ParCompMark::ConfigOptions Class Reference
#include <PCMConfigOptions.h>
Collaboration diagram for ParCompMark::ConfigOptions:
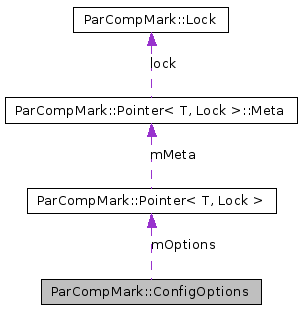
Detailed Description
Program configuration options.Stored in application ini file.
Definition at line 60 of file PCMConfigOptions.h.
Methods | |
virtual void | initialize () |
Initialize option container. | |
virtual void | finalize () |
Finalize option container. | |
virtual bool | hasOption (const std::string &name) |
Check for option. | |
virtual std::string | getString (const std::string &name) |
Get option. | |
virtual bool | getBool (const std::string &name) |
Get option. | |
virtual s32 | getInteger (const std::string &name) |
Get option. | |
virtual Real | getReal (const std::string &name) |
Get option. | |
virtual bool | testString (const std::string &name, const std::string &value) |
Return true if the option exists with the given name and equals to the given value. | |
virtual void | setString (const std::string &name, const std::string &value, const std::string &description="") |
Set option. | |
virtual void | setBool (const std::string &name, const bool &value, const std::string &description="") |
Set option. | |
virtual void | setInteger (const std::string &name, const s32 &value, const std::string &description="") |
Set option. | |
virtual void | setReal (const std::string &name, const Real &value, const std::string &description="") |
Set option. | |
virtual void | removeOption (const std::string &name) |
Remove option. | |
virtual void | saveToIniFile (const std::string &filename) |
Create user-level ini file in the application directory (existing file will be overwritten). | |
virtual void | loadFromIniFile (const std::string &filename) |
Load user-level ini file. | |
virtual ConfigOptions::Option::Pointer | getValue (const std::string &name, const ConfigOptions::OptionType &type) |
Get option value for the specified type (internal method). | |
virtual void | setValue (const std::string &name, const void *value, const ConfigOptions::OptionType &type, const std::string &description) |
Set option value for the specified type (internal method). | |
virtual u32 | getOptionTypeSize (const ConfigOptions::OptionType &type) |
Get size of option type. | |
virtual std::string | optionToString (ConfigOptions::Option::Pointer &option) |
Give string representation of the option value. | |
Public Types | |
typedef Pointer< ConfigOptions, Mutex > | Pointer |
Type for pointer on this class. | |
Public Member Functions | |
Constructors & destructor | |
ConfigOptions () | |
Default constructor. | |
virtual | ~ConfigOptions () |
The destructor. | |
Getters & setters | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
Protected Types | |
typedef ParCompMark::ConfigOptions::Option | Option |
Struct for option. | |
STRING | |
STL string type. | |
BOOL | |
Bool type. | |
INTEGER | |
s32 integer type | |
REAL | |
Real type. | |
enum | OptionType { STRING, BOOL, INTEGER, REAL } |
Option type definitions. More... | |
Protected Attributes | |
Variables | |
Container< ConfigOptions::Option, Mutex >::Pointer | mOptions |
Container for application options. | |
bool | mInitialized |
The option container is initialized. | |
Classes | |
struct | Option |
Struct for option. More... |
Member Typedef Documentation
typedef struct ParCompMark::ConfigOptions::Option
ParCompMark::ConfigOptions::Option [protected] |
Struct for option.
typedef Pointer< ConfigOptions, Mutex > ParCompMark::ConfigOptions::Pointer |
Member Enumeration Documentation
enum ParCompMark::ConfigOptions::OptionType [protected] |
Constructor & Destructor Documentation
ParCompMark::ConfigOptions::ConfigOptions | ( | ) |
Default constructor.
Definition at line 51 of file PCMConfigOptions.cpp.
References ParCompMark::Singleton< T >::getInstance(), mInitialized, mOptions, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::ConfigOptions::~ConfigOptions | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 64 of file PCMConfigOptions.cpp.
References finalize(), ParCompMark::Singleton< T >::getInstance(), mInitialized, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::ConfigOptions::finalize | ( | ) | [virtual] |
Finalize option container.
Definition at line 88 of file PCMConfigOptions.cpp.
References Assert, ParCompMark::Singleton< T >::getInstance(), mInitialized, and ParCompMark::Logger::NOTICE.
Referenced by ~ConfigOptions().
Here is the call graph for this function:

bool ParCompMark::ConfigOptions::getBool | ( | const std::string & | name | ) | [virtual] |
Get option.
- Parameters:
-
[in] name Name of the option
- Returns:
- Bool value
Definition at line 116 of file PCMConfigOptions.cpp.
References BOOL, and getValue().
Here is the call graph for this function:

const bool & ParCompMark::ConfigOptions::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 382 of file PCMConfigOptions.h.
s32 ParCompMark::ConfigOptions::getInteger | ( | const std::string & | name | ) | [virtual] |
Get option.
- Parameters:
-
[in] name Name of the option
- Returns:
- Integer value
Definition at line 123 of file PCMConfigOptions.cpp.
References getValue(), and INTEGER.
Here is the call graph for this function:

u32 ParCompMark::ConfigOptions::getOptionTypeSize | ( | const ConfigOptions::OptionType & | type | ) | [protected, virtual] |
Get size of option type.
- Parameters:
-
[in] type Type of the option
- Returns:
- Size of the specified type
Definition at line 339 of file PCMConfigOptions.cpp.
References BOOL, INTEGER, REAL, and STRING.
Referenced by setValue().
Real ParCompMark::ConfigOptions::getReal | ( | const std::string & | name | ) | [virtual] |
Get option.
- Parameters:
-
[in] name Name of the option
- Returns:
- Real value
Definition at line 130 of file PCMConfigOptions.cpp.
References getValue(), and REAL.
Here is the call graph for this function:

std::string ParCompMark::ConfigOptions::getString | ( | const std::string & | name | ) | [virtual] |
Get option.
- Parameters:
-
[in] name Name of the option
- Returns:
- STL string value
Definition at line 109 of file PCMConfigOptions.cpp.
References getValue(), and STRING.
Referenced by testString().
Here is the call graph for this function:

ConfigOptions::Option::Pointer ParCompMark::ConfigOptions::getValue | ( | const std::string & | name, | |
const ConfigOptions::OptionType & | type | |||
) | [protected, virtual] |
Get option value for the specified type (internal method).
- Parameters:
-
[in] name Name of the option [in] type Type of the option
- Returns:
- Pointer to the specified option
Definition at line 281 of file PCMConfigOptions.cpp.
References Assert, Except, hasOption(), ParCompMark::Pointer< T, Lock >::lock(), mOptions, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by getBool(), getInteger(), getReal(), and getString().
Here is the call graph for this function:

bool ParCompMark::ConfigOptions::hasOption | ( | const std::string & | name | ) | [virtual] |
Check for option.
- Parameters:
-
[in] name Name of the option
- Returns:
- True if the container has the specified option.
Definition at line 98 of file PCMConfigOptions.cpp.
References ParCompMark::Pointer< T, Lock >::lock(), mOptions, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by getValue(), removeOption(), saveToIniFile(), and testString().
Here is the call graph for this function:

void ParCompMark::ConfigOptions::initialize | ( | ) | [virtual] |
Initialize option container.
Definition at line 78 of file PCMConfigOptions.cpp.
References Assert, ParCompMark::Singleton< T >::getInstance(), mInitialized, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

void ParCompMark::ConfigOptions::loadFromIniFile | ( | const std::string & | filename | ) | [virtual] |
Load user-level ini file.
- Parameters:
-
[in] filename Name of the config file
Definition at line 235 of file PCMConfigOptions.cpp.
References ParCompMark::FileSystemManager::closeFile(), Except, ParCompMark::Singleton< T >::getInstance(), ParCompMark::FileSystemManager::getInstance(), ParCompMark::Logger::NOTICE, ParCompMark::FileSystemManager::READ, setString(), ParCompMark::StringConverter::toString(), and ParCompMark::StringConverter::trim().
Here is the call graph for this function:

std::string ParCompMark::ConfigOptions::optionToString | ( | ConfigOptions::Option::Pointer & | option | ) | [protected, virtual] |
Give string representation of the option value.
- Parameters:
-
[out] option Option
- Returns:
- String representation
Definition at line 359 of file PCMConfigOptions.cpp.
References BOOL, INTEGER, REAL, STRING, and ParCompMark::StringConverter::toString().
Referenced by saveToIniFile().
Here is the call graph for this function:

void ParCompMark::ConfigOptions::removeOption | ( | const std::string & | name | ) | [virtual] |
Remove option.
- Parameters:
-
[in] name Name of the option
Definition at line 183 of file PCMConfigOptions.cpp.
References Assert, hasOption(), ParCompMark::Pointer< T, Lock >::lock(), mOptions, and ParCompMark::Pointer< T, Lock >::unlock().
Here is the call graph for this function:

void ParCompMark::ConfigOptions::saveToIniFile | ( | const std::string & | filename | ) | [virtual] |
Create user-level ini file in the application directory (existing file will be overwritten).
- Parameters:
-
[in] filename Name of the config file
Definition at line 194 of file PCMConfigOptions.cpp.
References ParCompMark::FileSystemManager::closeFile(), ParCompMark::FileSystemManager::getAppDirectory(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::FileSystemManager::getInstance(), ParCompMark::Pointer< T, Lock >::getPtr(), ParCompMark::Timer::getTimeDateString(), hasOption(), mOptions, ParCompMark::Logger::NOTICE, optionToString(), and ParCompMark::FileSystemManager::WRITE.
Here is the call graph for this function:
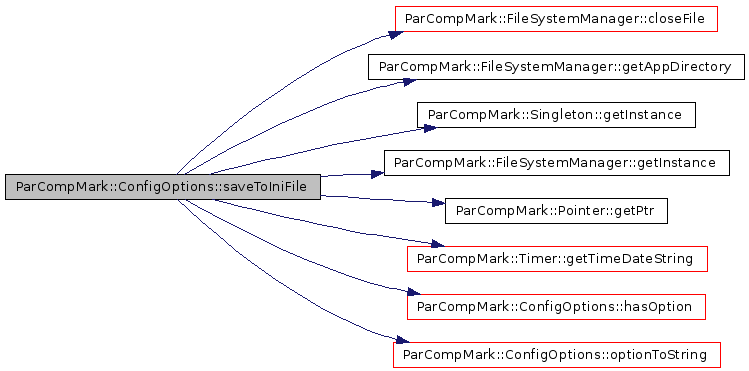
void ParCompMark::ConfigOptions::setBool | ( | const std::string & | name, | |
const bool & | value, | |||
const std::string & | description = "" | |||
) | [virtual] |
Set option.
- Parameters:
-
[in] name Name of the option [in] value Value of the option [in] description Description of the option
Definition at line 153 of file PCMConfigOptions.cpp.
References BOOL, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), setValue(), and ParCompMark::StringConverter::toString().
Here is the call graph for this function:
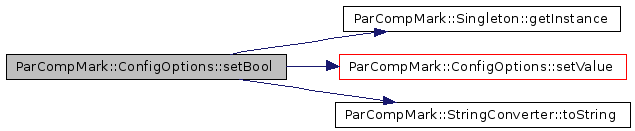
void ParCompMark::ConfigOptions::setInteger | ( | const std::string & | name, | |
const s32 & | value, | |||
const std::string & | description = "" | |||
) | [virtual] |
Set option.
- Parameters:
-
[in] name Name of the option [in] value Value of the option [in] description Description of the option
Definition at line 163 of file PCMConfigOptions.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), INTEGER, setValue(), and ParCompMark::StringConverter::toString().
Here is the call graph for this function:

void ParCompMark::ConfigOptions::setReal | ( | const std::string & | name, | |
const Real & | value, | |||
const std::string & | description = "" | |||
) | [virtual] |
Set option.
- Parameters:
-
[in] name Name of the option [in] value Value of the option [in] description Description of the option
Definition at line 173 of file PCMConfigOptions.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), REAL, setValue(), and ParCompMark::StringConverter::toString().
Here is the call graph for this function:
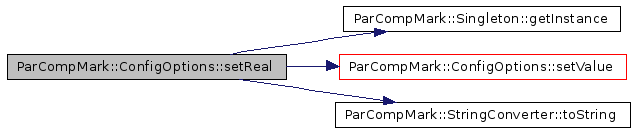
void ParCompMark::ConfigOptions::setString | ( | const std::string & | name, | |
const std::string & | value, | |||
const std::string & | description = "" | |||
) | [virtual] |
Set option.
- Parameters:
-
[in] name Name of the option [in] value Value of the option [in] description Description of the option
Definition at line 144 of file PCMConfigOptions.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), setValue(), and STRING.
Referenced by loadFromIniFile().
Here is the call graph for this function:

void ParCompMark::ConfigOptions::setValue | ( | const std::string & | name, | |
const void * | value, | |||
const ConfigOptions::OptionType & | type, | |||
const std::string & | description | |||
) | [protected, virtual] |
Set option value for the specified type (internal method).
- Parameters:
-
[in] name Name of the option [in] value Address of value [in] type Type of the option [in] description Description of the option
Definition at line 300 of file PCMConfigOptions.cpp.
References getOptionTypeSize(), ParCompMark::Pointer< T, Lock >::lock(), mOptions, STRING, and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by setBool(), setInteger(), setReal(), and setString().
Here is the call graph for this function:

bool ParCompMark::ConfigOptions::testString | ( | const std::string & | name, | |
const std::string & | value | |||
) | [virtual] |
Return true if the option exists with the given name and equals to the given value.
- Parameters:
-
[in] name Name of the option [in] value Value to test
- Returns:
- True if the option exists with the given name and equals to the given value
Definition at line 137 of file PCMConfigOptions.cpp.
References getString(), and hasOption().
Here is the call graph for this function:

Member Data Documentation
bool ParCompMark::ConfigOptions::mInitialized [protected] |
The option container is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 149 of file PCMConfigOptions.h.
Referenced by ConfigOptions(), finalize(), initialize(), and ~ConfigOptions().
Container< ConfigOptions::Option, Mutex >::Pointer ParCompMark::ConfigOptions::mOptions [protected] |
Container for application options.
- Remarks:
- This is own attribute of this class.
Definition at line 142 of file PCMConfigOptions.h.
Referenced by ConfigOptions(), getValue(), hasOption(), removeOption(), saveToIniFile(), and setValue().
The documentation for this class was generated from the following files: