ParCompMark::Application Class Reference
#include <PCMApplication.h>
Inheritance diagram for ParCompMark::Application:
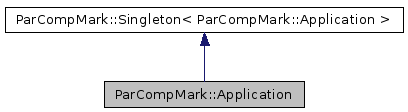
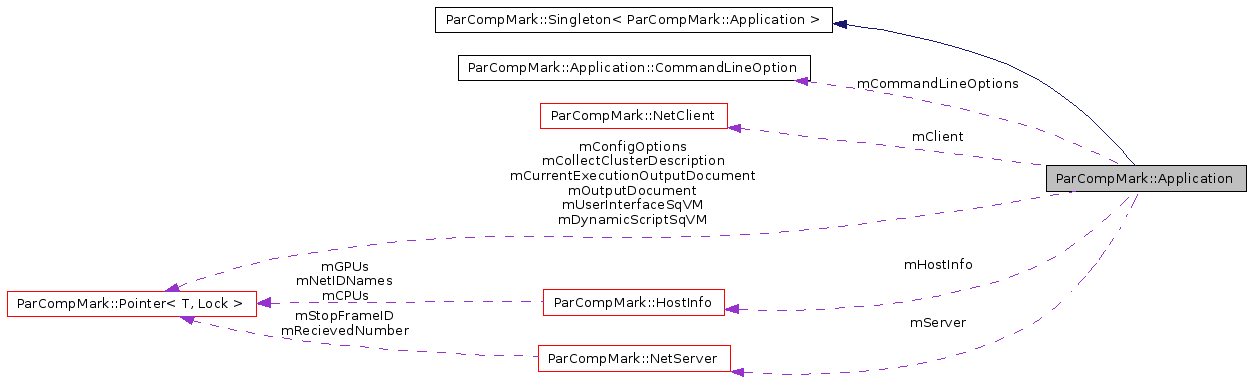
Detailed Description
This singleton class handles the application initializing, command line parsing, starting tasks etc.
Definition at line 87 of file PCMApplication.h.
Getters & setters | |
HostInfo * | getHostInfo () const |
Getter of mHostInfo. | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
const Application::PostCommand & | getPostCommand () const |
Getter of mPostCommand. | |
const bool & | getQuiting () const |
Getter of mQuiting. | |
const bool & | getOutputIsDone () const |
Getter of mOutputIsDone. | |
OutputNode::Pointer & | getOutputDocument () |
Getter of mOutputDocument. | |
OutputNode::Pointer & | getCurrentExecutionOutputDocument () |
Getter of mCurrentExecutionOutputDocument. | |
u32 & | getExecutionIndex () |
Getter of mExecutionIndex. | |
OutputNode::Pointer & | getCollectClusterDescription () |
Getter of mCollectClusterDescription. | |
ConfigOptions::Pointer & | getConfigOptions () |
Getter of mConfigOptions. | |
const std::string & | getLowLevelScript () const |
Getter of mLowLevelScript. | |
const std::string & | getDynamicScript () const |
Getter of mDynamicScript. | |
const std::string & | getScenarioScript () const |
Getter of mScenarioScript. | |
const std::list< std::string > & | getSquirrelCommandList () const |
Getter of mSquirrelCommandList. | |
bool & | getDynamicScriptCompiled () |
Getter of mDynamicScriptCompiled. | |
void | setDynamicScriptCompiled (const bool dynamicscriptcompiled) |
Setter of mDynamicScriptCompiled. | |
NetServer * | getServer () const |
Getter of mServer. | |
NetClient * | getClient () const |
Getter of mClient. | |
bool & | getCompRun () |
Getter of mCompRun. | |
static const std::string & | getLibrarySearchPath () |
Getter of mLibrarySearchPath. | |
static void | setLibrarySearchPath (const std::string &librarysearchpath) |
Setter of mLibrarySearchPath. | |
static const std::string & | getUsageString () |
Getter of mUsageString. | |
static const bool & | getCommanderMode () |
Getter of mCommanderMode. | |
static const bool & | getGUIMode () |
Getter of mGUIMode. | |
static const bool & | getManualClusterDescription () |
Getter of mManualClusterDescription. | |
static const std::string & | getClusterDescription () |
Getter of mClusterDescription. | |
static const bool & | getLowLevelMode () |
Getter of mLowLevelMode. | |
static const bool & | getInteractiveParameters () |
Getter of mInteractiveParameters. | |
static const std::string & | getParameters () |
Getter of mParameters. | |
static const std::string & | getInput () |
Getter of mInput. | |
static const std::string & | getOutput () |
Getter of mOutput. | |
static int & | getExpectedHostCount () |
Getter of mExpectedHostCount. | |
static const Application::UserInterface & | getUserInterface () |
Getter of mUserInterface. | |
Methods | |
virtual void | setupHandlers () const |
Setup special event handlers. | |
virtual void | initialize () |
Initialize the PCM application. | |
virtual void | finalize () |
Finalize the PCM application. | |
virtual void | finalizeCommander () |
Addintional finalization code for commander mode. | |
virtual void | finalizeSoldier () |
Addintional finalization code for soldier mode. | |
virtual void | setFromConfigOptions () |
Call application-wide setters on config options. | |
virtual void | createVirtualMachines () |
Create and initialize virtual machines for dynamic script execution and for user interaction handling. | |
virtual bool | startOperation () |
The application starts its operation. | |
virtual void | writeOutput () |
Write collected output. | |
virtual void | waitForOutput () |
Wait until the output is fully written. | |
virtual void | quit () |
Quit from application. | |
virtual void | compileDynamic () |
Compile dynamic script. | |
virtual void | start () |
Start compositing. | |
virtual void | stop () |
Stop compositing. | |
virtual void | autoDetection () |
Auto detection of the cluster. | |
virtual void | cleanup () |
Do cleanup. | |
virtual char * | getHostListForSquirrel () |
Return the list of hosts in cluster as a Squirrel string array. | |
virtual char * | getLowLevelScriptForSquirrel () |
Return the low-level script as a string. | |
virtual char * | getDynamicScriptForSquirrel () |
Return the dynamic script as a string. | |
virtual bool | hasScenarioScript () |
Return true if a scenario script is loaded. | |
virtual char * | getDynamicScriptParametersForSquirrel () |
Return the list of dynamic script parameters as a Squirrel string array. | |
virtual char * | getProgramInfo () |
Return one line program info as a string. | |
virtual void | calculateMessageSendTime () |
Calcualtes the message sendign time. | |
virtual void | _pseudoStop () |
Handle pseudoSTOP post command. | |
virtual bool | commanderOperation () |
Operation of a commander mode application. | |
virtual bool | soldierOperation () |
Operation of a soldier mode (not commander mode) application. | |
virtual bool | textUserInterface () |
Start textual user interface. | |
virtual bool | noUserInterface () |
Start "no" user interface. | |
virtual bool | executeUserCommand (const std::string &command) |
Executes a single line user command. | |
virtual bool | processSquirrelCommandList () |
Executes the commands in the squirrel command list. | |
virtual void | doPostCommand () |
Executes current post command task. | |
virtual void | loadLowLevelScript (const char *filename) |
Load low-level script from the specified filename. | |
virtual void | loadDynamicScript (const char *filename) |
Load dynamic script from the specified filename. | |
virtual void | loadScenarioScript (const char *filename) |
Load scenario script from the specified filename. | |
virtual void | pushSquirrelCommand (const char *command) |
Push a command into the Squirrel command list. | |
virtual void | createLowLevelScript (const std::string &clusterDescription) |
Create low-level script from dynamic script and cluster description. | |
virtual void | processScenarioScript () |
Process scenario script file. | |
virtual void | setEnvironmentVariablesToSquirrel () |
Set environment variables to the Squirrel. | |
virtual void | initializeDynamicScriptParameters (const bool &reset) |
Compile dynamic script and initialize dynamic script parameters in reset or complement mode. | |
virtual void | retrieveDynamicScriptParameters () |
Retrieve dynamic script parameters from the loaded dynamic script. | |
virtual void | _loadScenario () |
Handle LOADSCENARIO post command. | |
virtual void | _loadDynamic () |
Handle LOADDYNAMIC post command. | |
virtual void | _compileDynamic () |
Handle COMPILEDYNAMIC post command. | |
virtual void | _start () |
Handle START post command. | |
virtual void | _stop () |
Handle STOP post command. | |
virtual void | _quit () |
Handle QUIT post command. | |
Class constants | |
static const std::string | PCAPINUT = "scripts/framework/pcapi.nut" |
PC API Squirrel script file (relative to the data directory). | |
static const std::string | PARCOMPMARKNUT = "scripts/framework/parcompmark.nut" |
ParCompMark API Squirrel script file (relative to the data directory). | |
static const std::string | UTILSNUT = "scripts/framework/utils.nut" |
Common utilities Squirrel script file (relative to the data directory). | |
static const std::string | ABBREVIATIONSNUT = "scripts/framework/abbreviations.nut" |
User command abbreviations resolver Squirrel script file (relative to the data directory). | |
static const std::string | DYNAMICINITNUT = "scripts/framework/dynamic-init.nut" |
Dynamic script initialization Squirrel script file (relative to the data directory). | |
static const std::string | UIINITNUT = "scripts/framework/ui-init.nut" |
User interface initialization Squirrel script file (relative to the data directory). | |
static const std::string | COMMANDDIRECTORY = "scripts/commands/" |
Directory that contatins user commands in separated nut files (relative to the data directory). | |
static const std::string | HELPNUT = "scripts/commands/help.nut" |
Path of help.nut file in user commands directory (relative to the data directory). | |
static const std::string | PCIMPLEMENTATIONS [] |
List of supported PC implementation libraries. | |
static const Application::CommandLineOption | mCommandLineOptions [] |
Command line options for ParCompMark. | |
static const u32 | mCommandLineOptionCount = sizeof(mCommandLineOptions) / sizeof(mCommandLineOptions[0]) |
Number of command line options. | |
static const std::string | mUsageString = "ParCompMark [ options ]" |
Application usage string. | |
Public Member Functions | |
Constructors & destructor | |
Application () | |
Default constructor. | |
virtual | ~Application () |
The destructor. | |
Static Public Member Functions | |
Scripting binding | |
static void | squirrelGlue () |
Static glue code method for Squirrel-C++ binding. | |
Class methods | |
static int | complement (int first, int second) |
Complement command line commands. | |
static void | parseCommandLine (const u32 &argc, const char **&argv) |
Parse ANSI C command line. | |
static void | showHelp (const std::string &strarg) |
Write help to std out. | |
static void | showVersion (const std::string &strarg) |
Write version to std out. | |
static void | setCommanderOn (const std::string &strarg) |
Set commander mode. | |
static void | setGUIOn (const std::string &strarg) |
Set GUI mode. | |
static void | setLowLevelOn (const std::string &strarg) |
Set low-level scripting mode. | |
static void | setLowLevelScriptFile (const std::string &strarg) |
Set low-level script file. | |
static void | setDynamicScriptFile (const std::string &strarg) |
Set dynamic script file. | |
static void | setScenarioScriptFile (const std::string &strarg) |
Set scenario script file. | |
static void | setCluster (const std::string &strarg) |
Set cluster description file. | |
static void | setParameters (const std::string &strarg) |
Set parameters description file. | |
static void | setInput (const std::string &strarg) |
Set input file. | |
static void | setOutput (const std::string &strarg) |
Set output file. | |
static void | setExpectedHostCount (const std::string &hostCount) |
Set expected number of rendering hosts. | |
static void | setUserInterface (const std::string &strUI) |
Set user the type of interface. | |
static bool | hasEnvironmentVariable (const std::string &name) |
Returns true if specified environment variable exists. | |
static std::string | getEnvironmentVariable (const std::string &name) |
Get environment variable. | |
static void | terminateHandler () |
Abnormal termination handler. | |
static void | terminateHandlerNOP () |
No operation termination handler. | |
static void | unexpectedHandler () |
Unexpected exception handler. | |
static void | segfaultHandler (int value) |
Segmentation fault handler. | |
static void | interruptHandler (int value) |
Interrupt signal handler. | |
static Application * | getInstance () |
Gets the instance of the singleton class. | |
Protected Types | |
NOTHING | |
Nothing to do. | |
LOADSCENARIO | |
Load scenario script. | |
LOADDYNAMIC | |
Load dynamic script. | |
COMPILE | |
Compile dynamic script. | |
START | |
Start compositing. | |
COMPILE_START | |
Compile dynamic script and start compositing. | |
STOP | |
Stop compositing. | |
QUIT | |
Quit from application. | |
STOP_QUIT | |
Stop compositing and quit from application. | |
STRING = 0 | |
String type. | |
INTEGER = 1 | |
Integer type. | |
REAL = 2 | |
Floating point type. | |
BOOL = 3 | |
Boolean type. | |
NONE | |
Do not provide user interface. | |
CONSOLE | |
Textual user interface using GNU readline() or gets(). | |
enum | PostCommand { NOTHING, LOADSCENARIO, LOADDYNAMIC, COMPILE, START, COMPILE_START, STOP, QUIT, STOP_QUIT } |
Definitions of for post command tasks. More... | |
enum | DynamicScriptParameterType { STRING = 0, INTEGER = 1, REAL = 2, BOOL = 3 } |
Definitions of for post command tasks. More... | |
enum | UserInterface { NONE, CONSOLE } |
Definitions of for user interface types. More... | |
Protected Attributes | |
Variables | |
HostInfo * | mHostInfo |
Information about the host. | |
bool | mInitialized |
The application is initialized. | |
PostCommand | mPostCommand |
Current post command task. | |
bool | mQuiting |
Flag indicating that the application is quitting now (to avoid multiple quit requests in quit() method). | |
bool | mOutputIsDone |
Flag indicating that the output document has been written now and it is safe to quit. | |
OutputNode::Pointer | mOutputDocument |
Root of the output document. | |
OutputNode::Pointer | mCurrentExecutionOutputDocument |
The output document has separate 'execution' children for each benchmark execution. | |
u32 | mExecutionIndex |
Index of the actual execution. | |
OutputNode::Pointer | mCollectClusterDescription |
Root of the output document. | |
ConfigOptions::Pointer | mConfigOptions |
Program configuration options. | |
std::string | mLowLevelScript |
Squirrel low-level script. | |
std::string | mDynamicScript |
Squirrel cluster dynamic script. | |
std::string | mScenarioScript |
Squirrel scenario script. | |
std::list< std::string > | mSquirrelCommandList |
List of Squirrel commands to execute. | |
bool | mDynamicScriptCompiled |
Indicates that the dynamic script is compiled. | |
NetServer * | mServer |
Server network. | |
NetClient * | mClient |
Client network. | |
bool | mCompRun |
Output file name. | |
SqVM::Pointer | mDynamicScriptSqVM |
Squirrel virtual machine for dynamic script execution. | |
SqVM::Pointer | mUserInterfaceSqVM |
Squirrel virtual machine to handle user interaction. | |
std::list< DynamicScriptParameter * > | mDynamicScriptParameters |
List of dynamic script parameters. | |
Static Protected Attributes | |
Class variables | |
static std::string | mLibrarySearchPath = "/usr/lib" |
PC dynamic library search path (default /usr/lib). | |
static bool | mCommanderMode = false |
Indicates commander mode (default false). | |
static bool | mGUIMode = false |
Indicates GUI mode (default false). | |
static bool | mManualClusterDescription = false |
Indicates manual cluster description (default false). | |
static std::string | mClusterDescription |
Cluster description file name. | |
static bool | mLowLevelMode = false |
Indicates low-level scripting mode (default false). | |
static bool | mInteractiveParameters = true |
Indicates interactive parameter settings (default true). | |
static std::string | mParameters |
Parameters description file name. | |
static std::string | mInput = "-" |
Input script file name. | |
static std::string | mOutput = "-" |
Output file name. | |
static int | mExpectedHostCount = 0 |
Expected number of rendering hosts. | |
static UserInterface | mUserInterface = CONSOLE |
Type of user interface. | |
Classes | |
struct | CommandLineOption |
Structure for command line options. More... | |
struct | DynamicScriptParameter |
Structure for dynamic script parameters. More... |
Member Enumeration Documentation
enum ParCompMark::Application::DynamicScriptParameterType [protected] |
Definitions of for post command tasks.
Post command task is a job to execute after stopping the user interface Squirrel VM.
Definition at line 182 of file PCMApplication.h.
enum ParCompMark::Application::PostCommand [protected] |
Definitions of for post command tasks.
Post command task is a job to execute after stopping the user interface Squirrel VM.
- Enumerator:
Definition at line 150 of file PCMApplication.h.
enum ParCompMark::Application::UserInterface [protected] |
Definitions of for user interface types.
- Enumerator:
-
NONE Do not provide user interface. This is used for batch jobs
CONSOLE Textual user interface using GNU readline() or gets().
Definition at line 199 of file PCMApplication.h.
Constructor & Destructor Documentation
ParCompMark::Application::Application | ( | ) |
Default constructor.
Definition at line 116 of file PCMApplication.cpp.
References ParCompMark::Singleton< T >::getInstance(), initialize(), mClient, mCompRun, mInitialized, mOutputIsDone, mPostCommand, mQuiting, mServer, NOTHING, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::Application::~Application | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 169 of file PCMApplication.cpp.
References finalize(), and mInitialized.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::Application::_compileDynamic | ( | ) | [protected, virtual] |
Handle COMPILEDYNAMIC post command.
Definition at line 1529 of file PCMApplication.cpp.
References createLowLevelScript(), ParCompMark::Singleton< T >::getInstance(), and mDynamicScript.
Referenced by doPostCommand().
Here is the call graph for this function:

void ParCompMark::Application::_loadDynamic | ( | ) | [protected, virtual] |
Handle LOADDYNAMIC post command.
Definition at line 1518 of file PCMApplication.cpp.
References initializeDynamicScriptParameters(), and setEnvironmentVariablesToSquirrel().
Referenced by doPostCommand().
Here is the call graph for this function:

void ParCompMark::Application::_loadScenario | ( | ) | [protected, virtual] |
Handle LOADSCENARIO post command.
Definition at line 1511 of file PCMApplication.cpp.
References processScenarioScript().
Referenced by doPostCommand().
Here is the call graph for this function:

void ParCompMark::Application::_pseudoStop | ( | ) | [virtual] |
Handle pseudoSTOP post command.
Definition at line 970 of file PCMApplication.cpp.
References Assert, mCompRun, mServer, and ParCompMark::NetServer::sendBroadcastMessage().
Referenced by ParCompMark::Process::threadFinalize().
Here is the call graph for this function:

void ParCompMark::Application::_quit | ( | ) | [protected, virtual] |
Handle QUIT post command.
Definition at line 1621 of file PCMApplication.cpp.
References _stop(), mCompRun, mQuiting, mServer, ParCompMark::NetServer::sendBroadcastMessage(), and writeOutput().
Referenced by doPostCommand(), interruptHandler(), showHelp(), and showVersion().
Here is the call graph for this function:

void ParCompMark::Application::_start | ( | ) | [protected, virtual] |
Handle START post command.
Definition at line 1544 of file PCMApplication.cpp.
References Assert, ParCompMark::StringConverter::DEFAULTFIELDWIDTH, Except, ParCompMark::Pointer< T, Lock >::getLocked(), ParCompMark::Exception::getType(), ParCompMark::Timer::getUncorrectedSystemTime(), ParCompMark::OutputNode::INFORMATION, ParCompMark::Pointer< T, Lock >::lock(), mCollectClusterDescription, mCompRun, mCurrentExecutionOutputDocument, mDynamicScriptSqVM, mExecutionIndex, mLowLevelScript, mOutputDocument, mOutputIsDone, mServer, ParCompMark::Exception::SCRIPT_ERROR, ParCompMark::NetServer::sendBroadcastMessage(), ParCompMark::StringConverter::toString(), and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by doPostCommand().
Here is the call graph for this function:
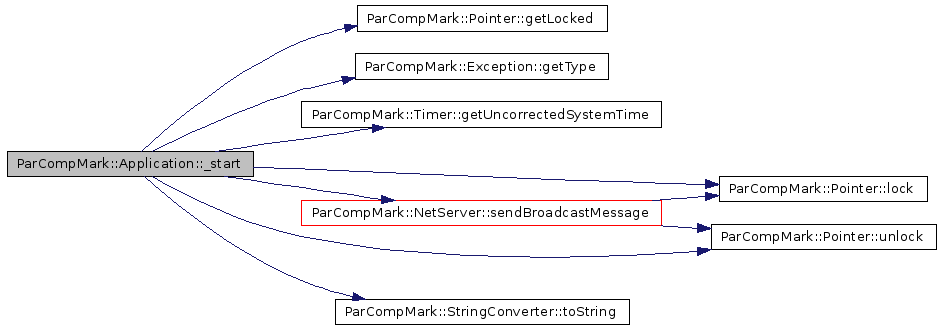
void ParCompMark::Application::_stop | ( | ) | [protected, virtual] |
Handle STOP post command.
Definition at line 1600 of file PCMApplication.cpp.
References Assert, ParCompMark::Pointer< T, Lock >::getPtr(), ParCompMark::NetServer::getStopFrameID(), ParCompMark::Pointer< T, Lock >::lock(), mCompRun, mServer, ParCompMark::NetServer::sendBroadcastMessage(), ParCompMark::StringConverter::toString(), and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by _quit(), and doPostCommand().
Here is the call graph for this function:
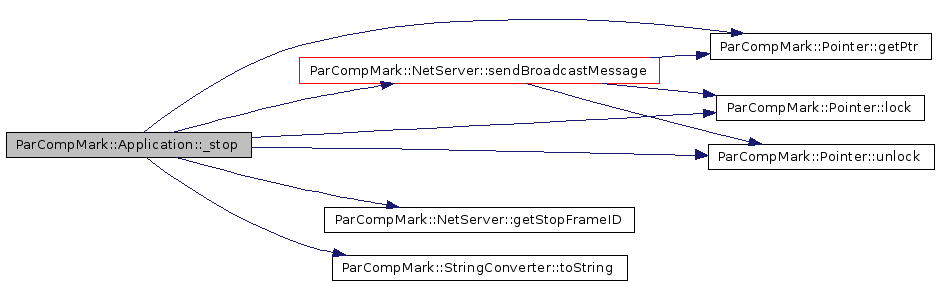
void ParCompMark::Application::autoDetection | ( | ) | [virtual] |
Auto detection of the cluster.
Definition at line 867 of file PCMApplication.cpp.
References Assert, ParCompMark::NetServer::buildCluster(), mCompRun, mDynamicScriptCompiled, and mServer.
Referenced by squirrelGlue().
Here is the call graph for this function:

void ParCompMark::Application::calculateMessageSendTime | ( | ) | [virtual] |
Calcualtes the message sendign time.
Definition at line 963 of file PCMApplication.cpp.
References mServer, and ParCompMark::NetServer::sendBroadcastMessage().
Here is the call graph for this function:

void ParCompMark::Application::cleanup | ( | ) | [virtual] |
Do cleanup.
Empty output document etc.
Definition at line 889 of file PCMApplication.cpp.
References mCurrentExecutionOutputDocument, and mOutputDocument.
Referenced by squirrelGlue().
bool ParCompMark::Application::commanderOperation | ( | ) | [protected, virtual] |
Operation of a commander mode application.
- Returns:
- Return true on error.
Definition at line 985 of file PCMApplication.cpp.
References Assert, ParCompMark::Singleton< T >::createInstance(), createVirtualMachines(), doPostCommand(), ParCompMark::Logger::FATAL, ParCompMark::FileSystemManager::getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::SqVM::getScriptOutput(), loadDynamicScript(), loadLowLevelScript(), loadScenarioScript(), ParCompMark::Pointer< T, Lock >::lock(), mCommanderMode, mConfigOptions, mServer, mUserInterfaceSqVM, ParCompMark::SqVM::NOMAINMETHOD, ParCompMark::Logger::NOTICE, ParCompMark::SqVM::setScriptOutput(), ParCompMark::Thread::startThread(), ParCompMark::SqVM::STD, UIINITNUT, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by startOperation().
Here is the call graph for this function:
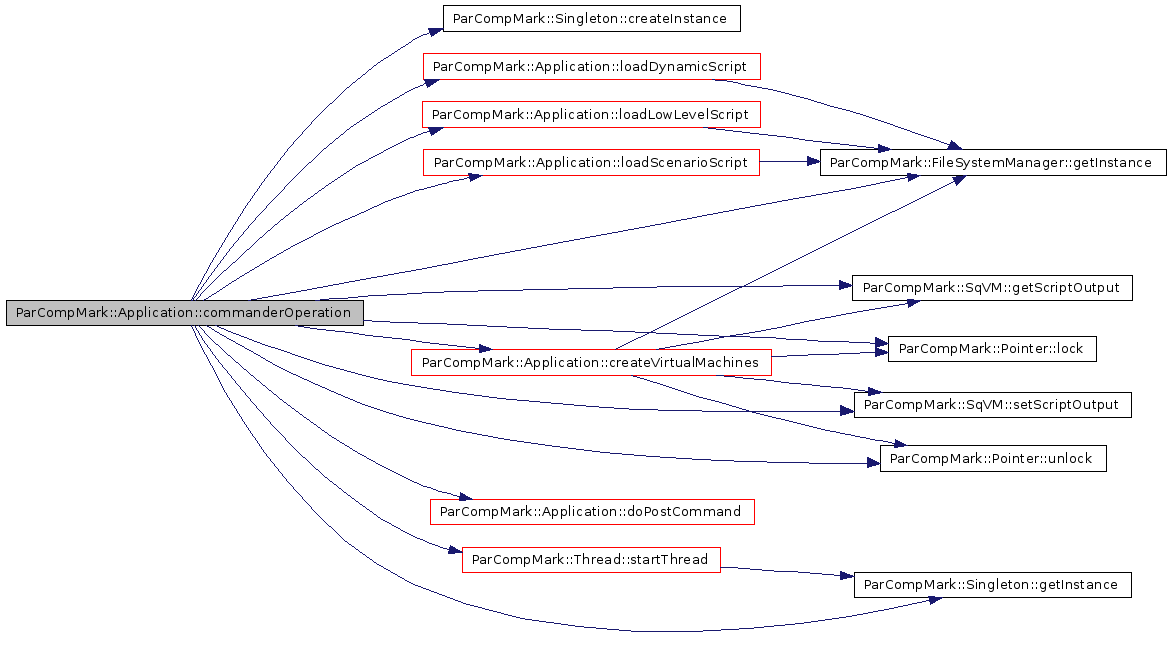
void ParCompMark::Application::compileDynamic | ( | ) | [virtual] |
Compile dynamic script.
Definition at line 846 of file PCMApplication.cpp.
References COMPILE, COMPILE_START, mPostCommand, and START.
Referenced by squirrelGlue().
int ParCompMark::Application::complement | ( | int | first, | |
int | second | |||
) | [static] |
Complement command line commands.
- Parameters:
-
[in] first Need for correct readline function type. [in] second Need for correct readline function type.
- Returns:
- Need for correct readline function type.
Definition at line 183 of file PCMApplication.cpp.
Referenced by initialize().
void ParCompMark::Application::createLowLevelScript | ( | const std::string & | clusterDescription | ) | [protected, virtual] |
Create low-level script from dynamic script and cluster description.
- Parameters:
-
[in] clusterDescription Squirrel cluster description.
Definition at line 1291 of file PCMApplication.cpp.
References ParCompMark::Pointer< T, Lock >::getLocked(), ParCompMark::Exception::getType(), ParCompMark::Pointer< T, Lock >::lock(), mDynamicScript, mDynamicScriptCompiled, mDynamicScriptSqVM, mLowLevelScript, ParCompMark::Exception::SCRIPT_ERROR, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by _compileDynamic().
Here is the call graph for this function:

void ParCompMark::Application::createVirtualMachines | ( | ) | [virtual] |
Create and initialize virtual machines for dynamic script execution and for user interaction handling.
Definition at line 684 of file PCMApplication.cpp.
References ABBREVIATIONSNUT, COMMANDDIRECTORY, DYNAMICINITNUT, ParCompMark::FileSystemManager::getInstance(), ParCompMark::SqVM::getScriptOutput(), HELPNUT, ParCompMark::FileSystemManager::listDataDirectory(), ParCompMark::Pointer< T, Lock >::lock(), mDynamicScriptSqVM, mUserInterfaceSqVM, ParCompMark::SqVM::NOMAINMETHOD, PARCOMPMARKNUT, PCAPINUT, ParCompMark::SqVM::setScriptOutput(), ParCompMark::SqVM::STD, ParCompMark::Pointer< T, Lock >::unlock(), and UTILSNUT.
Referenced by commanderOperation().
Here is the call graph for this function:

void ParCompMark::Application::doPostCommand | ( | ) | [protected, virtual] |
Executes current post command task.
Definition at line 1216 of file PCMApplication.cpp.
References _compileDynamic(), _loadDynamic(), _loadScenario(), _quit(), _start(), _stop(), COMPILE, COMPILE_START, LOADDYNAMIC, LOADSCENARIO, mPostCommand, NOTHING, QUIT, START, STOP, and STOP_QUIT.
Referenced by commanderOperation(), noUserInterface(), processSquirrelCommandList(), and textUserInterface().
Here is the call graph for this function:
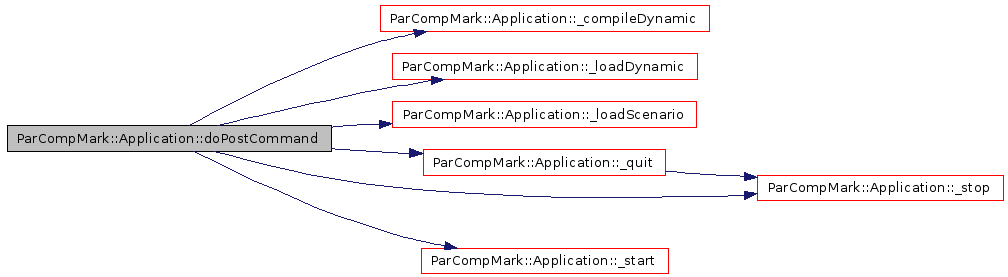
bool ParCompMark::Application::executeUserCommand | ( | const std::string & | command | ) | [protected, virtual] |
Executes a single line user command.
User command can be a Squirrel code fragment or an abrevation.
- Parameters:
-
[in] command User command.
- Returns:
- Return true on error.
Definition at line 1154 of file PCMApplication.cpp.
References ParCompMark::Pointer< T, Lock >::getLocked(), ParCompMark::SqVM::getScriptOutput(), ParCompMark::Exception::getType(), ParCompMark::Pointer< T, Lock >::lock(), mUserInterfaceSqVM, ParCompMark::SqVM::NOMAINMETHOD, ParCompMark::Exception::SCRIPT_ERROR, ParCompMark::SqVM::setScriptOutput(), ParCompMark::SqVM::STD, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by noUserInterface(), processSquirrelCommandList(), and textUserInterface().
Here is the call graph for this function:

void ParCompMark::Application::finalize | ( | ) | [virtual] |
Finalize the PCM application.
Definition at line 592 of file PCMApplication.cpp.
References Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::destroyInstance(), Except, finalizeCommander(), finalizeSoldier(), ParCompMark::FileSystemManager::getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::isNotNull(), ParCompMark::Pointer< T, Lock >::kill(), mCommanderMode, mConfigOptions, mDynamicScriptSqVM, mInitialized, mOutputDocument, and mUserInterfaceSqVM.
Referenced by terminateHandler(), and ~Application().
Here is the call graph for this function:

void ParCompMark::Application::finalizeCommander | ( | ) | [virtual] |
Addintional finalization code for commander mode.
This method is executed at the beginning of the common finalize() method.
Definition at line 635 of file PCMApplication.cpp.
References ParCompMark::Singleton< T >::destroyInstance(), and mServer.
Referenced by finalize().
Here is the call graph for this function:

void ParCompMark::Application::finalizeSoldier | ( | ) | [virtual] |
Addintional finalization code for soldier mode.
This method is executed at the beginning of the common finalize() method.
Definition at line 643 of file PCMApplication.cpp.
References ParCompMark::Host::closeXDisplays(), ParCompMark::Singleton< T >::destroyInstance(), ParCompMark::Host::getInstance(), and mClient.
Referenced by finalize().
Here is the call graph for this function:

NetClient * ParCompMark::Application::getClient | ( | ) | const [inline] |
Getter of mClient.
Returns value of mClient.
- Returns:
- The value of mClient
Definition at line 1451 of file PCMApplication.h.
const std::string & ParCompMark::Application::getClusterDescription | ( | ) | [inline, static] |
Getter of mClusterDescription.
Returns value of mClusterDescription.
- Returns:
- The value of mClusterDescription
Definition at line 1486 of file PCMApplication.h.
OutputNode::Pointer & ParCompMark::Application::getCollectClusterDescription | ( | ) | [inline] |
Getter of mCollectClusterDescription.
Returns value of mCollectClusterDescription.
- Returns:
- The value of mCollectClusterDescription
Definition at line 1388 of file PCMApplication.h.
const bool & ParCompMark::Application::getCommanderMode | ( | ) | [inline, static] |
Getter of mCommanderMode.
Returns value of mCommanderMode.
- Returns:
- The value of mCommanderMode
Definition at line 1465 of file PCMApplication.h.
bool & ParCompMark::Application::getCompRun | ( | ) | [inline] |
Getter of mCompRun.
Returns value of mCompRun.
- Returns:
- The value of mCompRun
Definition at line 1542 of file PCMApplication.h.
Referenced by squirrelGlue().
ConfigOptions::Pointer & ParCompMark::Application::getConfigOptions | ( | ) | [inline] |
Getter of mConfigOptions.
Returns value of mConfigOptions.
- Returns:
- The value of mConfigOptions
Definition at line 1395 of file PCMApplication.h.
Referenced by setDynamicScriptFile(), setLowLevelScriptFile(), and setScenarioScriptFile().
OutputNode::Pointer & ParCompMark::Application::getCurrentExecutionOutputDocument | ( | ) | [inline] |
Getter of mCurrentExecutionOutputDocument.
Returns value of mCurrentExecutionOutputDocument.
- Returns:
- The value of mCurrentExecutionOutputDocument
Definition at line 1374 of file PCMApplication.h.
const std::string & ParCompMark::Application::getDynamicScript | ( | ) | const [inline] |
Getter of mDynamicScript.
Returns value of mDynamicScript.
- Returns:
- The value of mDynamicScript
Definition at line 1409 of file PCMApplication.h.
bool & ParCompMark::Application::getDynamicScriptCompiled | ( | ) | [inline] |
Getter of mDynamicScriptCompiled.
Returns value of mDynamicScriptCompiled.
- Returns:
- The value of mDynamicScriptCompiled
Definition at line 1430 of file PCMApplication.h.
Referenced by squirrelGlue().
char * ParCompMark::Application::getDynamicScriptForSquirrel | ( | ) | [virtual] |
Return the dynamic script as a string.
- Returns:
- Dynamic script.
Definition at line 923 of file PCMApplication.cpp.
References mDynamicScript.
Referenced by squirrelGlue().
char * ParCompMark::Application::getDynamicScriptParametersForSquirrel | ( | ) | [virtual] |
Return the list of dynamic script parameters as a Squirrel string array.
- Returns:
- Dynamic parameter list.
Definition at line 938 of file PCMApplication.cpp.
References mDynamicScriptParameters.
Referenced by squirrelGlue().
std::string ParCompMark::Application::getEnvironmentVariable | ( | const std::string & | name | ) | [static] |
Get environment variable.
- Parameters:
-
[in] name Name of the variable.
- Returns:
- Value of the specified variable.
Definition at line 389 of file PCMApplication.cpp.
References Assert.
Referenced by ParCompMark::FileSystemManager::_findHomeDirectory(), ParCompMark::FileSystemManager::initialize(), and initialize().
u32 & ParCompMark::Application::getExecutionIndex | ( | ) | [inline] |
Getter of mExecutionIndex.
Returns value of mExecutionIndex.
- Returns:
- The value of mExecutionIndex
Definition at line 1381 of file PCMApplication.h.
int & ParCompMark::Application::getExpectedHostCount | ( | ) | [inline, static] |
Getter of mExpectedHostCount.
Returns value of mExpectedHostCount.
- Returns:
- The value of mExpectedHostCount
Definition at line 1528 of file PCMApplication.h.
Referenced by squirrelGlue().
const bool & ParCompMark::Application::getGUIMode | ( | ) | [inline, static] |
Getter of mGUIMode.
Returns value of mGUIMode.
- Returns:
- The value of mGUIMode
Definition at line 1472 of file PCMApplication.h.
HostInfo * ParCompMark::Application::getHostInfo | ( | ) | const [inline] |
Getter of mHostInfo.
Returns value of mHostInfo.
- Returns:
- The value of mHostInfo
Definition at line 1332 of file PCMApplication.h.
char * ParCompMark::Application::getHostListForSquirrel | ( | ) | [virtual] |
Return the list of hosts in cluster as a Squirrel string array.
- Returns:
- Host list.
Definition at line 897 of file PCMApplication.cpp.
References ParCompMark::Singleton< T >::getInstance().
Referenced by squirrelGlue().
Here is the call graph for this function:

const bool & ParCompMark::Application::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 1339 of file PCMApplication.h.
const std::string & ParCompMark::Application::getInput | ( | ) | [inline, static] |
Getter of mInput.
Returns value of mInput.
- Returns:
- The value of mInput
Definition at line 1514 of file PCMApplication.h.
Application * ParCompMark::Application::getInstance | ( | ) | [static] |
Gets the instance of the singleton class.
Reimplemented for squirrel usage.
- Returns:
- Instance of the class
Reimplemented from ParCompMark::Singleton< T >.
Definition at line 460 of file PCMApplication.cpp.
Referenced by ParCompMark::FileSystemManager::_findHomeDirectory(), ParCompMark::FileSystemManager::findLibraryPath(), ParCompMark::Client::handleMessage(), ParCompMark::FileSystemManager::initialize(), initialize(), interruptHandler(), setDynamicScriptFile(), setLowLevelScriptFile(), setScenarioScriptFile(), showHelp(), showVersion(), squirrelGlue(), terminateHandler(), ParCompMark::Process::threadFinalize(), and ParCompMark::Process::threadInitialize().
const bool & ParCompMark::Application::getInteractiveParameters | ( | ) | [inline, static] |
Getter of mInteractiveParameters.
Returns value of mInteractiveParameters.
- Returns:
- The value of mInteractiveParameters
Definition at line 1500 of file PCMApplication.h.
const std::string & ParCompMark::Application::getLibrarySearchPath | ( | ) | [inline, static] |
Getter of mLibrarySearchPath.
Returns value of mLibrarySearchPath.
- Returns:
- The value of mLibrarySearchPath
Definition at line 1318 of file PCMApplication.h.
const bool & ParCompMark::Application::getLowLevelMode | ( | ) | [inline, static] |
Getter of mLowLevelMode.
Returns value of mLowLevelMode.
- Returns:
- The value of mLowLevelMode
Definition at line 1493 of file PCMApplication.h.
const std::string & ParCompMark::Application::getLowLevelScript | ( | ) | const [inline] |
Getter of mLowLevelScript.
Returns value of mLowLevelScript.
- Returns:
- The value of mLowLevelScript
Definition at line 1402 of file PCMApplication.h.
char * ParCompMark::Application::getLowLevelScriptForSquirrel | ( | ) | [virtual] |
Return the low-level script as a string.
- Returns:
- Low-level script.
Definition at line 915 of file PCMApplication.cpp.
References mLowLevelScript.
Referenced by squirrelGlue().
const bool & ParCompMark::Application::getManualClusterDescription | ( | ) | [inline, static] |
Getter of mManualClusterDescription.
Returns value of mManualClusterDescription.
- Returns:
- The value of mManualClusterDescription
Definition at line 1479 of file PCMApplication.h.
const std::string & ParCompMark::Application::getOutput | ( | ) | [inline, static] |
Getter of mOutput.
Returns value of mOutput.
- Returns:
- The value of mOutput
Definition at line 1521 of file PCMApplication.h.
OutputNode::Pointer & ParCompMark::Application::getOutputDocument | ( | ) | [inline] |
Getter of mOutputDocument.
Returns value of mOutputDocument.
- Returns:
- The value of mOutputDocument
Definition at line 1367 of file PCMApplication.h.
const bool & ParCompMark::Application::getOutputIsDone | ( | ) | const [inline] |
Getter of mOutputIsDone.
Returns value of mOutputIsDone.
- Returns:
- The value of mOutputIsDone
Definition at line 1360 of file PCMApplication.h.
const std::string & ParCompMark::Application::getParameters | ( | ) | [inline, static] |
Getter of mParameters.
Returns value of mParameters.
- Returns:
- The value of mParameters
Definition at line 1507 of file PCMApplication.h.
const Application::PostCommand & ParCompMark::Application::getPostCommand | ( | ) | const [inline] |
Getter of mPostCommand.
Returns value of mPostCommand.
- Returns:
- The value of mPostCommand
Definition at line 1346 of file PCMApplication.h.
char * ParCompMark::Application::getProgramInfo | ( | ) | [virtual] |
Return one line program info as a string.
- Returns:
- Program info.
Definition at line 954 of file PCMApplication.cpp.
References PACKAGE_COPYRIGHT, and PACKAGE_STRING.
Referenced by showHelp(), and squirrelGlue().
const bool & ParCompMark::Application::getQuiting | ( | ) | const [inline] |
Getter of mQuiting.
Returns value of mQuiting.
- Returns:
- The value of mQuiting
Definition at line 1353 of file PCMApplication.h.
const std::string & ParCompMark::Application::getScenarioScript | ( | ) | const [inline] |
Getter of mScenarioScript.
Returns value of mScenarioScript.
- Returns:
- The value of mScenarioScript
Definition at line 1416 of file PCMApplication.h.
NetServer * ParCompMark::Application::getServer | ( | ) | const [inline] |
Getter of mServer.
Returns value of mServer.
- Returns:
- The value of mServer
Definition at line 1444 of file PCMApplication.h.
const std::list< std::string > & ParCompMark::Application::getSquirrelCommandList | ( | ) | const [inline] |
Getter of mSquirrelCommandList.
Returns value of mSquirrelCommandList.
- Returns:
- The value of mSquirrelCommandList
Definition at line 1423 of file PCMApplication.h.
const std::string & ParCompMark::Application::getUsageString | ( | ) | [inline, static] |
Getter of mUsageString.
Returns value of mUsageString.
- Returns:
- The value of mUsageString
Definition at line 1458 of file PCMApplication.h.
const Application::UserInterface & ParCompMark::Application::getUserInterface | ( | ) | [inline, static] |
Getter of mUserInterface.
Returns value of mUserInterface.
- Returns:
- The value of mUserInterface
Definition at line 1535 of file PCMApplication.h.
bool ParCompMark::Application::hasEnvironmentVariable | ( | const std::string & | name | ) | [static] |
Returns true if specified environment variable exists.
- Parameters:
-
[in] name Name of the variable.
- Returns:
- Indicates that the specified environment variable exists.
Definition at line 379 of file PCMApplication.cpp.
Referenced by initialize().
bool ParCompMark::Application::hasScenarioScript | ( | ) | [virtual] |
Return true if a scenario script is loaded.
- Returns:
- Flag indicates that the scenario script is loaded.
Definition at line 931 of file PCMApplication.cpp.
References mScenarioScript.
Referenced by squirrelGlue().
void ParCompMark::Application::initialize | ( | ) | [virtual] |
Initialize the PCM application.
Definition at line 484 of file PCMApplication.cpp.
References Assert, complement(), ParCompMark::Singleton< T >::createInstance(), ParCompMark::Logger::DEBUG, Except, getEnvironmentVariable(), getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::FileSystemManager::getInstance(), hasEnvironmentVariable(), ParCompMark::OutputNode::INFORMATION, ParCompMark::FileSystemManager::initialize(), mCollectClusterDescription, mConfigOptions, mCurrentExecutionOutputDocument, mExecutionIndex, mInitialized, mLibrarySearchPath, mOutputDocument, PCIMPLEMENTATIONS, setFromConfigOptions(), ParCompMark::Timer::setTimeCorrection(), setupHandlers(), ParCompMark::StringConverter::tokenize(), and ParCompMark::Logger::WARNING.
Referenced by Application().
Here is the call graph for this function:
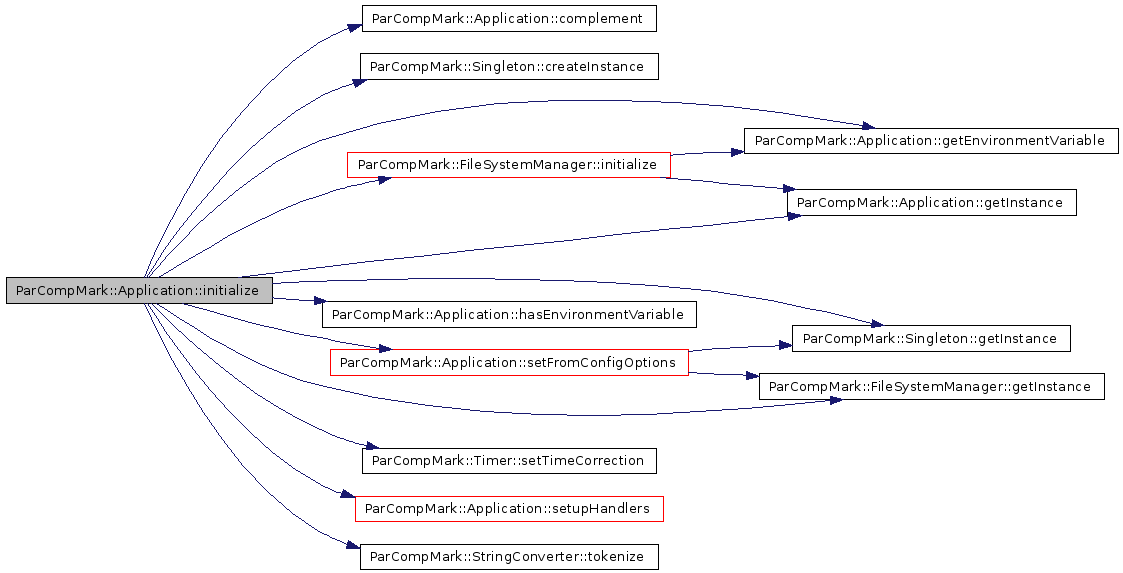
void ParCompMark::Application::initializeDynamicScriptParameters | ( | const bool & | reset | ) | [protected, virtual] |
Compile dynamic script and initialize dynamic script parameters in reset or complement mode.
- Parameters:
-
[in] reset Reset dynamic script parameters.
Definition at line 1397 of file PCMApplication.cpp.
References ParCompMark::Pointer< T, Lock >::lock(), mDynamicScript, mDynamicScriptSqVM, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by _loadDynamic().
Here is the call graph for this function:

void ParCompMark::Application::interruptHandler | ( | int | value | ) | [static] |
Interrupt signal handler.
- Parameters:
-
[in] value Signal parameter.
Definition at line 443 of file PCMApplication.cpp.
References _quit(), getInstance(), ParCompMark::Singleton< T >::getInstance(), and ParCompMark::Logger::NOTICE.
Referenced by setupHandlers().
Here is the call graph for this function:

void ParCompMark::Application::loadDynamicScript | ( | const char * | filename | ) | [protected, virtual] |
Load dynamic script from the specified filename.
- Parameters:
-
[in] filename File containing dynamic script (in application data directory).
Definition at line 1261 of file PCMApplication.cpp.
References ParCompMark::FileSystemManager::getInstance(), LOADDYNAMIC, mDynamicScript, mDynamicScriptCompiled, mPostCommand, and ParCompMark::FileSystemManager::readDataTextFile().
Referenced by commanderOperation(), and squirrelGlue().
Here is the call graph for this function:

void ParCompMark::Application::loadLowLevelScript | ( | const char * | filename | ) | [protected, virtual] |
Load low-level script from the specified filename.
- Parameters:
-
[in] filename File containing low level script (in application data directory).
Definition at line 1254 of file PCMApplication.cpp.
References ParCompMark::FileSystemManager::getInstance(), mLowLevelScript, and ParCompMark::FileSystemManager::readDataTextFile().
Referenced by commanderOperation(), and squirrelGlue().
Here is the call graph for this function:

void ParCompMark::Application::loadScenarioScript | ( | const char * | filename | ) | [protected, virtual] |
Load scenario script from the specified filename.
- Parameters:
-
[in] filename File containing scenario script (in application data directory).
Definition at line 1274 of file PCMApplication.cpp.
References ParCompMark::FileSystemManager::getInstance(), LOADSCENARIO, mPostCommand, mScenarioScript, and ParCompMark::FileSystemManager::readDataTextFile().
Referenced by commanderOperation(), and squirrelGlue().
Here is the call graph for this function:

bool ParCompMark::Application::noUserInterface | ( | ) | [protected, virtual] |
Start "no" user interface.
- Returns:
- Return true on error.
Definition at line 1117 of file PCMApplication.cpp.
References doPostCommand(), executeUserCommand(), mCompRun, mQuiting, processSquirrelCommandList(), and ParCompMark::Timer::sleep().
Referenced by startOperation().
Here is the call graph for this function:

void ParCompMark::Application::parseCommandLine | ( | const u32 & | argc, | |
const char **& | argv | |||
) | [static] |
Parse ANSI C command line.
- Parameters:
-
[in] argc Number of command line arguments. [in] argv Array of command line arguments.
Definition at line 193 of file PCMApplication.cpp.
Referenced by main().
void ParCompMark::Application::processScenarioScript | ( | ) | [protected, virtual] |
Process scenario script file.
Definition at line 1323 of file PCMApplication.cpp.
References ParCompMark::Pointer< T, Lock >::getLocked(), ParCompMark::Exception::getType(), ParCompMark::Pointer< T, Lock >::lock(), mDynamicScriptCompiled, mDynamicScriptSqVM, mScenarioScript, ParCompMark::Exception::SCRIPT_ERROR, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by _loadScenario().
Here is the call graph for this function:

bool ParCompMark::Application::processSquirrelCommandList | ( | ) | [protected, virtual] |
Executes the commands in the squirrel command list.
- Returns:
- Return true on error.
Definition at line 1197 of file PCMApplication.cpp.
References doPostCommand(), executeUserCommand(), mCompRun, mSquirrelCommandList, and ParCompMark::Timer::sleep().
Referenced by noUserInterface(), and textUserInterface().
Here is the call graph for this function:

void ParCompMark::Application::pushSquirrelCommand | ( | const char * | command | ) | [protected, virtual] |
Push a command into the Squirrel command list.
- Parameters:
-
[in] command Command line to push.
Definition at line 1284 of file PCMApplication.cpp.
References mSquirrelCommandList.
Referenced by squirrelGlue().
void ParCompMark::Application::quit | ( | ) | [virtual] |
Quit from application.
Definition at line 839 of file PCMApplication.cpp.
References mPostCommand, QUIT, STOP, and STOP_QUIT.
Referenced by squirrelGlue().
void ParCompMark::Application::retrieveDynamicScriptParameters | ( | ) | [protected, virtual] |
Retrieve dynamic script parameters from the loaded dynamic script.
Definition at line 1408 of file PCMApplication.cpp.
References Assert, BOOL, ParCompMark::Application::DynamicScriptParameter::defaultValue, ParCompMark::Application::DynamicScriptParameter::description, Except, ParCompMark::Pointer< T, Lock >::getLocked(), ParCompMark::Exception::getType(), INTEGER, ParCompMark::Pointer< T, Lock >::lock(), mDynamicScript, mDynamicScriptParameters, mDynamicScriptSqVM, ParCompMark::Application::DynamicScriptParameter::name, REAL, ParCompMark::Exception::SCRIPT_ERROR, STRING, ParCompMark::Application::DynamicScriptParameter::type, and ParCompMark::Pointer< T, Lock >::unlock().
Here is the call graph for this function:

void ParCompMark::Application::segfaultHandler | ( | int | value | ) | [static] |
Segmentation fault handler.
- Parameters:
-
[in] value Signal parameter.
Definition at line 435 of file PCMApplication.cpp.
void ParCompMark::Application::setCluster | ( | const std::string & | strarg | ) | [static] |
Set cluster description file.
- Parameters:
-
[in] strarg String argument.
Definition at line 325 of file PCMApplication.cpp.
References mClusterDescription, and mManualClusterDescription.
void ParCompMark::Application::setCommanderOn | ( | const std::string & | strarg | ) | [static] |
Set commander mode.
- Parameters:
-
[in] strarg String argument (dummy).
Definition at line 283 of file PCMApplication.cpp.
References mCommanderMode.
void ParCompMark::Application::setDynamicScriptCompiled | ( | const bool | dynamicscriptcompiled | ) | [inline] |
Setter of mDynamicScriptCompiled.
Sets value of mDynamicScriptCompiled.
- Parameters:
-
[in] dynamicscriptcompiled The value of mDynamicScriptCompiled
Definition at line 1437 of file PCMApplication.h.
Referenced by squirrelGlue().
void ParCompMark::Application::setDynamicScriptFile | ( | const std::string & | strarg | ) | [static] |
Set dynamic script file.
- Parameters:
-
[in] strarg String argument.
Definition at line 311 of file PCMApplication.cpp.
References getConfigOptions(), and getInstance().
Here is the call graph for this function:

void ParCompMark::Application::setEnvironmentVariablesToSquirrel | ( | ) | [protected, virtual] |
Set environment variables to the Squirrel.
Definition at line 1352 of file PCMApplication.cpp.
References ParCompMark::Host::getFirstXDisplay(), ParCompMark::Network::getHostName(), ParCompMark::Host::getInstance(), ParCompMark::Pointer< T, Lock >::getPtr(), ParCompMark::Pointer< T, Lock >::lock(), mDynamicScript, mDynamicScriptSqVM, ParCompMark::StringConverter::toString(), and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by _loadDynamic().
Here is the call graph for this function:

void ParCompMark::Application::setExpectedHostCount | ( | const std::string & | hostCount | ) | [static] |
Set expected number of rendering hosts.
- Parameters:
-
[in] hostCount String argument.
Definition at line 355 of file PCMApplication.cpp.
References mExpectedHostCount, and ParCompMark::StringConverter::toU32().
Here is the call graph for this function:

void ParCompMark::Application::setFromConfigOptions | ( | ) | [virtual] |
Call application-wide setters on config options.
Definition at line 658 of file PCMApplication.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), ParCompMark::FileSystemManager::getInstance(), mConfigOptions, ParCompMark::Network::setBroadcastPort(), ParCompMark::Network::setCommunicationPort(), ParCompMark::FileSystemManager::setDataDirectory(), and setLibrarySearchPath().
Referenced by initialize().
Here is the call graph for this function:
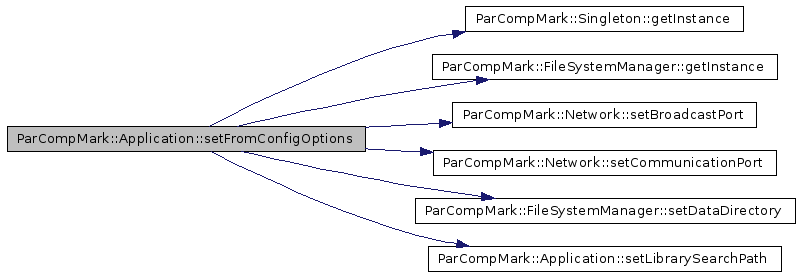
void ParCompMark::Application::setGUIOn | ( | const std::string & | strarg | ) | [static] |
Set GUI mode.
- Parameters:
-
[in] strarg String argument (dummy).
Definition at line 290 of file PCMApplication.cpp.
References mGUIMode.
void ParCompMark::Application::setInput | ( | const std::string & | strarg | ) | [static] |
Set input file.
- Parameters:
-
[in] strarg String argument.
Definition at line 341 of file PCMApplication.cpp.
References mInput.
void ParCompMark::Application::setLibrarySearchPath | ( | const std::string & | librarysearchpath | ) | [inline, static] |
Setter of mLibrarySearchPath.
Sets value of mLibrarySearchPath.
- Parameters:
-
[in] librarysearchpath The value of mLibrarySearchPath
Definition at line 1325 of file PCMApplication.h.
Referenced by setFromConfigOptions().
void ParCompMark::Application::setLowLevelOn | ( | const std::string & | strarg | ) | [static] |
Set low-level scripting mode.
- Parameters:
-
[in] strarg String argument (dummy).
Definition at line 297 of file PCMApplication.cpp.
References mLowLevelMode.
void ParCompMark::Application::setLowLevelScriptFile | ( | const std::string & | strarg | ) | [static] |
Set low-level script file.
- Parameters:
-
[in] strarg String argument.
Definition at line 304 of file PCMApplication.cpp.
References getConfigOptions(), and getInstance().
Here is the call graph for this function:

void ParCompMark::Application::setOutput | ( | const std::string & | strarg | ) | [static] |
Set output file.
- Parameters:
-
[in] strarg String argument.
Definition at line 348 of file PCMApplication.cpp.
References mOutput.
void ParCompMark::Application::setParameters | ( | const std::string & | strarg | ) | [static] |
Set parameters description file.
- Parameters:
-
[in] strarg String argument.
Definition at line 333 of file PCMApplication.cpp.
References mInteractiveParameters, and mParameters.
void ParCompMark::Application::setScenarioScriptFile | ( | const std::string & | strarg | ) | [static] |
Set scenario script file.
- Parameters:
-
[in] strarg String argument.
Definition at line 318 of file PCMApplication.cpp.
References getConfigOptions(), and getInstance().
Here is the call graph for this function:

void ParCompMark::Application::setupHandlers | ( | ) | const [virtual] |
Setup special event handlers.
Definition at line 471 of file PCMApplication.cpp.
References interruptHandler(), terminateHandler(), and unexpectedHandler().
Referenced by initialize().
Here is the call graph for this function:

void ParCompMark::Application::setUserInterface | ( | const std::string & | strUI | ) | [static] |
Set user the type of interface.
- Parameters:
-
[in] strUI String argument.
Definition at line 365 of file PCMApplication.cpp.
References CONSOLE, Except, mUserInterface, and NONE.
void ParCompMark::Application::showHelp | ( | const std::string & | strarg | ) | [static] |
Write help to std out.
- Parameters:
-
[in] strarg String argument (dummy).
Definition at line 248 of file PCMApplication.cpp.
References _quit(), getInstance(), getProgramInfo(), mCommandLineOptionCount, mUsageString, and PACKAGE_BUGREPORT.
Here is the call graph for this function:

void ParCompMark::Application::showVersion | ( | const std::string & | strarg | ) | [static] |
Write version to std out.
- Parameters:
-
[in] strarg String argument (dummy).
Definition at line 273 of file PCMApplication.cpp.
References _quit(), getInstance(), and PACKAGE_VERSION.
Here is the call graph for this function:

bool ParCompMark::Application::soldierOperation | ( | ) | [protected, virtual] |
Operation of a soldier mode (not commander mode) application.
- Returns:
- Return true on error.
Definition at line 1043 of file PCMApplication.cpp.
References ParCompMark::Singleton< T >::createInstance(), ParCompMark::Logger::FATAL, ParCompMark::Network::getHostName(), ParCompMark::Host::getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::OutputNode::INFORMATION, mClient, mHostInfo, ParCompMark::Logger::NOTICE, ParCompMark::Host::openXDisplays(), ParCompMark::HostInfo::refreshData(), ParCompMark::Host::setNetClient(), and ParCompMark::Thread::startThread().
Referenced by startOperation().
Here is the call graph for this function:

static void ParCompMark::Application::squirrelGlue | ( | ) | [inline, static] |
Static glue code method for Squirrel-C++ binding.
This method should be call from each Squirrel virtual machines to generate the proper Squirrel glue code. To content of this method is fully autogenerated in the header implementation file, you should not modify it.
Definition at line 111 of file PCMApplication.h.
References autoDetection(), cleanup(), compileDynamic(), getCompRun(), getDynamicScriptCompiled(), getDynamicScriptForSquirrel(), getDynamicScriptParametersForSquirrel(), getExpectedHostCount(), getHostListForSquirrel(), getInstance(), getLowLevelScriptForSquirrel(), getProgramInfo(), hasScenarioScript(), loadDynamicScript(), loadLowLevelScript(), loadScenarioScript(), pushSquirrelCommand(), quit(), setDynamicScriptCompiled(), start(), and stop().
Referenced by ParCompMark::squirrelClassBindings().
Here is the call graph for this function:

void ParCompMark::Application::start | ( | ) | [virtual] |
Start compositing.
Definition at line 853 of file PCMApplication.cpp.
References COMPILE, COMPILE_START, mPostCommand, and START.
Referenced by squirrelGlue().
bool ParCompMark::Application::startOperation | ( | ) | [virtual] |
The application starts its operation.
The operation depends on the commander mode flag.
- Returns:
- Return true on error.
Definition at line 733 of file PCMApplication.cpp.
References commanderOperation(), CONSOLE, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Thread::joinThread(), mClient, mCommanderMode, mUserInterface, ParCompMark::Logger::NOTICE, noUserInterface(), soldierOperation(), textUserInterface(), and waitForOutput().
Referenced by main().
Here is the call graph for this function:

void ParCompMark::Application::stop | ( | ) | [virtual] |
Stop compositing.
Definition at line 860 of file PCMApplication.cpp.
References mPostCommand, QUIT, STOP, and STOP_QUIT.
Referenced by squirrelGlue().
void ParCompMark::Application::terminateHandler | ( | ) | [static] |
Abnormal termination handler.
Definition at line 400 of file PCMApplication.cpp.
References ParCompMark::Logger::FATAL, finalize(), getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Exception::getLastException(), terminateHandlerNOP(), and ParCompMark::Logger::translateException().
Referenced by setupHandlers(), and unexpectedHandler().
Here is the call graph for this function:
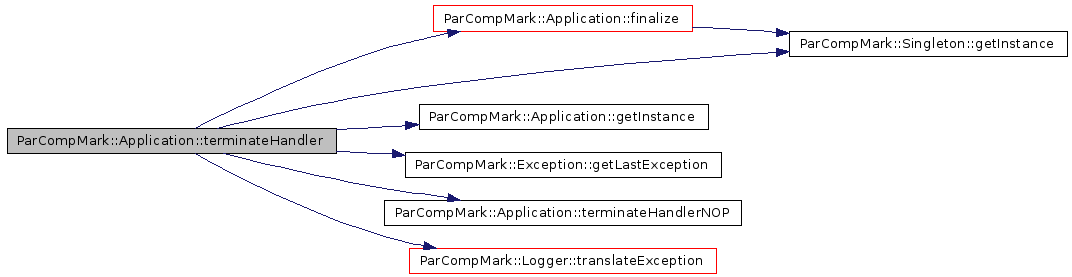
void ParCompMark::Application::terminateHandlerNOP | ( | ) | [static] |
No operation termination handler.
The terminateHandler() method sets this method as Abnormal termination handler during its run.
Definition at line 421 of file PCMApplication.cpp.
Referenced by terminateHandler().
bool ParCompMark::Application::textUserInterface | ( | ) | [protected, virtual] |
Start textual user interface.
- Returns:
- Return true on error.
Definition at line 1076 of file PCMApplication.cpp.
References doPostCommand(), executeUserCommand(), HAVE_READLINE_HISTORY_H, HAVE_READLINE_READLINE_H, mQuiting, and processSquirrelCommandList().
Referenced by startOperation().
Here is the call graph for this function:

void ParCompMark::Application::unexpectedHandler | ( | ) | [static] |
Unexpected exception handler.
Definition at line 428 of file PCMApplication.cpp.
References terminateHandler().
Referenced by setupHandlers().
Here is the call graph for this function:

void ParCompMark::Application::waitForOutput | ( | ) | [virtual] |
Wait until the output is fully written.
Definition at line 828 of file PCMApplication.cpp.
References mOutputIsDone, ParCompMark::Timer::sleep(), and ParCompMark::Thread::yield().
Referenced by startOperation().
Here is the call graph for this function:

void ParCompMark::Application::writeOutput | ( | ) | [virtual] |
Write collected output.
Definition at line 787 of file PCMApplication.cpp.
References ParCompMark::Singleton< T >::getInstance(), ParCompMark::Pointer< T, Lock >::lock(), mCollectClusterDescription, mOutput, mOutputDocument, mOutputIsDone, ParCompMark::Logger::NOTICE, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by _quit().
Here is the call graph for this function:
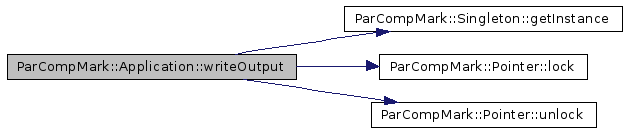
Member Data Documentation
const std::string ParCompMark::Application::ABBREVIATIONSNUT = "scripts/framework/abbreviations.nut" [static] |
User command abbreviations resolver Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 280 of file PCMApplication.h.
Referenced by createVirtualMachines().
const std::string ParCompMark::Application::COMMANDDIRECTORY = "scripts/commands/" [static] |
Directory that contatins user commands in separated nut files (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 301 of file PCMApplication.h.
Referenced by createVirtualMachines().
const std::string ParCompMark::Application::DYNAMICINITNUT = "scripts/framework/dynamic-init.nut" [static] |
Dynamic script initialization Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 287 of file PCMApplication.h.
Referenced by createVirtualMachines().
const std::string ParCompMark::Application::HELPNUT = "scripts/commands/help.nut" [static] |
Path of help.nut file in user commands directory (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 308 of file PCMApplication.h.
Referenced by createVirtualMachines().
NetClient* ParCompMark::Application::mClient [protected] |
Client network.
- Remarks:
- This is own attribute of this class.
Definition at line 565 of file PCMApplication.h.
Referenced by Application(), finalizeSoldier(), soldierOperation(), and startOperation().
std::string ParCompMark::Application::mClusterDescription [static, protected] |
Cluster description file name.
- Remarks:
- This is own attribute of this class.
Definition at line 384 of file PCMApplication.h.
Referenced by setCluster().
Root of the output document.
- Remarks:
- This is own attribute of this class.
Definition at line 507 of file PCMApplication.h.
Referenced by _start(), initialize(), and writeOutput().
bool ParCompMark::Application::mCommanderMode = false [static, protected] |
Indicates commander mode (default false).
- Remarks:
- This is own attribute of this class.
Definition at line 363 of file PCMApplication.h.
Referenced by commanderOperation(), finalize(), setCommanderOn(), and startOperation().
const u32 ParCompMark::Application::mCommandLineOptionCount = sizeof(mCommandLineOptions) / sizeof(mCommandLineOptions[0]) [static, protected] |
Number of command line options.
- Remarks:
- This is own attribute of this class.
Definition at line 331 of file PCMApplication.h.
Referenced by showHelp().
const Application::CommandLineOption ParCompMark::Application::mCommandLineOptions [static, protected] |
Initial value:
{ {'h', "help", " ........... : show help", false, Application::showHelp} , {'v', "version", " ........ : show version", false, Application::showVersion} , {'c', "commander", " ...... : enable commander mode", false, Application::setCommanderOn} , {'l', "low-level", " ...... : set low-level script file", true, Application::setLowLevelScriptFile} , {'d', "dynamic", " ........ : set cluster dynamic script file", true, Application::setDynamicScriptFile} , {'s', "scenario", " ....... : set scenario script file", true, Application::setScenarioScriptFile} , {'i', "input", " .......... : set intput script file", true, Application::setInput} , {'o', "output", " ......... : set output filename", true, Application::setOutput} , {'H', "hostcount", " ...... : set expected number of rendering hosts", true, Application::setExpectedHostCount} , {'u', "ui", " ............. : set user interface (none|console)", true, Application::setUserInterface} }
- Remarks:
- This is own attribute of this class.
Definition at line 324 of file PCMApplication.h.
bool ParCompMark::Application::mCompRun [protected] |
Output file name.
- Remarks:
- This is own attribute of this class.
Definition at line 572 of file PCMApplication.h.
Referenced by _pseudoStop(), _quit(), _start(), _stop(), Application(), autoDetection(), noUserInterface(), and processSquirrelCommandList().
Program configuration options.
- Remarks:
- This is own attribute of this class.
Definition at line 514 of file PCMApplication.h.
Referenced by commanderOperation(), finalize(), initialize(), and setFromConfigOptions().
The output document has separate 'execution' children for each benchmark execution.
This pointer addresses the actual execution.
- Remarks:
- This is own attribute of this class.
Definition at line 493 of file PCMApplication.h.
Referenced by _start(), cleanup(), and initialize().
std::string ParCompMark::Application::mDynamicScript [protected] |
Squirrel cluster dynamic script.
- Remarks:
- This is own attribute of this class.
Definition at line 528 of file PCMApplication.h.
Referenced by _compileDynamic(), createLowLevelScript(), getDynamicScriptForSquirrel(), initializeDynamicScriptParameters(), loadDynamicScript(), retrieveDynamicScriptParameters(), and setEnvironmentVariablesToSquirrel().
bool ParCompMark::Application::mDynamicScriptCompiled [protected] |
Indicates that the dynamic script is compiled.
- Remarks:
- This is own attribute of this class.
Definition at line 551 of file PCMApplication.h.
Referenced by autoDetection(), createLowLevelScript(), loadDynamicScript(), and processScenarioScript().
std::list< DynamicScriptParameter * > ParCompMark::Application::mDynamicScriptParameters [protected] |
List of dynamic script parameters.
- Remarks:
- This is own attribute of this class.
Definition at line 593 of file PCMApplication.h.
Referenced by getDynamicScriptParametersForSquirrel(), and retrieveDynamicScriptParameters().
Squirrel virtual machine for dynamic script execution.
- Remarks:
- This is own attribute of this class.
Definition at line 579 of file PCMApplication.h.
Referenced by _start(), createLowLevelScript(), createVirtualMachines(), finalize(), initializeDynamicScriptParameters(), processScenarioScript(), retrieveDynamicScriptParameters(), and setEnvironmentVariablesToSquirrel().
u32 ParCompMark::Application::mExecutionIndex [protected] |
Index of the actual execution.
- Remarks:
- This is own attribute of this class.
Definition at line 500 of file PCMApplication.h.
Referenced by _start(), and initialize().
int ParCompMark::Application::mExpectedHostCount = 0 [static, protected] |
Expected number of rendering hosts.
- Remarks:
- This is own attribute of this class.
Definition at line 426 of file PCMApplication.h.
Referenced by setExpectedHostCount().
bool ParCompMark::Application::mGUIMode = false [static, protected] |
Indicates GUI mode (default false).
- Remarks:
- This is own attribute of this class.
Definition at line 370 of file PCMApplication.h.
Referenced by setGUIOn().
HostInfo* ParCompMark::Application::mHostInfo [protected] |
Information about the host.
Soldire opertaion fills it.
- Remarks:
- This is own attribute of this class.
Definition at line 451 of file PCMApplication.h.
Referenced by soldierOperation().
bool ParCompMark::Application::mInitialized [protected] |
The application is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 458 of file PCMApplication.h.
Referenced by Application(), finalize(), initialize(), and ~Application().
std::string ParCompMark::Application::mInput = "-" [static, protected] |
Input script file name.
- Remarks:
- This is own attribute of this class.
Definition at line 412 of file PCMApplication.h.
Referenced by setInput().
bool ParCompMark::Application::mInteractiveParameters = true [static, protected] |
Indicates interactive parameter settings (default true).
- Remarks:
- This is own attribute of this class.
Definition at line 398 of file PCMApplication.h.
Referenced by setParameters().
std::string ParCompMark::Application::mLibrarySearchPath = "/usr/lib" [static, protected] |
PC dynamic library search path (default /usr/lib).
- Remarks:
- This is own attribute of this class.
Definition at line 356 of file PCMApplication.h.
Referenced by initialize().
bool ParCompMark::Application::mLowLevelMode = false [static, protected] |
Indicates low-level scripting mode (default false).
- Remarks:
- This is own attribute of this class.
Definition at line 391 of file PCMApplication.h.
Referenced by setLowLevelOn().
std::string ParCompMark::Application::mLowLevelScript [protected] |
Squirrel low-level script.
- Remarks:
- This is own attribute of this class.
Definition at line 521 of file PCMApplication.h.
Referenced by _start(), createLowLevelScript(), getLowLevelScriptForSquirrel(), and loadLowLevelScript().
bool ParCompMark::Application::mManualClusterDescription = false [static, protected] |
Indicates manual cluster description (default false).
- Remarks:
- This is own attribute of this class.
Definition at line 377 of file PCMApplication.h.
Referenced by setCluster().
std::string ParCompMark::Application::mOutput = "-" [static, protected] |
Output file name.
- Remarks:
- This is own attribute of this class.
Definition at line 419 of file PCMApplication.h.
Referenced by setOutput(), and writeOutput().
Root of the output document.
- Remarks:
- This is own attribute of this class.
Definition at line 486 of file PCMApplication.h.
Referenced by _start(), cleanup(), finalize(), initialize(), and writeOutput().
bool ParCompMark::Application::mOutputIsDone [protected] |
Flag indicating that the output document has been written now and it is safe to quit.
- Remarks:
- This is own attribute of this class.
Definition at line 479 of file PCMApplication.h.
Referenced by _start(), Application(), waitForOutput(), and writeOutput().
std::string ParCompMark::Application::mParameters [static, protected] |
Parameters description file name.
- Remarks:
- This is own attribute of this class.
Definition at line 405 of file PCMApplication.h.
Referenced by setParameters().
PostCommand ParCompMark::Application::mPostCommand [protected] |
Current post command task.
- Remarks:
- This is own attribute of this class.
Definition at line 465 of file PCMApplication.h.
Referenced by Application(), compileDynamic(), doPostCommand(), loadDynamicScript(), loadScenarioScript(), quit(), start(), and stop().
bool ParCompMark::Application::mQuiting [protected] |
Flag indicating that the application is quitting now (to avoid multiple quit requests in quit() method).
- Remarks:
- This is own attribute of this class.
Definition at line 472 of file PCMApplication.h.
Referenced by _quit(), Application(), noUserInterface(), and textUserInterface().
std::string ParCompMark::Application::mScenarioScript [protected] |
Squirrel scenario script.
- Remarks:
- This is own attribute of this class.
Definition at line 535 of file PCMApplication.h.
Referenced by hasScenarioScript(), loadScenarioScript(), and processScenarioScript().
NetServer* ParCompMark::Application::mServer [protected] |
Server network.
- Remarks:
- This is own attribute of this class.
Definition at line 558 of file PCMApplication.h.
Referenced by _pseudoStop(), _quit(), _start(), _stop(), Application(), autoDetection(), calculateMessageSendTime(), commanderOperation(), and finalizeCommander().
std::list< std::string > ParCompMark::Application::mSquirrelCommandList [protected] |
List of Squirrel commands to execute.
If this list is not empty, the user interface take and executes these commands instead of putting command prompt. Moreover, you can put commands to this list from Squirrel scripts, too. This is useful for example scenario scripts.
- Remarks:
- This is own attribute of this class.
Definition at line 544 of file PCMApplication.h.
Referenced by processSquirrelCommandList(), and pushSquirrelCommand().
const std::string ParCompMark::Application::mUsageString = "ParCompMark [ options ]" [static, protected] |
Application usage string.
- Remarks:
- This is own attribute of this class.
Definition at line 338 of file PCMApplication.h.
Referenced by showHelp().
Application::UserInterface ParCompMark::Application::mUserInterface = CONSOLE [static, protected] |
Type of user interface.
- Remarks:
- This is own attribute of this class.
Definition at line 433 of file PCMApplication.h.
Referenced by setUserInterface(), and startOperation().
Squirrel virtual machine to handle user interaction.
- Remarks:
- This is own attribute of this class.
Definition at line 586 of file PCMApplication.h.
Referenced by commanderOperation(), createVirtualMachines(), executeUserCommand(), and finalize().
const std::string ParCompMark::Application::PARCOMPMARKNUT = "scripts/framework/parcompmark.nut" [static] |
ParCompMark API Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 266 of file PCMApplication.h.
Referenced by createVirtualMachines(), ParCompMark::Host::initialize(), and ParCompMark::Process::threadInitialize().
const std::string ParCompMark::Application::PCAPINUT = "scripts/framework/pcapi.nut" [static] |
PC API Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 259 of file PCMApplication.h.
Referenced by createVirtualMachines(), ParCompMark::Host::initialize(), and ParCompMark::Process::threadInitialize().
const std::string ParCompMark::Application::PCIMPLEMENTATIONS [static] |
Initial value:
{ "libpcapi.so", "libparcomp.so" }
- Remarks:
- This is own attribute of this class.
Definition at line 315 of file PCMApplication.h.
Referenced by initialize().
const std::string ParCompMark::Application::UIINITNUT = "scripts/framework/ui-init.nut" [static] |
User interface initialization Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 294 of file PCMApplication.h.
Referenced by commanderOperation().
const std::string ParCompMark::Application::UTILSNUT = "scripts/framework/utils.nut" [static] |
Common utilities Squirrel script file (relative to the data directory).
- Remarks:
- This is own attribute of this class.
Definition at line 273 of file PCMApplication.h.
Referenced by createVirtualMachines(), ParCompMark::Host::initialize(), and ParCompMark::Process::threadInitialize().
The documentation for this class was generated from the following files: