ParCompMark::Plugin Class Reference
#include <PCMPlugin.h>
Inheritance diagram for ParCompMark::Plugin:
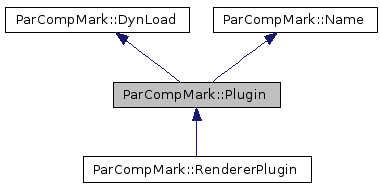
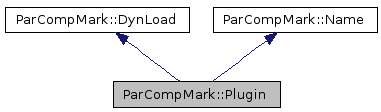
Detailed Description
Application plugin.
Definition at line 60 of file PCMPlugin.h.
Methods | |
virtual void | initialize () |
Initialize the plugin. | |
virtual void | finalize () |
Finalize the plugin. | |
virtual void | _initializeSpecific ()=0 |
Specific initialization code. | |
virtual void | _finalizeSpecific ()=0 |
Specific finalization code. | |
virtual void | _getNeededLibs () |
Get needed libraries from the plugin. | |
virtual void | _setPluginHandle () |
Set plugin handle to the plugin. | |
virtual void | _setLoggerFunction () |
Set logger function to the plugin. | |
virtual void | _onLoad () |
Call pcmOnLoad event handler of the plugin. | |
virtual void | _onUnload () |
Call pcmOnUnload event handler of the plugin. | |
virtual const char * | _getErrorMsg () |
Call pcmGetErrorMsg function of the plugin. | |
virtual void | _checkError () |
Check the error code of the last operation. | |
Public Types | |
typedef ParCompMark::Pointer< Plugin, Mutex > | Pointer |
Type for pointer on this class. | |
BASIC_PLUGIN | |
Type for Plugin class. | |
RENDERER_PLUGIN | |
Type for Renderer class. | |
enum | PluginType { BASIC_PLUGIN, RENDERER_PLUGIN } |
Definitions of plugin types. More... | |
Public Member Functions | |
Constructors & destructor | |
Plugin (const PluginType &type, const std::string &name, const std::string &filename) | |
Create a plugin. | |
virtual | ~Plugin () |
The destructor. | |
Getters & setters | |
const Plugin::PluginType & | getPluginType () const |
Getter of mPluginType. | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
const std::list< std::string > & | getNeededLibs () const |
Getter of mNeededLibs. | |
const s32 & | getLastError () const |
Getter of mLastError. | |
Protected Types | |
typedef const char **(*) | pcmGetNeededLibsType () |
Function pointer type for. | |
typedef void(*) | loggerFunctionType (const void *, const char *) |
Function pointer type for the argument of. | |
typedef int(*) | pcmSetPluginHandleType (void *) |
Function pointer type for. | |
typedef int(*) | pcmSetLoggerFunctionType (loggerFunctionType) |
Function pointer type for. | |
typedef int(*) | pcmOnLoadType () |
Function pointer type for. | |
typedef int(*) | pcmOnUnloadType () |
Function pointer type for. | |
typedef const char *(*) | pcmGetErrorMsgType (const int) |
Function pointer type for. | |
Static Protected Member Functions | |
Class methods | |
static void | _loggerFunction (const void *handle, const char *message) |
Logger function with simple C style interface can be called by the plugin. | |
Protected Attributes | |
Variables | |
PluginType | mPluginType |
Plugin type. | |
bool | mInitialized |
The plugin is initialized. | |
std::list< std::string > | mNeededLibs |
Needed dynamic libraries. | |
s32 | mLastError |
Error code of the last error occured by the plugin code. |
Member Typedef Documentation
typedef void(*) ParCompMark::Plugin::loggerFunctionType(const void *, const char *) [protected] |
typedef const char*(*) ParCompMark::Plugin::pcmGetErrorMsgType(const int) [protected] |
typedef const char**(*) ParCompMark::Plugin::pcmGetNeededLibsType() [protected] |
typedef int(*) ParCompMark::Plugin::pcmOnLoadType() [protected] |
typedef int(*) ParCompMark::Plugin::pcmOnUnloadType() [protected] |
typedef int(*) ParCompMark::Plugin::pcmSetLoggerFunctionType(loggerFunctionType) [protected] |
typedef int(*) ParCompMark::Plugin::pcmSetPluginHandleType(void *) [protected] |
typedef ParCompMark::Pointer< Plugin, Mutex > ParCompMark::Plugin::Pointer |
Type for pointer on this class.
Reimplemented from ParCompMark::DynLoad.
Reimplemented in ParCompMark::RendererPlugin.
Definition at line 96 of file PCMPlugin.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
ParCompMark::Plugin::Plugin | ( | const PluginType & | type, | |
const std::string & | name, | |||
const std::string & | filename | |||
) |
Create a plugin.
- Parameters:
-
[in] type Type of the plugin. [in] name Name of the plugin. [in] filename Plugin file name.
Definition at line 38 of file PCMPlugin.cpp.
References _getNeededLibs(), _setLoggerFunction(), _setPluginHandle(), ParCompMark::Singleton< T >::getInstance(), mInitialized, mLastError, ParCompMark::DynLoad::mLibraryName, ParCompMark::Name::mName, mPluginType, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::Plugin::~Plugin | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 65 of file PCMPlugin.cpp.
References finalize(), ParCompMark::Singleton< T >::getInstance(), mInitialized, ParCompMark::DynLoad::mLibraryName, ParCompMark::Name::mName, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::Plugin::_checkError | ( | ) | [inline, protected, virtual] |
Check the error code of the last operation.
Definition at line 379 of file PCMPlugin.h.
Referenced by _onLoad(), _onUnload(), ParCompMark::RendererPlugin::_setBufferGetter(), _setLoggerFunction(), _setPluginHandle(), and ParCompMark::RendererPlugin::destroyRenderer().
virtual void ParCompMark::Plugin::_finalizeSpecific | ( | ) | [protected, pure virtual] |
const char * ParCompMark::Plugin::_getErrorMsg | ( | ) | [protected, virtual] |
Call pcmGetErrorMsg function of the plugin.
- Returns:
- Description of the last error.
Definition at line 207 of file PCMPlugin.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::DynLoad::getFunction(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::DynLoad::hasFunction(), mLastError, and ParCompMark::Name::mName.
Here is the call graph for this function:
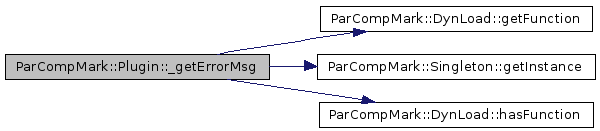
void ParCompMark::Plugin::_getNeededLibs | ( | ) | [protected, virtual] |
Get needed libraries from the plugin.
Definition at line 125 of file PCMPlugin.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::DynLoad::getFunction(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::DynLoad::hasFunction(), ParCompMark::Name::mName, and mNeededLibs.
Referenced by Plugin().
Here is the call graph for this function:

virtual void ParCompMark::Plugin::_initializeSpecific | ( | ) | [protected, pure virtual] |
Specific initialization code.
Implemented in ParCompMark::RendererPlugin.
Referenced by initialize().
void ParCompMark::Plugin::_loggerFunction | ( | const void * | handle, | |
const char * | message | |||
) | [static, protected] |
Logger function with simple C style interface can be called by the plugin.
- Parameters:
-
[in] handle Plugin handle [in] message Message to log.
Definition at line 80 of file PCMPlugin.cpp.
References ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), and ParCompMark::Logger::NOTICE.
Referenced by _setLoggerFunction().
Here is the call graph for this function:

void ParCompMark::Plugin::_onLoad | ( | ) | [protected, virtual] |
Call pcmOnLoad event handler of the plugin.
Definition at line 175 of file PCMPlugin.cpp.
References _checkError(), ParCompMark::Logger::DEBUG, ParCompMark::DynLoad::getFunction(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::DynLoad::hasFunction(), mLastError, and ParCompMark::Name::mName.
Referenced by initialize().
Here is the call graph for this function:
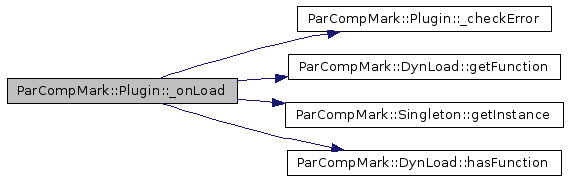
void ParCompMark::Plugin::_onUnload | ( | ) | [protected, virtual] |
Call pcmOnUnload event handler of the plugin.
Definition at line 191 of file PCMPlugin.cpp.
References _checkError(), ParCompMark::Logger::DEBUG, ParCompMark::DynLoad::getFunction(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::DynLoad::hasFunction(), mLastError, and ParCompMark::Name::mName.
Referenced by finalize().
Here is the call graph for this function:

void ParCompMark::Plugin::_setLoggerFunction | ( | ) | [protected, virtual] |
Set logger function to the plugin.
Definition at line 159 of file PCMPlugin.cpp.
References _checkError(), _loggerFunction(), ParCompMark::Logger::DEBUG, ParCompMark::DynLoad::getFunction(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::DynLoad::hasFunction(), mLastError, and ParCompMark::Name::mName.
Referenced by Plugin().
Here is the call graph for this function:
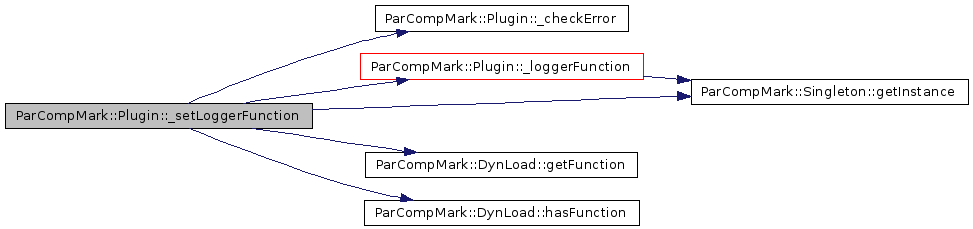
void ParCompMark::Plugin::_setPluginHandle | ( | ) | [protected, virtual] |
Set plugin handle to the plugin.
Definition at line 143 of file PCMPlugin.cpp.
References _checkError(), ParCompMark::Logger::DEBUG, ParCompMark::DynLoad::getFunction(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::DynLoad::hasFunction(), mLastError, and ParCompMark::Name::mName.
Referenced by Plugin().
Here is the call graph for this function:

void ParCompMark::Plugin::finalize | ( | ) | [virtual] |
Finalize the plugin.
Definition at line 108 of file PCMPlugin.cpp.
References _onUnload(), Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), mInitialized, and ParCompMark::Name::mName.
Referenced by ~Plugin().
Here is the call graph for this function:

const bool & ParCompMark::Plugin::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 354 of file PCMPlugin.h.
const s32 & ParCompMark::Plugin::getLastError | ( | ) | const [inline] |
Getter of mLastError.
Returns value of mLastError.
- Returns:
- The value of mLastError
Definition at line 368 of file PCMPlugin.h.
const std::list< std::string > & ParCompMark::Plugin::getNeededLibs | ( | ) | const [inline] |
Getter of mNeededLibs.
Returns value of mNeededLibs.
- Returns:
- The value of mNeededLibs
Definition at line 361 of file PCMPlugin.h.
const Plugin::PluginType & ParCompMark::Plugin::getPluginType | ( | ) | const [inline] |
Getter of mPluginType.
Returns value of mPluginType.
- Returns:
- The value of mPluginType
Definition at line 347 of file PCMPlugin.h.
void ParCompMark::Plugin::initialize | ( | ) | [virtual] |
Initialize the plugin.
Definition at line 93 of file PCMPlugin.cpp.
References _initializeSpecific(), _onLoad(), Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), mInitialized, and ParCompMark::Name::mName.
Here is the call graph for this function:

Member Data Documentation
bool ParCompMark::Plugin::mInitialized [protected] |
The plugin is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 142 of file PCMPlugin.h.
Referenced by finalize(), initialize(), Plugin(), and ~Plugin().
s32 ParCompMark::Plugin::mLastError [protected] |
Error code of the last error occured by the plugin code.
0 must mean no error.
- Remarks:
- This is own attribute of this class.
Definition at line 156 of file PCMPlugin.h.
Referenced by _getErrorMsg(), _onLoad(), _onUnload(), ParCompMark::RendererPlugin::_setBufferGetter(), _setLoggerFunction(), _setPluginHandle(), ParCompMark::RendererPlugin::destroyRenderer(), and Plugin().
std::list< std::string > ParCompMark::Plugin::mNeededLibs [protected] |
Needed dynamic libraries.
- Remarks:
- This is own attribute of this class.
Definition at line 149 of file PCMPlugin.h.
Referenced by _getNeededLibs().
PluginType ParCompMark::Plugin::mPluginType [protected] |
Plugin type.
- Remarks:
- This is own attribute of this class.
Definition at line 135 of file PCMPlugin.h.
Referenced by Plugin().
The documentation for this class was generated from the following files: