ParCompMark::FileSystemManager Class Reference
#include <PCMFileSystemManager.h>
Inheritance diagram for ParCompMark::FileSystemManager:
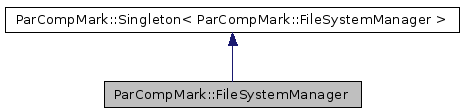
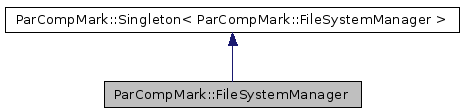
Detailed Description
Singleton class managing file system.This class handles file opening, config file handling.
Definition at line 76 of file PCMFileSystemManager.h.
Methods | |
virtual void | initialize () |
Initialize the file system manager. | |
virtual void | finalize () |
Finalize the file system manager. | |
virtual bool | existsDirectory (const std::string &name) const |
Return true if the specified directory exists. | |
virtual bool | createDirectory (const std::string &name) const |
Create directory. | |
virtual std::string | findDataDirectory () const |
Find data directory path. | |
virtual bool | existsLibrary (const std::string &library, const std::vector< std::string > &additionalPaths=std::vector< std::string >()) const |
Returns true if the library can be found. | |
virtual std::string | findLibraryPath (const std::string &library, const std::vector< std::string > &additionalPaths=std::vector< std::string >()) const |
Find path for library. | |
virtual std::list< std::string > | listDirectory (const std::string &directory) const |
List files in the specified directory. | |
virtual std::list< std::string > | listDataDirectory (const std::string &directory) const |
List files in the specified directory relative to the data directory. | |
virtual bool | existsFile (const std::string &name) const |
Return true if the specified file exists. | |
virtual bool | existsAppFile (const std::string &name) const |
Return true if the specified file exists in the application directory. | |
virtual bool | existsDataFile (const char *name) const |
Return true if the specified file exists in the data directory. | |
virtual FileSystemManager::CFilePointer | openFileC (const std::string &name, const FileOperation &operation=FileSystemManager::READ) const |
Open file for reading or writing and return a C-style file pointer. | |
virtual FileSystemManager::CFilePointer | openAppFileC (const std::string &name, const FileOperation &operation=FileSystemManager::READ) const |
Open file for reading or writing in the application directory and return a C-style file pointer. | |
virtual FileSystemManager::CFilePointer | openDataFileC (const std::string &name, const FileOperation &operation=FileSystemManager::READ) const |
Open file for reading or writing in the application data directory and return a C-style file pointer. | |
virtual FileSystemManager::CppFilePointer | openFileCpp (const std::string &name, const FileOperation &operation=FileSystemManager::READ) const |
Open file for reading or writing and return a fstream object. | |
virtual FileSystemManager::CppFilePointer | openAppFileCpp (const std::string &name, const FileOperation &operation=FileSystemManager::READ) const |
Open file for reading or writing in the application directory and return a fstream object. | |
virtual FileSystemManager::CppFilePointer | openDataFileCpp (const std::string &name, const FileOperation &operation=FileSystemManager::READ) const |
Open file for reading or writing in the application data directory and return a fstream object. | |
virtual std::string | getPathDataFile (const std::string &name) const |
Get full path of the specified data file. | |
virtual std::string | readTextFile (const std::string &name) const |
Read contents of a text file. | |
virtual std::string | readAppTextFile (const std::string &name) const |
Read contents of a text file in the application directory. | |
virtual std::string | readDataTextFile (const std::string &name) const |
Read contents of a text file in the application data directory. | |
virtual void | _findHomeDirectory () |
Find the home directory of the user on the filesystem. | |
virtual std::string | _replaceHomeChar (const std::string &path) const |
Replace '~' char in the specified path string. | |
virtual std::string | _translateToAbsolutePath (const std::string &path) const |
Translate relative path to absolute (also replace '~' char in the specified path string). | |
virtual void | _createAppDirectory () |
Create user-level application directory. | |
Public Types | |
typedef Pointer< FILE, Mutex > | CFilePointer |
Type for pointer on a C-style file pointer. | |
typedef Pointer< std::fstream, Mutex > | CppFilePointer |
Type for pointer on a std::fstream object. | |
READ | |
Read. | |
WRITE | |
Write. | |
APPEND | |
Append. | |
enum | FileOperation { READ, WRITE, APPEND } |
Type of file operation. More... | |
Public Member Functions | |
Constructors & destructor | |
FileSystemManager (const std::string &appDirectory="~/.ParCompMark/", const std::string &iniFile="parcompmark.ini") | |
Creates file system manager. | |
virtual | ~FileSystemManager () |
The destructor. | |
Getters & setters | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
const std::string & | getAppDirectory () const |
Getter of mAppDirectory. | |
void | setAppDirectory (const std::string &appdirectory) |
Setter of mAppDirectory. | |
const std::string & | getDataDirectory () const |
Getter of mDataDirectory. | |
void | setDataDirectory (const std::string &datadirectory) |
Setter of mDataDirectory. | |
const std::string & | getHomeDirectory () const |
Getter of mHomeDirectory. | |
const std::string & | getCurrentDirectory () const |
Getter of mCurrentDirectory. | |
const std::string & | getIniFile () const |
Getter of mIniFile. | |
Static Public Member Functions | |
Scripting binding | |
static void | squirrelGlue () |
Static glue code method for Squirrel-C++ binding. | |
Class methods | |
static FileSystemManager * | getInstance () |
Gets the instance of the singleton class. | |
static void | closeFile (FileSystemManager::CppFilePointer &fp) |
Close file from fstream object. | |
static void | closeFile (FileSystemManager::CFilePointer &fp) |
Close file from C-style pointer. | |
Static Public Attributes | |
Class constants | |
static const u32 | MAX_PATH = 1024 |
Maximal number of chars in paths. | |
static const std::string | PATH_SEPARATOR = "/" |
Path separator on the host OS. | |
Protected Attributes | |
Variables | |
bool | mInitialized |
The application is initialized. | |
std::string | mAppDirectory |
Path of user-level application directory. | |
std::string | mDataDirectory |
Path of application data directory. | |
std::string | mHomeDirectory |
Home directory of the user. | |
std::string | mCurrentDirectory |
Path of current directory. | |
std::string | mIniFile |
Name of user-level ini file. |
Member Typedef Documentation
typedef Pointer< FILE, Mutex > ParCompMark::FileSystemManager::CFilePointer |
typedef Pointer< std::fstream, Mutex > ParCompMark::FileSystemManager::CppFilePointer |
Member Enumeration Documentation
Constructor & Destructor Documentation
ParCompMark::FileSystemManager::FileSystemManager | ( | const std::string & | appDirectory = "~/.ParCompMark/" , |
|
const std::string & | iniFile = "parcompmark.ini" | |||
) |
Creates file system manager.
- Parameters:
-
[in] appDirectory Path of application directory. [in] iniFile Name of user-level ini file.
Definition at line 63 of file PCMFileSystemManager.cpp.
References mAppDirectory, mCurrentDirectory, mDataDirectory, mHomeDirectory, mIniFile, and mInitialized.
ParCompMark::FileSystemManager::~FileSystemManager | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 84 of file PCMFileSystemManager.cpp.
References finalize(), ParCompMark::Singleton< T >::getInstance(), mInitialized, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::FileSystemManager::_createAppDirectory | ( | ) | [protected, virtual] |
Create user-level application directory.
Do nothing if already exists.
Definition at line 625 of file PCMFileSystemManager.cpp.
References createDirectory(), and mAppDirectory.
Referenced by initialize().
Here is the call graph for this function:

void ParCompMark::FileSystemManager::_findHomeDirectory | ( | ) | [protected, virtual] |
Find the home directory of the user on the filesystem.
Definition at line 526 of file PCMFileSystemManager.cpp.
References ParCompMark::Application::getEnvironmentVariable(), ParCompMark::Application::getInstance(), and mHomeDirectory.
Referenced by initialize().
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::_replaceHomeChar | ( | const std::string & | path | ) | const [protected, virtual] |
Replace '~' char in the specified path string.
- Parameters:
-
[in] path Path string.
- Returns:
- Replaced path string.
Definition at line 533 of file PCMFileSystemManager.cpp.
References mHomeDirectory, and ParCompMark::StringConverter::trim().
Referenced by _translateToAbsolutePath(), createDirectory(), existsDirectory(), existsFile(), and initialize().
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::_translateToAbsolutePath | ( | const std::string & | path | ) | const [protected, virtual] |
Translate relative path to absolute (also replace '~' char in the specified path string).
Original code: http://www.codeproject.com/useritems/path_conversion.asp
- Parameters:
-
[in] path Path string.
- Returns:
- Replaced path string.
Definition at line 551 of file PCMFileSystemManager.cpp.
References _replaceHomeChar(), MAX_PATH, mCurrentDirectory, and PATH_SEPARATOR.
Referenced by findDataDirectory(), and findLibraryPath().
Here is the call graph for this function:

void ParCompMark::FileSystemManager::closeFile | ( | FileSystemManager::CFilePointer & | fp | ) | [static] |
Close file from C-style pointer.
This method is static to be able to close the logfile.
- Parameters:
-
[out] fp Smart pointer on a C-style file pointer.
Definition at line 114 of file PCMFileSystemManager.cpp.
References ParCompMark::Pointer< T, Lock >::getPtr(), ParCompMark::Pointer< T, Lock >::lock(), ParCompMark::Pointer< T, Lock >::setNull(), and ParCompMark::Pointer< T, Lock >::unlock().
Here is the call graph for this function:
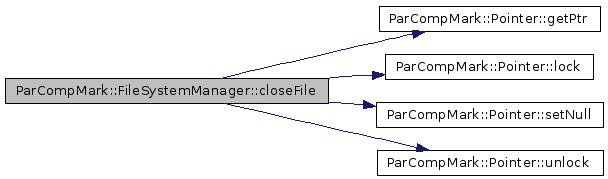
void ParCompMark::FileSystemManager::closeFile | ( | FileSystemManager::CppFilePointer & | fp | ) | [static] |
Close file from fstream object.
This method is static to be able to close the logfile.
- Parameters:
-
[out] fp Smart pointer on a fstream object.
Definition at line 105 of file PCMFileSystemManager.cpp.
References ParCompMark::Pointer< T, Lock >::lock(), and ParCompMark::Pointer< T, Lock >::unlock().
Referenced by ParCompMark::ConfigOptions::loadFromIniFile(), ParCompMark::PluginManager::loadPlugins_old(), readTextFile(), ParCompMark::HostInfo::refreshCPUs(), ParCompMark::ConfigOptions::saveToIniFile(), and ParCompMark::Logger::~Logger().
Here is the call graph for this function:

bool ParCompMark::FileSystemManager::createDirectory | ( | const std::string & | name | ) | const [virtual] |
Create directory.
- Parameters:
-
[in] name Name of the directory.
- Returns:
- True if the directory did not exist, and had to be created.
Definition at line 170 of file PCMFileSystemManager.cpp.
References _replaceHomeChar(), ParCompMark::Logger::DEBUG, errno, Except, existsDirectory(), ParCompMark::Singleton< T >::getInstance(), and ParCompMark::Logger::WARNING.
Referenced by _createAppDirectory().
Here is the call graph for this function:

bool ParCompMark::FileSystemManager::existsAppFile | ( | const std::string & | name | ) | const [virtual] |
Return true if the specified file exists in the application directory.
- Parameters:
-
[in] name Name of the file.
- Returns:
- True if the specified file exists.
Definition at line 377 of file PCMFileSystemManager.cpp.
References existsFile(), and mAppDirectory.
Referenced by findDataDirectory().
Here is the call graph for this function:

bool ParCompMark::FileSystemManager::existsDataFile | ( | const char * | name | ) | const [virtual] |
Return true if the specified file exists in the data directory.
- Parameters:
-
[in] name Name of the file.
- Returns:
- True if the specified file exists.
Definition at line 384 of file PCMFileSystemManager.cpp.
References existsFile(), and mDataDirectory.
Referenced by squirrelGlue().
Here is the call graph for this function:

bool ParCompMark::FileSystemManager::existsDirectory | ( | const std::string & | name | ) | const [virtual] |
Return true if the specified directory exists.
- Parameters:
-
[in] name Name of the directory.
- Returns:
- True if the specified directory exists.
Definition at line 162 of file PCMFileSystemManager.cpp.
References _replaceHomeChar().
Referenced by createDirectory(), and findDataDirectory().
Here is the call graph for this function:

bool ParCompMark::FileSystemManager::existsFile | ( | const std::string & | name | ) | const [virtual] |
Return true if the specified file exists.
- Parameters:
-
[in] name Name of the file.
- Returns:
- True if the specified file exists.
Definition at line 333 of file PCMFileSystemManager.cpp.
References _replaceHomeChar(), errno, and Except.
Referenced by existsAppFile(), existsDataFile(), findLibraryPath(), openFileC(), and openFileCpp().
Here is the call graph for this function:

bool ParCompMark::FileSystemManager::existsLibrary | ( | const std::string & | library, | |
const std::vector< std::string > & | additionalPaths = std::vector< std::string >() | |||
) | const [virtual] |
Returns true if the library can be found.
- Parameters:
-
[in] library Name of the library file. [in] additionalPaths Array if additional paths to seach
- Returns:
- The library can be found.
Definition at line 239 of file PCMFileSystemManager.cpp.
References findLibraryPath().
Here is the call graph for this function:

void ParCompMark::FileSystemManager::finalize | ( | ) | [virtual] |
Finalize the file system manager.
Definition at line 152 of file PCMFileSystemManager.cpp.
References Assert, ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), and mInitialized.
Referenced by ~FileSystemManager().
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::findDataDirectory | ( | ) | const [virtual] |
Find data directory path.
Throw exception if not found.
- Returns:
- Path of data directory.
Definition at line 216 of file PCMFileSystemManager.cpp.
References _translateToAbsolutePath(), Except, existsAppFile(), existsDirectory(), mAppDirectory, and mIniFile.
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::findLibraryPath | ( | const std::string & | library, | |
const std::vector< std::string > & | additionalPaths = std::vector< std::string >() | |||
) | const [virtual] |
Find path for library.
Throw exception if not found.
- Parameters:
-
[in] library Name of the library file. [in] additionalPaths Array if additional paths to seach
- Returns:
- Path of the library.
Definition at line 256 of file PCMFileSystemManager.cpp.
References _translateToAbsolutePath(), Except, existsFile(), ParCompMark::Application::getInstance(), and ParCompMark::StringConverter::tokenize().
Referenced by existsLibrary().
Here is the call graph for this function:
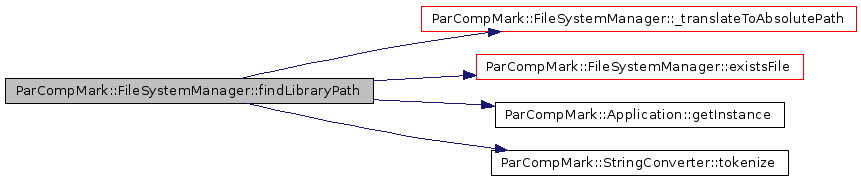
const std::string & ParCompMark::FileSystemManager::getAppDirectory | ( | ) | const [inline] |
Getter of mAppDirectory.
Returns value of mAppDirectory.
- Returns:
- The value of mAppDirectory
Definition at line 599 of file PCMFileSystemManager.h.
Referenced by ParCompMark::ConfigOptions::saveToIniFile().
const std::string & ParCompMark::FileSystemManager::getCurrentDirectory | ( | ) | const [inline] |
Getter of mCurrentDirectory.
Returns value of mCurrentDirectory.
- Returns:
- The value of mCurrentDirectory
Definition at line 634 of file PCMFileSystemManager.h.
const std::string & ParCompMark::FileSystemManager::getDataDirectory | ( | ) | const [inline] |
Getter of mDataDirectory.
Returns value of mDataDirectory.
- Returns:
- The value of mDataDirectory
Definition at line 613 of file PCMFileSystemManager.h.
const std::string & ParCompMark::FileSystemManager::getHomeDirectory | ( | ) | const [inline] |
Getter of mHomeDirectory.
Returns value of mHomeDirectory.
- Returns:
- The value of mHomeDirectory
Definition at line 627 of file PCMFileSystemManager.h.
const std::string & ParCompMark::FileSystemManager::getIniFile | ( | ) | const [inline] |
Getter of mIniFile.
Returns value of mIniFile.
- Returns:
- The value of mIniFile
Definition at line 641 of file PCMFileSystemManager.h.
const bool & ParCompMark::FileSystemManager::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 592 of file PCMFileSystemManager.h.
FileSystemManager * ParCompMark::FileSystemManager::getInstance | ( | ) | [static] |
Gets the instance of the singleton class.
Reimplemented for squirrel usage.
- Returns:
- Instance of the class
Reimplemented from ParCompMark::Singleton< T >.
Definition at line 98 of file PCMFileSystemManager.cpp.
Referenced by ParCompMark::PluginManager::_loadPlugin(), ParCompMark::Application::commanderOperation(), ParCompMark::Application::createVirtualMachines(), ParCompMark::Application::finalize(), ParCompMark::Logger::initialize(), ParCompMark::Host::initialize(), ParCompMark::Application::initialize(), ParCompMark::Application::loadDynamicScript(), ParCompMark::ConfigOptions::loadFromIniFile(), ParCompMark::Application::loadLowLevelScript(), ParCompMark::PluginManager::loadPlugins(), ParCompMark::PluginManager::loadPlugins_old(), ParCompMark::Application::loadScenarioScript(), readTextFile(), ParCompMark::HostInfo::refreshCPUs(), ParCompMark::ConfigOptions::saveToIniFile(), ParCompMark::Application::setFromConfigOptions(), squirrelGlue(), and ParCompMark::Process::threadInitialize().
std::string ParCompMark::FileSystemManager::getPathDataFile | ( | const std::string & | name | ) | const [virtual] |
Get full path of the specified data file.
- Parameters:
-
[in] name Name of the file.
- Returns:
- Full path for the data file.
Definition at line 484 of file PCMFileSystemManager.cpp.
References mDataDirectory.
Referenced by ParCompMark::PluginManager::_loadPlugin().
void ParCompMark::FileSystemManager::initialize | ( | ) | [virtual] |
Initialize the file system manager.
Definition at line 129 of file PCMFileSystemManager.cpp.
References _createAppDirectory(), _findHomeDirectory(), _replaceHomeChar(), Assert, ParCompMark::Application::getEnvironmentVariable(), ParCompMark::Application::getInstance(), mAppDirectory, mCurrentDirectory, and mInitialized.
Referenced by ParCompMark::Logger::initialize(), and ParCompMark::Application::initialize().
Here is the call graph for this function:

std::list< std::string > ParCompMark::FileSystemManager::listDataDirectory | ( | const std::string & | directory | ) | const [virtual] |
List files in the specified directory relative to the data directory.
Throw exception if not found.
- Parameters:
-
[in] directory Name of the directory to scan.
- Returns:
- List of files in the specified directory (relative to the data directory).
Definition at line 322 of file PCMFileSystemManager.cpp.
References listDirectory(), and mDataDirectory.
Referenced by ParCompMark::Application::createVirtualMachines(), and ParCompMark::PluginManager::loadPlugins().
Here is the call graph for this function:

std::list< std::string > ParCompMark::FileSystemManager::listDirectory | ( | const std::string & | directory | ) | const [virtual] |
List files in the specified directory.
Throw exception if not found.
- Parameters:
-
[in] directory Name of the directory to scan.
- Returns:
- List of files in the specified directory.
Definition at line 295 of file PCMFileSystemManager.cpp.
References Except.
Referenced by listDataDirectory().
FileSystemManager::CFilePointer ParCompMark::FileSystemManager::openAppFileC | ( | const std::string & | name, | |
const FileOperation & | operation = FileSystemManager::READ | |||
) | const [virtual] |
Open file for reading or writing in the application directory and return a C-style file pointer.
- Parameters:
-
[in] name Name of the file. [in] operation File operation.
- Returns:
- Smart pointer on a C-style file pointer.
Definition at line 421 of file PCMFileSystemManager.cpp.
References mAppDirectory, and openFileC().
Referenced by ParCompMark::Logger::initialize().
Here is the call graph for this function:

FileSystemManager::CppFilePointer ParCompMark::FileSystemManager::openAppFileCpp | ( | const std::string & | name, | |
const FileOperation & | operation = FileSystemManager::READ | |||
) | const [virtual] |
Open file for reading or writing in the application directory and return a fstream object.
- Parameters:
-
[in] name Name of the file. [in] operation File operation.
- Returns:
- Smart pointer on a fstream object.
Definition at line 466 of file PCMFileSystemManager.cpp.
References mAppDirectory, and openFileCpp().
Here is the call graph for this function:

FileSystemManager::CFilePointer ParCompMark::FileSystemManager::openDataFileC | ( | const std::string & | name, | |
const FileOperation & | operation = FileSystemManager::READ | |||
) | const [virtual] |
Open file for reading or writing in the application data directory and return a C-style file pointer.
- Parameters:
-
[in] name Name of the file. [in] operation File operation.
- Returns:
- Smart pointer on a C-style file pointer.
Definition at line 429 of file PCMFileSystemManager.cpp.
References mDataDirectory, and openFileC().
Here is the call graph for this function:

FileSystemManager::CppFilePointer ParCompMark::FileSystemManager::openDataFileCpp | ( | const std::string & | name, | |
const FileOperation & | operation = FileSystemManager::READ | |||
) | const [virtual] |
Open file for reading or writing in the application data directory and return a fstream object.
- Parameters:
-
[in] name Name of the file. [in] operation File operation.
- Returns:
- Smart pointer on a fstream object.
Definition at line 475 of file PCMFileSystemManager.cpp.
References mDataDirectory, and openFileCpp().
Here is the call graph for this function:

FileSystemManager::CFilePointer ParCompMark::FileSystemManager::openFileC | ( | const std::string & | name, | |
const FileOperation & | operation = FileSystemManager::READ | |||
) | const [virtual] |
Open file for reading or writing and return a C-style file pointer.
- Parameters:
-
[in] name Name of the file. [in] operation File operation.
- Returns:
- Smart pointer on a C-style file pointer.
Definition at line 391 of file PCMFileSystemManager.cpp.
References APPEND, Assert, existsFile(), READ, and WRITE.
Referenced by openAppFileC(), openDataFileC(), and ParCompMark::HostInfo::refreshCPUs().
Here is the call graph for this function:

FileSystemManager::CppFilePointer ParCompMark::FileSystemManager::openFileCpp | ( | const std::string & | name, | |
const FileOperation & | operation = FileSystemManager::READ | |||
) | const [virtual] |
Open file for reading or writing and return a fstream object.
- Parameters:
-
[in] name Name of the file. [in] operation File operation.
- Returns:
- Smart pointer on a fstream object.
Definition at line 437 of file PCMFileSystemManager.cpp.
References APPEND, Except, existsFile(), READ, and WRITE.
Referenced by openAppFileCpp(), openDataFileCpp(), and readTextFile().
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::readAppTextFile | ( | const std::string & | name | ) | const [virtual] |
Read contents of a text file in the application directory.
- Parameters:
-
[in] name Name of the file.
- Returns:
- Content of the file.
Definition at line 512 of file PCMFileSystemManager.cpp.
References mAppDirectory, and readTextFile().
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::readDataTextFile | ( | const std::string & | name | ) | const [virtual] |
Read contents of a text file in the application data directory.
- Parameters:
-
[in] name Name of the file.
- Returns:
- Content of the file.
Definition at line 519 of file PCMFileSystemManager.cpp.
References mDataDirectory, and readTextFile().
Referenced by ParCompMark::Application::loadDynamicScript(), ParCompMark::Application::loadLowLevelScript(), and ParCompMark::Application::loadScenarioScript().
Here is the call graph for this function:

std::string ParCompMark::FileSystemManager::readTextFile | ( | const std::string & | name | ) | const [virtual] |
Read contents of a text file.
- Parameters:
-
[in] name Name of the file.
- Returns:
- Content of the file.
Definition at line 491 of file PCMFileSystemManager.cpp.
References closeFile(), getInstance(), openFileCpp(), and READ.
Referenced by readAppTextFile(), and readDataTextFile().
Here is the call graph for this function:

void ParCompMark::FileSystemManager::setAppDirectory | ( | const std::string & | appdirectory | ) | [inline] |
Setter of mAppDirectory.
Sets value of mAppDirectory.
- Parameters:
-
[in] appdirectory The value of mAppDirectory
Definition at line 606 of file PCMFileSystemManager.h.
void ParCompMark::FileSystemManager::setDataDirectory | ( | const std::string & | datadirectory | ) | [inline] |
Setter of mDataDirectory.
Sets value of mDataDirectory.
- Parameters:
-
[in] datadirectory The value of mDataDirectory
Definition at line 620 of file PCMFileSystemManager.h.
Referenced by ParCompMark::Application::setFromConfigOptions().
static void ParCompMark::FileSystemManager::squirrelGlue | ( | ) | [inline, static] |
Static glue code method for Squirrel-C++ binding.
This method should be call from each Squirrel virtual machines to generate the proper Squirrel glue code. To content of this method is fully autogenerated in the header implementation file, you should not modify it.
Definition at line 100 of file PCMFileSystemManager.h.
References existsDataFile(), and getInstance().
Referenced by ParCompMark::squirrelClassBindings().
Here is the call graph for this function:

Member Data Documentation
std::string ParCompMark::FileSystemManager::mAppDirectory [protected] |
Path of user-level application directory.
- Remarks:
- This is own attribute of this class.
Definition at line 190 of file PCMFileSystemManager.h.
Referenced by _createAppDirectory(), existsAppFile(), FileSystemManager(), findDataDirectory(), initialize(), openAppFileC(), openAppFileCpp(), and readAppTextFile().
const u32 ParCompMark::FileSystemManager::MAX_PATH = 1024 [static] |
Maximal number of chars in paths.
- Remarks:
- This is own attribute of this class.
Definition at line 158 of file PCMFileSystemManager.h.
Referenced by _translateToAbsolutePath().
std::string ParCompMark::FileSystemManager::mCurrentDirectory [protected] |
Path of current directory.
- Remarks:
- This is own attribute of this class.
Definition at line 211 of file PCMFileSystemManager.h.
Referenced by _translateToAbsolutePath(), FileSystemManager(), and initialize().
std::string ParCompMark::FileSystemManager::mDataDirectory [protected] |
Path of application data directory.
- Remarks:
- This is own attribute of this class.
Definition at line 197 of file PCMFileSystemManager.h.
Referenced by existsDataFile(), FileSystemManager(), getPathDataFile(), listDataDirectory(), openDataFileC(), openDataFileCpp(), and readDataTextFile().
std::string ParCompMark::FileSystemManager::mHomeDirectory [protected] |
Home directory of the user.
- Remarks:
- This is own attribute of this class.
Definition at line 204 of file PCMFileSystemManager.h.
Referenced by _findHomeDirectory(), _replaceHomeChar(), and FileSystemManager().
std::string ParCompMark::FileSystemManager::mIniFile [protected] |
Name of user-level ini file.
- Remarks:
- This is own attribute of this class.
Definition at line 218 of file PCMFileSystemManager.h.
Referenced by FileSystemManager(), and findDataDirectory().
bool ParCompMark::FileSystemManager::mInitialized [protected] |
The application is initialized.
- Remarks:
- This is own attribute of this class.
Definition at line 183 of file PCMFileSystemManager.h.
Referenced by FileSystemManager(), finalize(), initialize(), and ~FileSystemManager().
const std::string ParCompMark::FileSystemManager::PATH_SEPARATOR = "/" [static] |
Path separator on the host OS.
- Remarks:
- This is own attribute of this class.
Definition at line 165 of file PCMFileSystemManager.h.
Referenced by _translateToAbsolutePath().
The documentation for this class was generated from the following files: