ParCompMark::Logger Class Reference
#include <PCMLogger.h>
Inheritance diagram for ParCompMark::Logger:
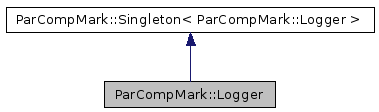
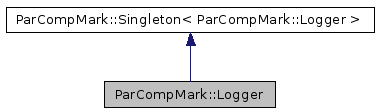
Detailed Description
Class for very simple logging mechanism.
Definition at line 60 of file PCMLogger.h.
Public Types | |
FATAL | |
Fatal error. | |
ERROR | |
Error. | |
WARNING | |
Warning. | |
NOTICE | |
Notice. | |
DEBUG | |
Debug. | |
enum | LogLevel { FATAL, ERROR, WARNING, NOTICE, DEBUG } |
Definitions logging levels. More... | |
Public Member Functions | |
Constructors & destructor | |
Logger (const std::string &logFileName="parcompmark.log") | |
Create logger. | |
virtual | ~Logger () |
The destructor. | |
Getters & setters | |
const u8 & | getLogMode () const |
Getter of mLogMode. | |
const Logger::LogLevel & | getConsoleLogLevel () const |
Getter of mConsoleLogLevel. | |
void | setConsoleLogLevel (const Logger::LogLevel &consoleloglevel) |
Setter of mConsoleLogLevel. | |
const Logger::LogLevel & | getFileLogLevel () const |
Getter of mFileLogLevel. | |
void | setFileLogLevel (const Logger::LogLevel &fileloglevel) |
Setter of mFileLogLevel. | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
const std::string & | getLogFileName () const |
Getter of mLogFileName. | |
void | setLogFileName (const std::string &logfilename) |
Setter of mLogFileName. | |
Methods | |
virtual void | initialize () |
Initializes the logger. | |
virtual void | log (const LogLevel &loglevel, const std::string &message) |
Logs a message. | |
virtual void | logMultiLine (const LogLevel &loglevel, const std::string &message) |
Logs a message. | |
virtual void | log (const Exception &exception) |
Logs an exception. | |
Static Public Member Functions | |
Class methods | |
static std::string | translateLogLevel (const LogLevel &loglevel) |
Translate log level to human readable. | |
static std::string | translateException (const Exception &exception) |
Translate an exception to human readable. | |
Static Public Attributes | |
Class constants | |
static const u8 | LOGTOCONSOLE = 1 |
Indicates that the logger logs to console. | |
static const u8 | LOGTOFILE = 2 |
Indicates that the logger logs to a textfile. | |
Protected Attributes | |
Variables | |
u8 | mLogMode |
Logging mode (console and/or textfile). | |
LogLevel | mConsoleLogLevel |
Logging level for console (from FATAL to NOTICE). | |
LogLevel | mFileLogLevel |
Logging level for file (from FATAL to NOTICE). | |
Pointer< FILE, Mutex > | mFp |
File pointer. | |
bool | mInitialized |
Indicates that the logger is initialized. | |
std::string | mLogFileName |
Name of the log file. |
Member Enumeration Documentation
Constructor & Destructor Documentation
ParCompMark::Logger::Logger | ( | const std::string & | logFileName = "parcompmark.log" |
) |
Create logger.
- Parameters:
-
[in] logFileName Name of the log file In the application directory.
Definition at line 52 of file PCMLogger.cpp.
References DEBUG, ParCompMark::Network::getIfConfs(), ParCompMark::Network::getIP(), LOGTOCONSOLE, LOGTOFILE, mConsoleLogLevel, mFileLogLevel, mFp, mInitialized, mLogFileName, mLogMode, and WARNING.
Here is the call graph for this function:

ParCompMark::Logger::~Logger | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 72 of file PCMLogger.cpp.
References ParCompMark::FileSystemManager::closeFile(), log(), mFp, mInitialized, and NOTICE.
Here is the call graph for this function:

Member Function Documentation
const Logger::LogLevel & ParCompMark::Logger::getConsoleLogLevel | ( | ) | const [inline] |
Getter of mConsoleLogLevel.
Returns value of mConsoleLogLevel.
- Returns:
- The value of mConsoleLogLevel
Definition at line 368 of file PCMLogger.h.
const Logger::LogLevel & ParCompMark::Logger::getFileLogLevel | ( | ) | const [inline] |
Getter of mFileLogLevel.
Returns value of mFileLogLevel.
- Returns:
- The value of mFileLogLevel
Definition at line 382 of file PCMLogger.h.
const bool & ParCompMark::Logger::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 396 of file PCMLogger.h.
const std::string & ParCompMark::Logger::getLogFileName | ( | ) | const [inline] |
Getter of mLogFileName.
Returns value of mLogFileName.
- Returns:
- The value of mLogFileName
Definition at line 403 of file PCMLogger.h.
const u8 & ParCompMark::Logger::getLogMode | ( | ) | const [inline] |
Getter of mLogMode.
Returns value of mLogMode.
- Returns:
- The value of mLogMode
Definition at line 361 of file PCMLogger.h.
void ParCompMark::Logger::initialize | ( | ) | [virtual] |
Initializes the logger.
Definition at line 107 of file PCMLogger.cpp.
References Assert, ParCompMark::Singleton< T >::createInstance(), ParCompMark::FileSystemManager::getInstance(), ParCompMark::Timer::getTimeDateString(), ParCompMark::FileSystemManager::initialize(), log(), LOGTOFILE, mFp, mInitialized, mLogFileName, mLogMode, NOTICE, ParCompMark::FileSystemManager::openAppFileC(), and ParCompMark::FileSystemManager::WRITE.
Here is the call graph for this function:
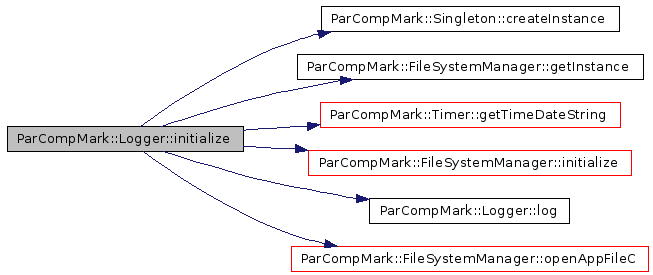
void ParCompMark::Logger::log | ( | const Exception & | exception | ) | [inline, virtual] |
Logs an exception.
- Parameters:
-
[in] exception Exception.
Definition at line 491 of file PCMLogger.h.
void ParCompMark::Logger::log | ( | const LogLevel & | loglevel, | |
const std::string & | message | |||
) | [inline, virtual] |
Logs a message.
(Does not care about multiline messages, because of performance).
- Parameters:
-
[in] loglevel Message level. [in] message Message string.
Definition at line 453 of file PCMLogger.h.
Referenced by initialize(), logMultiLine(), and ~Logger().
void ParCompMark::Logger::logMultiLine | ( | const LogLevel & | loglevel, | |
const std::string & | message | |||
) | [virtual] |
Logs a message.
Handles multiline messages too.
- Parameters:
-
[in] loglevel Message level. [in] message Message string.
Definition at line 129 of file PCMLogger.cpp.
References log().
Here is the call graph for this function:

void ParCompMark::Logger::setConsoleLogLevel | ( | const Logger::LogLevel & | consoleloglevel | ) | [inline] |
Setter of mConsoleLogLevel.
Sets value of mConsoleLogLevel.
- Parameters:
-
[in] consoleloglevel The value of mConsoleLogLevel
Definition at line 375 of file PCMLogger.h.
void ParCompMark::Logger::setFileLogLevel | ( | const Logger::LogLevel & | fileloglevel | ) | [inline] |
Setter of mFileLogLevel.
Sets value of mFileLogLevel.
- Parameters:
-
[in] fileloglevel The value of mFileLogLevel
Definition at line 389 of file PCMLogger.h.
void ParCompMark::Logger::setLogFileName | ( | const std::string & | logfilename | ) | [inline] |
Setter of mLogFileName.
Sets value of mLogFileName.
- Parameters:
-
[in] logfilename The value of mLogFileName
Definition at line 410 of file PCMLogger.h.
std::string ParCompMark::Logger::translateException | ( | const Exception & | exception | ) | [static] |
Translate an exception to human readable.
- Parameters:
-
[in] exception Exception.
- Returns:
Definition at line 90 of file PCMLogger.cpp.
References ParCompMark::Exception::getFileName(), ParCompMark::Network::getIfConfs(), ParCompMark::Exception::getType(), and ParCompMark::Exception::translateType().
Referenced by ParCompMark::Application::terminateHandler().
Here is the call graph for this function:
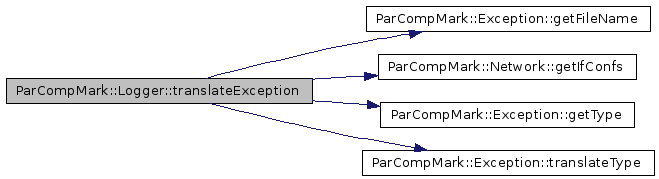
std::string ParCompMark::Logger::translateLogLevel | ( | const LogLevel & | loglevel | ) | [inline, static] |
Translate log level to human readable.
- Parameters:
-
[in] loglevel Logging level.
- Returns:
Definition at line 421 of file PCMLogger.h.
Member Data Documentation
const u8 ParCompMark::Logger::LOGTOCONSOLE = 1 [static] |
Indicates that the logger logs to console.
- Remarks:
- This is own attribute of this class.
Definition at line 110 of file PCMLogger.h.
Referenced by Logger().
const u8 ParCompMark::Logger::LOGTOFILE = 2 [static] |
Indicates that the logger logs to a textfile.
- Remarks:
- This is own attribute of this class.
Definition at line 117 of file PCMLogger.h.
Referenced by initialize(), and Logger().
LogLevel ParCompMark::Logger::mConsoleLogLevel [protected] |
Logging level for console (from FATAL to NOTICE).
- Remarks:
- This is own attribute of this class.
Definition at line 142 of file PCMLogger.h.
Referenced by Logger().
LogLevel ParCompMark::Logger::mFileLogLevel [protected] |
Logging level for file (from FATAL to NOTICE).
- Remarks:
- This is own attribute of this class.
Definition at line 149 of file PCMLogger.h.
Referenced by Logger().
Pointer< FILE, Mutex > ParCompMark::Logger::mFp [protected] |
File pointer.
- Remarks:
- This is own attribute of this class.
Definition at line 156 of file PCMLogger.h.
Referenced by initialize(), Logger(), and ~Logger().
bool ParCompMark::Logger::mInitialized [protected] |
Indicates that the logger is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 163 of file PCMLogger.h.
Referenced by initialize(), Logger(), and ~Logger().
std::string ParCompMark::Logger::mLogFileName [protected] |
Name of the log file.
- Remarks:
- This is own attribute of this class.
Definition at line 170 of file PCMLogger.h.
Referenced by initialize(), and Logger().
u8 ParCompMark::Logger::mLogMode [protected] |
Logging mode (console and/or textfile).
- Remarks:
- This is own attribute of this class.
Definition at line 135 of file PCMLogger.h.
Referenced by initialize(), and Logger().
The documentation for this class was generated from the following files: