ParCompMark::Renderer Class Reference
#include <PCMRenderer.h>
Collaboration diagram for ParCompMark::Renderer:
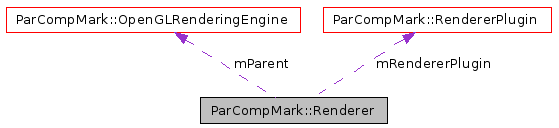
Detailed Description
Custom renderer object belongs to a renderer plugin.
Definition at line 65 of file PCMRenderer.h.
Public Types | |
typedef ParCompMark::Pointer< Renderer, Mutex > | Pointer |
Type for pointer on this class. | |
Public Member Functions | |
Constructors & destructor | |
Renderer () | |
Default constructor for Squirrel compatibility. | |
Renderer (RendererPlugin *rendererPlugin, void *rendererHandle, OpenGLRenderingEngine *parent) | |
Create a custom renderer. | |
virtual | ~Renderer () |
The destructor. | |
Getters & setters | |
void * | getRendererHandle () const |
Getter of mRendererHandle. | |
void | setRendererHandle (const void *rendererhandle) |
Setter of mRendererHandle. | |
OpenGLRenderingEngine * | getParent () const |
Getter of mParent. | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
s32 & | getAutoRenderOrder () |
Getter of mAutoRenderOrder. | |
void | setAutoRenderOrder (const s32 autorenderorder) |
Setter of mAutoRenderOrder. | |
Methods | |
virtual void | initialize () |
Initialize the renderer. | |
virtual void | finalize () |
Finalize the renderer. | |
virtual void | resize (const u32 width, const u32 height) |
Call the pcmOnResize event handler of the plugin. | |
virtual s32 | getSortOrder () |
Call the pcmSetSortOrder function of the plugin. | |
virtual void | render () |
Call the pcmOnRender event handler of the plugin. | |
virtual void | setMiscParam (const char *name, const char *value) |
Call the pcmSetMiscParam function of the plugin. | |
virtual void | setObjectSpaceBoundingBox (const double x0, const double y0, const double z0, const double x1, const double y1, const double z1) |
Call the pcmSetObjectSpaceBoundingBox function of the plugin. | |
virtual void | setObjectId (const unsigned objectId) |
Call the pcmSetObjectId function of the plugin. | |
virtual void | setScreenSpaceFramelet (const double u0, const double v0, const double u1, const double v1) |
Call the pcmSetScreenSpaceFramelet function of the plugin. | |
virtual void | registerObjectSpaceBoundingBox (const double x0, const double y0, const double z0, const double x1, const double y1, const double z1) |
Call the pcmRegisterObjectSpaceBoundingBox function of the plugin. | |
Static Public Member Functions | |
Scripting binding | |
static void | squirrelGlue () |
Static glue code method for Squirrel-C++ binding. | |
Static Public Attributes | |
Class constants | |
static const s32 | NOAUTORENDERING = -1 |
Constant for indication, that the renderer does not render automatically. | |
Protected Attributes | |
Variables | |
void * | mRendererHandle |
Pointer to the renderer instance in the plugin code. | |
RendererPlugin * | mRendererPlugin |
Corresponding renderer plugin. | |
OpenGLRenderingEngine * | mParent |
Parent rendering engine of the renderer. | |
bool | mInitialized |
The renderer is initialized. | |
s32 | mAutoRenderOrder |
If this attribute not equals Renderer::NOAUTORENDERING, it is the order in the automatic rendering queue on the correspondent rendering engine. |
Member Typedef Documentation
Constructor & Destructor Documentation
ParCompMark::Renderer::Renderer | ( | ) |
Default constructor for Squirrel compatibility.
Calling this constructor always raises an exception.
Definition at line 44 of file PCMRenderer.cpp.
References Except.
ParCompMark::Renderer::Renderer | ( | RendererPlugin * | rendererPlugin, | |
void * | rendererHandle, | |||
OpenGLRenderingEngine * | parent | |||
) |
Create a custom renderer.
- Parameters:
-
[in] rendererPlugin Corresponding renderer plugin. [in] rendererHandle Pointer to the renderer instance in the plugin code. [in] parent Parent rendering engine of the renderer.
Definition at line 58 of file PCMRenderer.cpp.
References ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mAutoRenderOrder, mInitialized, mRendererPlugin, NOAUTORENDERING, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::Renderer::~Renderer | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 85 of file PCMRenderer.cpp.
References finalize(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mInitialized, mRendererPlugin, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::Renderer::finalize | ( | ) | [virtual] |
Finalize the renderer.
Definition at line 113 of file PCMRenderer.cpp.
References Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mInitialized, and mRendererPlugin.
Referenced by ~Renderer().
Here is the call graph for this function:

s32 & ParCompMark::Renderer::getAutoRenderOrder | ( | ) | [inline] |
Getter of mAutoRenderOrder.
Returns value of mAutoRenderOrder.
- Returns:
- The value of mAutoRenderOrder
Definition at line 413 of file PCMRenderer.h.
Referenced by squirrelGlue().
const bool & ParCompMark::Renderer::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 406 of file PCMRenderer.h.
OpenGLRenderingEngine * ParCompMark::Renderer::getParent | ( | ) | const [inline] |
Getter of mParent.
Returns value of mParent.
- Returns:
- The value of mParent
Definition at line 399 of file PCMRenderer.h.
Referenced by ParCompMark::RendererPlugin::_bufferGetter().
void * ParCompMark::Renderer::getRendererHandle | ( | ) | const [inline] |
Getter of mRendererHandle.
Returns value of mRendererHandle.
- Returns:
- The value of mRendererHandle
Definition at line 385 of file PCMRenderer.h.
Referenced by ParCompMark::RendererPlugin::destroyRenderer().
s32 ParCompMark::Renderer::getSortOrder | ( | ) | [inline, virtual] |
Call the pcmSetSortOrder
function of the plugin.
- Returns:
- Sort order. -1 value indicates that the plugin has no sort order calculation routine or the sort order is unknown.
Definition at line 438 of file PCMRenderer.h.
void ParCompMark::Renderer::initialize | ( | ) | [virtual] |
Initialize the renderer.
Definition at line 101 of file PCMRenderer.cpp.
References Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mInitialized, and mRendererPlugin.
Here is the call graph for this function:

void ParCompMark::Renderer::registerObjectSpaceBoundingBox | ( | const double | x0, | |
const double | y0, | |||
const double | z0, | |||
const double | x1, | |||
const double | y1, | |||
const double | z1 | |||
) | [inline, virtual] |
Call the pcmRegisterObjectSpaceBoundingBox
function of the plugin.
- Parameters:
-
[in] x0 Lowest corner of the bounding box. [in] y0 Lowest corner of the bounding box. [in] z0 Lowest corner of the bounding box. [in] x1 Highest corner of the bounding box. [in] y1 Highest corner of the bounding box. [in] z1 Highest corner of the bounding box.
Definition at line 483 of file PCMRenderer.h.
Referenced by squirrelGlue().
void ParCompMark::Renderer::render | ( | ) | [inline, virtual] |
Call the pcmOnRender
event handler of the plugin.
Definition at line 445 of file PCMRenderer.h.
Referenced by squirrelGlue().
Call the pcmOnResize
event handler of the plugin.
- Parameters:
-
[in] width New width of the window on which the renderer renders. [in] height New height of the window on which the renderer renders.
Definition at line 431 of file PCMRenderer.h.
void ParCompMark::Renderer::setAutoRenderOrder | ( | const s32 | autorenderorder | ) | [inline] |
Setter of mAutoRenderOrder.
Sets value of mAutoRenderOrder.
- Parameters:
-
[in] autorenderorder The value of mAutoRenderOrder
Definition at line 420 of file PCMRenderer.h.
Referenced by squirrelGlue().
void ParCompMark::Renderer::setMiscParam | ( | const char * | name, | |
const char * | value | |||
) | [inline, virtual] |
Call the pcmSetMiscParam
function of the plugin.
- Parameters:
-
[in] name Name of the parameter. [in] value Value of the parameter.
Definition at line 453 of file PCMRenderer.h.
Referenced by squirrelGlue().
void ParCompMark::Renderer::setObjectId | ( | const unsigned | objectId | ) | [inline, virtual] |
Call the pcmSetObjectId
function of the plugin.
- Parameters:
-
[in] objectId Render object with the specified id.
Definition at line 468 of file PCMRenderer.h.
Referenced by squirrelGlue().
void ParCompMark::Renderer::setObjectSpaceBoundingBox | ( | const double | x0, | |
const double | y0, | |||
const double | z0, | |||
const double | x1, | |||
const double | y1, | |||
const double | z1 | |||
) | [inline, virtual] |
Call the pcmSetObjectSpaceBoundingBox
function of the plugin.
- Parameters:
-
[in] x0 Lowest corner of the bounding box. [in] y0 Lowest corner of the bounding box. [in] z0 Lowest corner of the bounding box. [in] x1 Highest corner of the bounding box. [in] y1 Highest corner of the bounding box. [in] z1 Highest corner of the bounding box.
Definition at line 460 of file PCMRenderer.h.
Referenced by squirrelGlue().
void ParCompMark::Renderer::setRendererHandle | ( | const void * | rendererhandle | ) | [inline] |
Setter of mRendererHandle.
Sets value of mRendererHandle.
- Parameters:
-
[in] rendererhandle The value of mRendererHandle
Definition at line 392 of file PCMRenderer.h.
void ParCompMark::Renderer::setScreenSpaceFramelet | ( | const double | u0, | |
const double | v0, | |||
const double | u1, | |||
const double | v1 | |||
) | [inline, virtual] |
Call the pcmSetScreenSpaceFramelet
function of the plugin.
- Parameters:
-
[in] u0 Top-left corner of the framelet. [in] v0 Top-left corner of the framelet. [in] u1 Bottom-right corner of the framelet. [in] v1 Bottom-right corner of the framelet.
Definition at line 475 of file PCMRenderer.h.
Referenced by squirrelGlue().
static void ParCompMark::Renderer::squirrelGlue | ( | ) | [inline, static] |
Static glue code method for Squirrel-C++ binding.
This method should be call from each Squirrel virtual machines to generate the proper Squirrel glue code. To content of this method is fully autogenerated in the header implementation file, you should not modify it.
Definition at line 89 of file PCMRenderer.h.
References getAutoRenderOrder(), registerObjectSpaceBoundingBox(), render(), setAutoRenderOrder(), setMiscParam(), setObjectId(), setObjectSpaceBoundingBox(), and setScreenSpaceFramelet().
Referenced by ParCompMark::squirrelClassBindings().
Here is the call graph for this function:

Member Data Documentation
s32 ParCompMark::Renderer::mAutoRenderOrder [protected] |
If this attribute not equals Renderer::NOAUTORENDERING, it is the order in the automatic rendering queue on the correspondent rendering engine.
- Remarks:
- This is own attribute of this class.
Definition at line 175 of file PCMRenderer.h.
Referenced by Renderer().
bool ParCompMark::Renderer::mInitialized [protected] |
The renderer is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 168 of file PCMRenderer.h.
Referenced by finalize(), initialize(), Renderer(), and ~Renderer().
OpenGLRenderingEngine* ParCompMark::Renderer::mParent [protected] |
Parent rendering engine of the renderer.
- Remarks:
- This attribute references an attribute.
Definition at line 161 of file PCMRenderer.h.
void* ParCompMark::Renderer::mRendererHandle [protected] |
Pointer to the renderer instance in the plugin code.
- Remarks:
- This attribute references an attribute.
Definition at line 147 of file PCMRenderer.h.
RendererPlugin* ParCompMark::Renderer::mRendererPlugin [protected] |
Corresponding renderer plugin.
- Remarks:
- This attribute references an attribute.
Definition at line 154 of file PCMRenderer.h.
Referenced by finalize(), initialize(), Renderer(), and ~Renderer().
const s32 ParCompMark::Renderer::NOAUTORENDERING = -1 [static] |
Constant for indication, that the renderer does not render automatically.
- Remarks:
- This is own attribute of this class.
Definition at line 129 of file PCMRenderer.h.
Referenced by Renderer(), and ParCompMark::OpenGLRenderingEngine::setAutoRenderOrder().
The documentation for this class was generated from the following files: