ParCompMark::Container< ElementType, LockType > Class Template Reference
#include <PCMContainer.h>
Inheritance diagram for ParCompMark::Container< ElementType, LockType >:
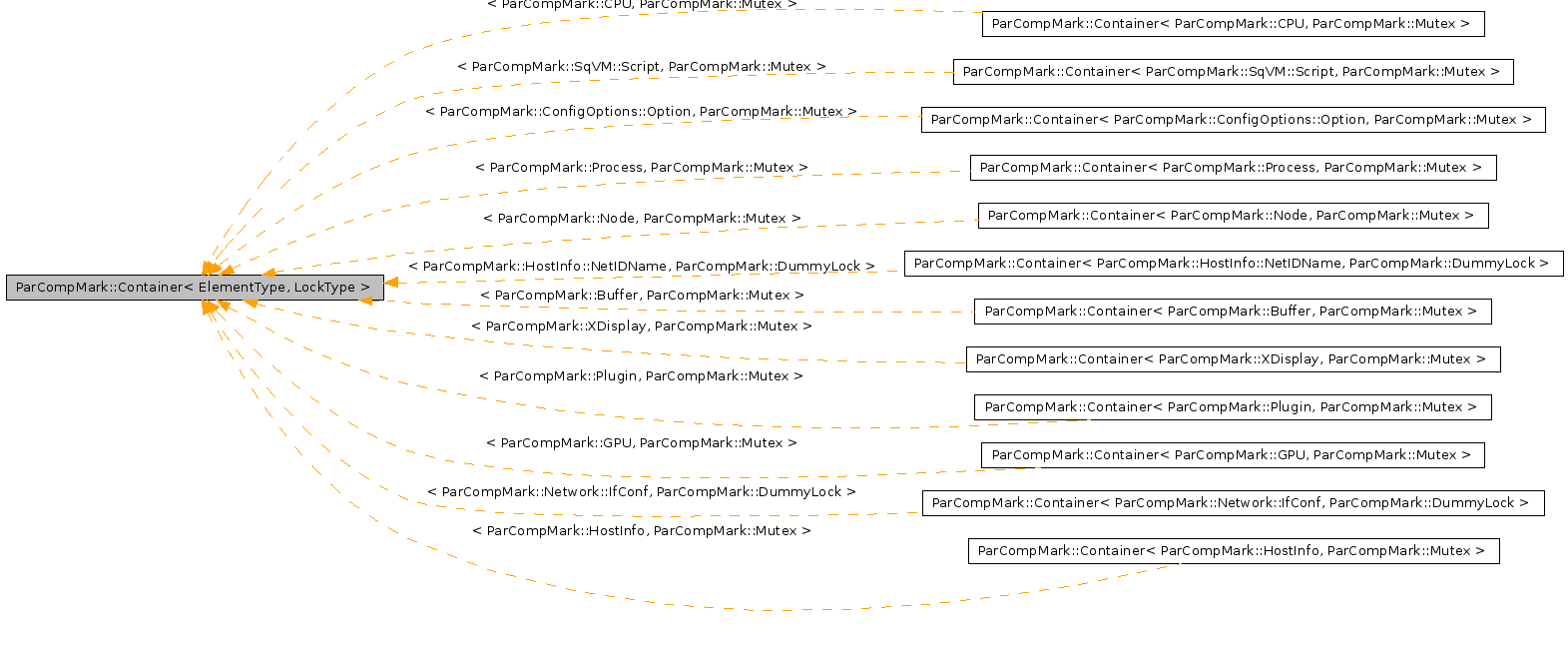
Detailed Description
template<class ElementType, class LockType>
class ParCompMark::Container< ElementType, LockType >
String addressed map of typed smart pointers.
Definition at line 55 of file PCMContainer.h.
Public Types | |
typedef Pointer< Container< ElementType, LockType >, LockType > | Pointer |
Type for pointer on this class. | |
typedef ElementType::Pointer | ElementPointer |
Type definition for pointer on elements. | |
typedef std::map< std::string, ElementPointer > | ElementsMap |
Type definition for map of elements. | |
typedef ElementsMap::iterator | Iterator |
Type definition for iterator on map of elements. | |
Public Member Functions | |
Constructors & destructor | |
Container () | |
Default constructor. | |
virtual | ~Container () |
The destructor. | |
Methods | |
virtual void | add (std::string name, ElementPointer element) |
Add an element. | |
virtual ElementPointer | get (const std::string &name) |
Get an element by name. | |
virtual bool | has (const std::string &name) |
Search for an element by name. | |
virtual void | remove (const std::string &name) |
Remove an element by name. | |
virtual u32 | getSize () |
Return the number of elements. | |
virtual bool | isEmpty () |
Return true if the container is empty. | |
virtual Iterator | begin () |
Begin iterator on the container. | |
virtual Iterator | end () |
End iterator on the container. | |
Protected Attributes | |
Variables | |
ElementsMap | mElements |
Map of elements. |
Member Typedef Documentation
typedef ElementType::Pointer ParCompMark::Container< ElementType, LockType >::ElementPointer |
typedef std::map< std::string, ElementPointer > ParCompMark::Container< ElementType, LockType >::ElementsMap |
typedef ElementsMap::iterator ParCompMark::Container< ElementType, LockType >::Iterator |
typedef Pointer< Container < ElementType, LockType >, LockType > ParCompMark::Container< ElementType, LockType >::Pointer |
Constructor & Destructor Documentation
ParCompMark::Container< ElementType, LockType >::Container | ( | ) | [inline] |
ParCompMark::Container< ElementType, LockType >::~Container | ( | ) | [inline, virtual] |
Member Function Documentation
void ParCompMark::Container< ElementType, LockType >::add | ( | std::string | name, | |
ElementPointer | element | |||
) | [virtual] |
Add an element.
- Parameters:
-
[in] name Name of the element [in] element Element
Definition at line 245 of file PCMContainer.h.
std::map< std::string, typename ElementType::Pointer >::iterator ParCompMark::Container< ElementType, LockType >::begin | ( | ) | [virtual] |
Begin iterator on the container.
- Returns:
- Iterator on the first element.
Definition at line 294 of file PCMContainer.h.
std::map< std::string, typename ElementType::Pointer >::iterator ParCompMark::Container< ElementType, LockType >::end | ( | ) | [virtual] |
End iterator on the container.
- Returns:
- Iterator on the element after the last element.
Definition at line 302 of file PCMContainer.h.
ElementType::Pointer ParCompMark::Container< ElementType, LockType >::get | ( | const std::string & | name | ) | [virtual] |
Get an element by name.
- Parameters:
-
[in] name Name of the element
- Returns:
- Pointer to the element
Definition at line 254 of file PCMContainer.h.
u32 ParCompMark::Container< ElementType, LockType >::getSize | ( | ) | [virtual] |
Return the number of elements.
- Returns:
- Pointer to the element
Definition at line 279 of file PCMContainer.h.
bool ParCompMark::Container< ElementType, LockType >::has | ( | const std::string & | name | ) | [virtual] |
Search for an element by name.
- Parameters:
-
[in] name Name of the element
- Returns:
- The container has the element.
Definition at line 263 of file PCMContainer.h.
bool ParCompMark::Container< ElementType, LockType >::isEmpty | ( | ) | [virtual] |
Return true if the container is empty.
- Returns:
- True if the container is empty
Definition at line 286 of file PCMContainer.h.
void ParCompMark::Container< ElementType, LockType >::remove | ( | const std::string & | name | ) | [virtual] |
Remove an element by name.
- Parameters:
-
[in] name Name of the element
Definition at line 271 of file PCMContainer.h.
Member Data Documentation
ElementsMap ParCompMark::Container< ElementType, LockType >::mElements [protected] |
Map of elements.
- Remarks:
- This is own attribute of this class.
Definition at line 100 of file PCMContainer.h.
The documentation for this class was generated from the following file: