ParCompMark::Network Class Reference
#include <PCMNetwork.h>
Inheritance diagram for ParCompMark::Network:
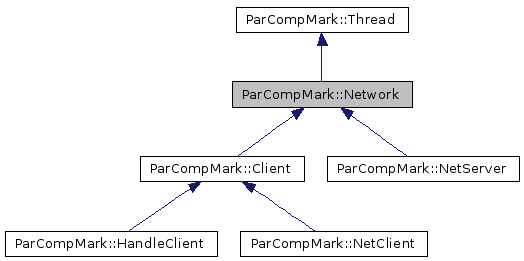
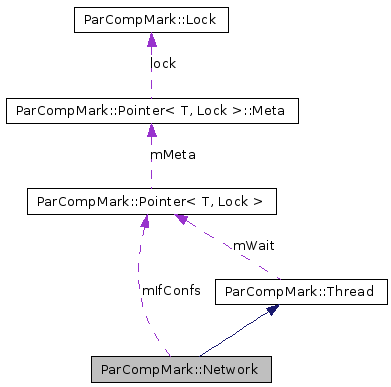
Detailed Description
Class for network routines.
Definition at line 70 of file PCMNetwork.h.
Getters & setters | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
const int & | getStreamSocket () const |
Getter of mStreamSocket. | |
void | setStreamSocket (const int &streamsocket) |
Setter of mStreamSocket. | |
const int & | getBroadcastSocket () const |
Getter of mBroadcastSocket. | |
const std::string & | getOwnIP () const |
Getter of mOwnIP. | |
static Container< Network::IfConf, DummyLock >::Pointer & | getIfConfs () |
Getter of mIfConfs. | |
static const unsigned short & | getCommunicationPort () |
Getter of mCommunicationPort. | |
static void | setCommunicationPort (const unsigned short &communicationport) |
Setter of mCommunicationPort. | |
static const unsigned short & | getBroadcastPort () |
Getter of mBroadcastPort. | |
static void | setBroadcastPort (const unsigned short &broadcastport) |
Setter of mBroadcastPort. | |
Public Types | |
typedef Pointer< Network, DummyLock > | Pointer |
Type for pointer to this class. | |
typedef ParCompMark::Network::IfConf | IfConf |
Struct for network interfaces IP information. | |
Public Member Functions | |
Constructors & destructor | |
Network (const std::string &name) | |
Create network class. | |
virtual | ~Network () |
The destructor. | |
Static Public Member Functions | |
Class methods | |
static void | getIP () |
Get local machine IP address-es. | |
static std::string | getHostName () |
Get local machine name. | |
Protected Attributes | |
Variables | |
bool | mInitialized |
Indicates initialized the class. | |
int | mStreamSocket |
The client socket number. | |
int | mBroadcastSocket |
The broadcast socket number. | |
std::string | mOwnIP |
IP address of the master node. | |
Static Protected Attributes | |
Class variables | |
static Container< Network::IfConf, DummyLock >::Pointer | mIfConfs |
Configurations of network interfaces of a host. | |
static unsigned short | mCommunicationPort = 1234 |
The communication port number. | |
static unsigned short | mBroadcastPort = 1235 |
The broadcast port number. | |
Classes | |
struct | IfConf |
Struct for network interfaces IP information. More... |
Member Typedef Documentation
typedef struct ParCompMark::Network::IfConf ParCompMark::Network::IfConf |
Struct for network interfaces IP information.
typedef Pointer< Network, DummyLock > ParCompMark::Network::Pointer |
Type for pointer to this class.
Reimplemented in ParCompMark::Client, ParCompMark::HandleClient, ParCompMark::NetClient, and ParCompMark::NetServer.
Definition at line 88 of file PCMNetwork.h.
Constructor & Destructor Documentation
ParCompMark::Network::Network | ( | const std::string & | name | ) |
Create network class.
- Parameters:
-
[in] name Name of the network.
Definition at line 46 of file PCMNetwork.cpp.
References ParCompMark::Singleton< T >::getInstance(), mBroadcastSocket, mInitialized, mOwnIP, mStreamSocket, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::Network::~Network | ( | ) | [virtual] |
Member Function Documentation
const unsigned short & ParCompMark::Network::getBroadcastPort | ( | ) | [inline, static] |
Getter of mBroadcastPort.
Returns value of mBroadcastPort.
- Returns:
- The value of mBroadcastPort
Definition at line 368 of file PCMNetwork.h.
const int & ParCompMark::Network::getBroadcastSocket | ( | ) | const [inline] |
Getter of mBroadcastSocket.
Returns value of mBroadcastSocket.
- Returns:
- The value of mBroadcastSocket
Definition at line 396 of file PCMNetwork.h.
const unsigned short & ParCompMark::Network::getCommunicationPort | ( | ) | [inline, static] |
Getter of mCommunicationPort.
Returns value of mCommunicationPort.
- Returns:
- The value of mCommunicationPort
Definition at line 354 of file PCMNetwork.h.
std::string ParCompMark::Network::getHostName | ( | ) | [static] |
Get local machine name.
- Returns:
- name of host
Definition at line 168 of file PCMNetwork.cpp.
Referenced by ParCompMark::Client::handleMessage(), ParCompMark::Application::setEnvironmentVariablesToSquirrel(), and ParCompMark::Application::soldierOperation().
Container< Network::IfConf, DummyLock >::Pointer & ParCompMark::Network::getIfConfs | ( | ) | [inline, static] |
Getter of mIfConfs.
Returns value of mIfConfs.
- Returns:
- The value of mIfConfs
Definition at line 340 of file PCMNetwork.h.
Referenced by ParCompMark::Logger::Logger(), and ParCompMark::Logger::translateException().
const bool & ParCompMark::Network::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 347 of file PCMNetwork.h.
void ParCompMark::Network::getIP | ( | ) | [static] |
Get local machine IP address-es.
Definition at line 81 of file PCMNetwork.cpp.
References ParCompMark::Pointer< T, Lock >::isNull(), ParCompMark::Pointer< T, Lock >::kill(), and mIfConfs.
Referenced by ParCompMark::Client::handleMessage(), ParCompMark::Logger::Logger(), ParCompMark::NetClient::NetClient(), and ParCompMark::NetServer::NetServer().
Here is the call graph for this function:

const std::string & ParCompMark::Network::getOwnIP | ( | ) | const [inline] |
Getter of mOwnIP.
Returns value of mOwnIP.
- Returns:
- The value of mOwnIP
Definition at line 403 of file PCMNetwork.h.
const int & ParCompMark::Network::getStreamSocket | ( | ) | const [inline] |
Getter of mStreamSocket.
Returns value of mStreamSocket.
- Returns:
- The value of mStreamSocket
Definition at line 382 of file PCMNetwork.h.
void ParCompMark::Network::setBroadcastPort | ( | const unsigned short & | broadcastport | ) | [inline, static] |
Setter of mBroadcastPort.
Sets value of mBroadcastPort.
- Parameters:
-
[in] broadcastport The value of mBroadcastPort
Definition at line 375 of file PCMNetwork.h.
Referenced by ParCompMark::Application::setFromConfigOptions().
void ParCompMark::Network::setCommunicationPort | ( | const unsigned short & | communicationport | ) | [inline, static] |
Setter of mCommunicationPort.
Sets value of mCommunicationPort.
- Parameters:
-
[in] communicationport The value of mCommunicationPort
Definition at line 361 of file PCMNetwork.h.
Referenced by ParCompMark::Application::setFromConfigOptions().
void ParCompMark::Network::setStreamSocket | ( | const int & | streamsocket | ) | [inline] |
Setter of mStreamSocket.
Sets value of mStreamSocket.
- Parameters:
-
[in] streamsocket The value of mStreamSocket
Definition at line 389 of file PCMNetwork.h.
Referenced by ParCompMark::NetServer::task().
Member Data Documentation
unsigned short ParCompMark::Network::mBroadcastPort = 1235 [static, protected] |
The broadcast port number.
- Remarks:
- This is own attribute of this class.
Definition at line 133 of file PCMNetwork.h.
Referenced by ParCompMark::NetServer::initialize(), and ParCompMark::NetClient::initialize().
int ParCompMark::Network::mBroadcastSocket [protected] |
The broadcast socket number.
- Remarks:
- This is own attribute of this class.
Definition at line 165 of file PCMNetwork.h.
Referenced by ParCompMark::NetServer::finalize(), ParCompMark::NetClient::finalize(), ParCompMark::NetServer::initialize(), ParCompMark::NetClient::initialize(), Network(), ParCompMark::NetServer::sendBroadcastMessage(), and ParCompMark::NetClient::task().
unsigned short ParCompMark::Network::mCommunicationPort = 1234 [static, protected] |
The communication port number.
- Remarks:
- This is own attribute of this class.
Definition at line 126 of file PCMNetwork.h.
Referenced by ParCompMark::NetServer::initialize(), and ParCompMark::Client::openConnection().
Container< Network::IfConf, DummyLock >::Pointer ParCompMark::Network::mIfConfs [static, protected] |
Configurations of network interfaces of a host.
- Remarks:
- This is own attribute of this class.
Definition at line 119 of file PCMNetwork.h.
Referenced by getIP(), ParCompMark::NetServer::initialize(), and ParCompMark::NetClient::NetClient().
bool ParCompMark::Network::mInitialized [protected] |
Indicates initialized the class.
- Remarks:
- This is own attribute of this class.
Definition at line 151 of file PCMNetwork.h.
Referenced by ParCompMark::NetServer::finalize(), ParCompMark::NetClient::finalize(), ParCompMark::HandleClient::finalize(), ParCompMark::HandleClient::HandleClient(), ParCompMark::NetServer::initialize(), ParCompMark::NetClient::initialize(), ParCompMark::HandleClient::initialize(), Network(), ParCompMark::HandleClient::~HandleClient(), ParCompMark::NetClient::~NetClient(), and ParCompMark::NetServer::~NetServer().
std::string ParCompMark::Network::mOwnIP [protected] |
IP address of the master node.
- Remarks:
- This is own attribute of this class.
Definition at line 172 of file PCMNetwork.h.
Referenced by ParCompMark::NetClient::NetClient(), Network(), and ParCompMark::NetServer::task().
int ParCompMark::Network::mStreamSocket [protected] |
The client socket number.
- Remarks:
- This is own attribute of this class.
Definition at line 158 of file PCMNetwork.h.
Referenced by ParCompMark::Client::closeConnection(), ParCompMark::NetServer::finalize(), ParCompMark::NetClient::finalize(), ParCompMark::HandleClient::finalize(), ParCompMark::Client::handleMessage(), ParCompMark::NetServer::initialize(), Network(), ParCompMark::HandleClient::openConnection(), ParCompMark::Client::openConnection(), ParCompMark::Client::recieveMessage(), ParCompMark::Client::sendMessage(), ParCompMark::NetServer::task(), and ParCompMark::HandleClient::task().
The documentation for this class was generated from the following files: