constructor_cstd__string(), and ParCompMarkTest::TestOutputNode::test_constructor_cstd__string_cNodeType().
const std::string ParCompMark::OutputNode::TEXTNODENAME = "#text" [static, protected] |
String constant for node name for text data nodes.
- Remarks:
- This is own attribute of this class.
Definition at line 138 of file PCMOutputNode.h.
The documentation for this class was generated from the following files: