ParCompMark::Client Class Reference
#include <PCMClient.h>
Inheritance diagram for ParCompMark::Client:
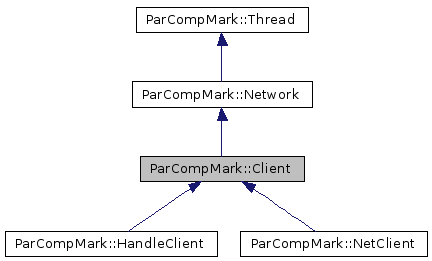
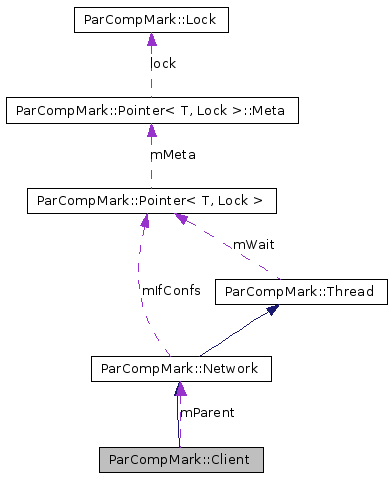
Detailed Description
Client functions.
Definition at line 54 of file PCMClient.h.
Public Types | |
typedef ParCompMark::Pointer< Client, DummyLock > | Pointer |
Type for pointer to this class. | |
Public Member Functions | |
Constructors & destructor | |
Client (const std::string &name) | |
Create network class. | |
virtual | ~Client () |
The destructor. | |
Getters & setters | |
const std::string & | getServerIP () const |
Getter of mServerIP. | |
void | setServerIP (const std::string &serverip) |
Setter of mServerIP. | |
const std::string & | getType () const |
Getter of mType. | |
void | setType (const std::string &type) |
Setter of mType. | |
Network * | getParent () const |
Getter of mParent. | |
const std::string & | getMessage () const |
Getter of mMessage. | |
void | setMessage (const std::string &message) |
Setter of mMessage. | |
Methods | |
virtual void | openConnection () |
Open TCP/IP connection (mServerIP). | |
virtual void | closeConnection () |
Close TCP/IP connection (mServerIP). | |
virtual void | sendMessage (const std::string &type, const std::string &message) |
Send message with TCP protocol. | |
virtual void | recieveMessage () |
Wait for TCP/IP message. | |
virtual void | handleMessage () |
Handle TCP/IP message. | |
Protected Attributes | |
Variables | |
std::string | mServerIP |
IP address of the server host. | |
std::string | mType |
IP address of the server host. | |
Network * | mParent |
The parent network. | |
std::string | mMessage |
IP address of the server host. |
Member Typedef Documentation
Type for pointer to this class.
Reimplemented from ParCompMark::Network.
Reimplemented in ParCompMark::HandleClient, and ParCompMark::NetClient.
Definition at line 72 of file PCMClient.h.
Constructor & Destructor Documentation
ParCompMark::Client::Client | ( | const std::string & | name | ) |
Create network class.
- Parameters:
-
[in] name Name of the network.
Definition at line 40 of file PCMClient.cpp.
References mParent.
ParCompMark::Client::~Client | ( | ) | [virtual] |
Member Function Documentation
void ParCompMark::Client::closeConnection | ( | ) | [virtual] |
Close TCP/IP connection (mServerIP).
Definition at line 97 of file PCMClient.cpp.
References Assert, and ParCompMark::Network::mStreamSocket.
Referenced by handleMessage().
const std::string & ParCompMark::Client::getMessage | ( | ) | const [inline] |
Getter of mMessage.
Returns value of mMessage.
- Returns:
- The value of mMessage
Definition at line 305 of file PCMClient.h.
Network * ParCompMark::Client::getParent | ( | ) | const [inline] |
Getter of mParent.
Returns value of mParent.
- Returns:
- The value of mParent
Definition at line 298 of file PCMClient.h.
const std::string & ParCompMark::Client::getServerIP | ( | ) | const [inline] |
Getter of mServerIP.
Returns value of mServerIP.
- Returns:
- The value of mServerIP
Definition at line 270 of file PCMClient.h.
const std::string & ParCompMark::Client::getType | ( | ) | const [inline] |
Getter of mType.
Returns value of mType.
- Returns:
- The value of mType
Definition at line 284 of file PCMClient.h.
void ParCompMark::Client::handleMessage | ( | ) | [virtual] |
Handle TCP/IP message.
Definition at line 282 of file PCMClient.cpp.
References closeConnection(), ParCompMark::Host::collectData(), ParCompMark::Logger::DEBUG, Except, ParCompMark::Network::getHostName(), ParCompMark::Application::getInstance(), ParCompMark::Host::getInstance(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Network::getIP(), ParCompMark::Host::getOutputDocument(), ParCompMark::Pointer< T, Lock >::getPtr(), ParCompMark::Timer::getUncorrectedSystemTime(), ParCompMark::OutputNode::INFORMATION, ParCompMark::Host::initialize(), ParCompMark::Pointer< T, Lock >::lock(), mMessage, mParent, ParCompMark::Network::mStreamSocket, mType, openConnection(), recieveMessage(), sendMessage(), ParCompMark::Host::setFrameID(), ParCompMark::Host::setID(), ParCompMark::Host::setLowLevelScript(), ParCompMark::Host::setMessageSendingTime(), ParCompMark::Host::setName(), ParCompMark::Host::start(), ParCompMark::Host::stop(), ParCompMark::Thread::stopThread(), ParCompMark::StringConverter::toString(), ParCompMark::StringConverter::toU32(), and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by ParCompMark::NetClient::task(), and ParCompMark::HandleClient::task().
Here is the call graph for this function:

void ParCompMark::Client::openConnection | ( | ) | [virtual] |
Open TCP/IP connection (mServerIP).
Reimplemented in ParCompMark::HandleClient.
Definition at line 65 of file PCMClient.cpp.
References Assert, Except, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Network::mCommunicationPort, mServerIP, ParCompMark::Network::mStreamSocket, and ParCompMark::Logger::NOTICE.
Referenced by handleMessage().
Here is the call graph for this function:

void ParCompMark::Client::recieveMessage | ( | ) | [virtual] |
Wait for TCP/IP message.
Definition at line 164 of file PCMClient.cpp.
References Assert, ParCompMark::Logger::DEBUG, Except, ParCompMark::Singleton< T >::getInstance(), mMessage, ParCompMark::Network::mStreamSocket, mType, and ParCompMark::StringConverter::toString().
Referenced by handleMessage(), and ParCompMark::HandleClient::task().
Here is the call graph for this function:

void ParCompMark::Client::sendMessage | ( | const std::string & | type, | |
const std::string & | message | |||
) | [virtual] |
Send message with TCP protocol.
- Parameters:
-
[in] type Type of the message. [in] message The message.
Definition at line 108 of file PCMClient.cpp.
References Assert, Except, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Network::mStreamSocket, and ParCompMark::Logger::NOTICE.
Referenced by handleMessage().
Here is the call graph for this function:

void ParCompMark::Client::setMessage | ( | const std::string & | message | ) | [inline] |
Setter of mMessage.
Sets value of mMessage.
- Parameters:
-
[in] message The value of mMessage
Definition at line 312 of file PCMClient.h.
void ParCompMark::Client::setServerIP | ( | const std::string & | serverip | ) | [inline] |
Setter of mServerIP.
Sets value of mServerIP.
- Parameters:
-
[in] serverip The value of mServerIP
Definition at line 277 of file PCMClient.h.
Referenced by ParCompMark::NetServer::task().
void ParCompMark::Client::setType | ( | const std::string & | type | ) | [inline] |
Setter of mType.
Sets value of mType.
- Parameters:
-
[in] type The value of mType
Definition at line 291 of file PCMClient.h.
Member Data Documentation
std::string ParCompMark::Client::mMessage [protected] |
IP address of the server host.
- Remarks:
- This is own attribute of this class.
Definition at line 109 of file PCMClient.h.
Referenced by handleMessage(), recieveMessage(), and ParCompMark::NetClient::task().
Network* ParCompMark::Client::mParent [protected] |
The parent network.
- Remarks:
- This attribute references an attribute.
Definition at line 102 of file PCMClient.h.
Referenced by Client(), ParCompMark::HandleClient::HandleClient(), handleMessage(), and ParCompMark::HandleClient::task().
std::string ParCompMark::Client::mServerIP [protected] |
IP address of the server host.
- Remarks:
- This is own attribute of this class.
Definition at line 88 of file PCMClient.h.
Referenced by openConnection(), and ParCompMark::NetClient::task().
std::string ParCompMark::Client::mType [protected] |
IP address of the server host.
- Remarks:
- This is own attribute of this class.
Definition at line 95 of file PCMClient.h.
Referenced by handleMessage(), recieveMessage(), and ParCompMark::NetClient::task().
The documentation for this class was generated from the following files: