ParCompMark::Thread Class Reference
#include <PCMThread.h>
Inheritance diagram for ParCompMark::Thread:
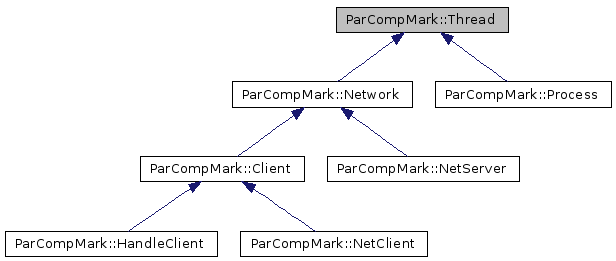
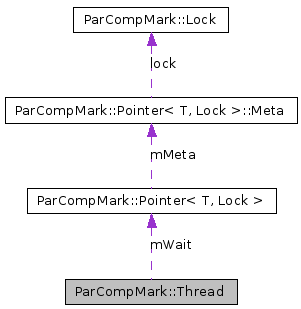
Detailed Description
This is a unique thread class.
Definition at line 60 of file PCMThread.h.
Methods | |
virtual void | initThread (const u32 &iterationNumber=0, const u32 &expectedFPS=0, const bool &joinable=false, const bool &waitThread=false) |
Start thread. | |
virtual void | threadInitialize () |
Some init step as thread. | |
virtual void | threadFinalize () |
Some finitiate step as thread. | |
virtual void | startThread () |
Start thread. | |
virtual void | joinThread () |
Join thread. | |
virtual void | shutDownThread () |
Immediately stop the thread. | |
virtual void | stopThread () |
Request thread shut down. | |
virtual void | go () |
Go forward from wait. | |
virtual void | thread () |
This is the thread method containing one or more (or inifinite) iterations. | |
virtual bool | iteration () |
One iteration step. | |
virtual void | task () |
It is the task of the thread. | |
virtual void | wait () |
Wait for something. | |
Class methods | |
static void | yield () |
Yield current thread. | |
static Real | getUSTime () |
Get system time in us. | |
static void * | entryPoint (void *arg) |
Static entry point for the thread. | |
Public Types | |
typedef ParCompMark::Pointer< bool, Mutex > | BoolPointer |
Type for pointer to an int. | |
Public Member Functions | |
Constructors & destructor | |
Thread (const std::string &name) | |
Thread constructor. | |
virtual | ~Thread () |
The destructor. | |
Getters & setters | |
const std::string & | getThreadName () const |
Getter of mThreadName. | |
const Thread::BoolPointer & | getWait () const |
Getter of mWait. | |
const bool & | getStopRequested () const |
Getter of mStopRequested. | |
const bool & | getJoinable () const |
Getter of mJoinable. | |
const bool & | getWaitThread () const |
Getter of mWaitThread. | |
const bool & | getRunning () const |
Getter of mRunning. | |
const u32 & | getCurrentFPS () const |
Getter of mCurrentFPS. | |
const u32 & | getExpectedFPS () const |
Getter of mExpectedFPS. | |
const u32 & | getIterationNumber () const |
Getter of mIterationNumber. | |
Protected Attributes | |
Variables | |
std::string | mThreadName |
Name of the thread. | |
BoolPointer | mWait |
Indicates that thread waits or not. | |
bool | mStopRequested |
The thread is requested to stop. | |
bool | mJoinable |
The thread is created as joinable or not. | |
bool | mWaitThread |
When class destructor call, wait thread or not. | |
pthread_t | mThread |
Thread handle. | |
pthread_cond_t | mCondition |
Condition. | |
pthread_mutex_t | mConditionMutex |
Condition. | |
bool | mRunning |
The thread is running. | |
u32 | mCurrentFPS |
The thread is running. | |
u32 | mExpectedFPS |
The thread is running. | |
u32 | mIterationNumber |
Iteration number of task. |
Member Typedef Documentation
typedef ParCompMark::Pointer< bool, Mutex > ParCompMark::Thread::BoolPointer |
Constructor & Destructor Documentation
ParCompMark::Thread::Thread | ( | const std::string & | name | ) |
Thread constructor.
- Parameters:
-
[in] name Name of the thread.
Definition at line 38 of file PCMThread.cpp.
References Except, ParCompMark::Pointer< T, Lock >::getPtr(), mCondition, mConditionMutex, mCurrentFPS, mExpectedFPS, mIterationNumber, mJoinable, mRunning, mStopRequested, mThread, mThreadName, and mWait.
Here is the call graph for this function:

ParCompMark::Thread::~Thread | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 77 of file PCMThread.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), mCondition, mConditionMutex, mRunning, mThreadName, and shutDownThread().
Here is the call graph for this function:

Member Function Documentation
void * ParCompMark::Thread::entryPoint | ( | void * | arg | ) | [static, protected] |
Static entry point for the thread.
- Parameters:
-
[in] arg This is only for thread.
- Returns:
- This is only for thread.
Definition at line 95 of file PCMThread.cpp.
References ParCompMark::Logger::DEBUG, Except, ParCompMark::Singleton< T >::getInstance(), mRunning, mThreadName, and thread().
Referenced by startThread().
Here is the call graph for this function:

const u32 & ParCompMark::Thread::getCurrentFPS | ( | ) | const [inline] |
Getter of mCurrentFPS.
Returns value of mCurrentFPS.
- Returns:
- The value of mCurrentFPS
Definition at line 470 of file PCMThread.h.
const u32 & ParCompMark::Thread::getExpectedFPS | ( | ) | const [inline] |
Getter of mExpectedFPS.
Returns value of mExpectedFPS.
- Returns:
- The value of mExpectedFPS
Definition at line 477 of file PCMThread.h.
const u32 & ParCompMark::Thread::getIterationNumber | ( | ) | const [inline] |
Getter of mIterationNumber.
Returns value of mIterationNumber.
- Returns:
- The value of mIterationNumber
Definition at line 484 of file PCMThread.h.
const bool & ParCompMark::Thread::getJoinable | ( | ) | const [inline] |
Getter of mJoinable.
Returns value of mJoinable.
- Returns:
- The value of mJoinable
Definition at line 449 of file PCMThread.h.
const bool & ParCompMark::Thread::getRunning | ( | ) | const [inline] |
Getter of mRunning.
Returns value of mRunning.
- Returns:
- The value of mRunning
Definition at line 463 of file PCMThread.h.
const bool & ParCompMark::Thread::getStopRequested | ( | ) | const [inline] |
Getter of mStopRequested.
Returns value of mStopRequested.
- Returns:
- The value of mStopRequested
Definition at line 442 of file PCMThread.h.
const std::string & ParCompMark::Thread::getThreadName | ( | ) | const [inline] |
Getter of mThreadName.
Returns value of mThreadName.
- Returns:
- The value of mThreadName
Definition at line 428 of file PCMThread.h.
Real ParCompMark::Thread::getUSTime | ( | ) | [inline, static] |
const Thread::BoolPointer & ParCompMark::Thread::getWait | ( | ) | const [inline] |
Getter of mWait.
Returns value of mWait.
- Returns:
- The value of mWait
Definition at line 435 of file PCMThread.h.
const bool & ParCompMark::Thread::getWaitThread | ( | ) | const [inline] |
Getter of mWaitThread.
Returns value of mWaitThread.
- Returns:
- The value of mWaitThread
Definition at line 456 of file PCMThread.h.
void ParCompMark::Thread::go | ( | ) | [virtual] |
Go forward from wait.
Definition at line 242 of file PCMThread.cpp.
References Except, mCondition, and mConditionMutex.
Referenced by ParCompMark::Process::task().
void ParCompMark::Thread::initThread | ( | const u32 & | iterationNumber = 0 , |
|
const u32 & | expectedFPS = 0 , |
|||
const bool & | joinable = false , |
|||
const bool & | waitThread = false | |||
) | [virtual] |
Start thread.
- Parameters:
-
[in] iterationNumber How much is the task running. Zero means nan. [in] expectedFPS Expected FPS. [in] joinable Joinable? [in] waitThread Wait when destroy class?
Definition at line 126 of file PCMThread.cpp.
References Assert, mExpectedFPS, mIterationNumber, mJoinable, and mWaitThread.
Referenced by ParCompMark::NetServer::initialize(), ParCompMark::NetClient::initialize(), ParCompMark::NetServer::task(), ParCompMarkTest::TestThread::test_destructor(), ParCompMarkTest::TestThread::test_initThread_cu32_cu32_cbool_cbool(), ParCompMarkTest::TestThread::test_joinThread(), ParCompMarkTest::TestThread::test_startThread(), and ParCompMarkTest::TestThread::test_stopThread().
bool ParCompMark::Thread::iteration | ( | ) | [inline, protected, virtual] |
One iteration step.
This method calls the overridable task method.
- Returns:
- If true, the thread is stopped.
Definition at line 517 of file PCMThread.h.
Referenced by thread().
void ParCompMark::Thread::joinThread | ( | ) | [virtual] |
Join thread.
Wait for its ending.
- Remarks:
- !!! You can join if the thread will stop (mIterationNumber != 0 or mStopRequested = true).
Definition at line 181 of file PCMThread.cpp.
References Assert, Except, mJoinable, mRunning, mThread, and mThreadName.
Referenced by ParCompMark::Application::startOperation().
void ParCompMark::Thread::shutDownThread | ( | ) | [virtual] |
Immediately stop the thread.
Definition at line 203 of file PCMThread.cpp.
References Assert, ParCompMark::Logger::DEBUG, Except, ParCompMark::Singleton< T >::getInstance(), mRunning, mStopRequested, mThread, mThreadName, and mWaitThread.
Referenced by ~Thread().
Here is the call graph for this function:

void ParCompMark::Thread::startThread | ( | ) | [virtual] |
Start thread.
Definition at line 152 of file PCMThread.cpp.
References Assert, ParCompMark::Logger::DEBUG, entryPoint(), ParCompMark::Singleton< T >::getInstance(), mJoinable, mRunning, mThread, and mThreadName.
Referenced by ParCompMark::Application::commanderOperation(), ParCompMark::Application::soldierOperation(), ParCompMark::Process::start(), ParCompMark::NetServer::task(), ParCompMarkTest::TestThread::test_joinThread(), ParCompMarkTest::TestThread::test_startThread(), and ParCompMarkTest::TestThread::test_stopThread().
Here is the call graph for this function:

void ParCompMark::Thread::stopThread | ( | ) | [virtual] |
Request thread shut down.
Definition at line 234 of file PCMThread.cpp.
References ParCompMark::Logger::DEBUG, ParCompMark::Singleton< T >::getInstance(), mStopRequested, and mThreadName.
Referenced by ParCompMark::Client::handleMessage(), ParCompMark::Process::task(), and ParCompMarkTest::TestThread::test_stopThread().
Here is the call graph for this function:

void ParCompMark::Thread::task | ( | ) | [protected, virtual] |
It is the task of the thread.
It have to be overiden.
- Remarks:
- This method should be abstact. It is not, because of unit testing of this class.
Reimplemented in ParCompMark::HandleClient, ParCompMark::NetClient, ParCompMark::NetServer, and ParCompMark::Process.
Definition at line 295 of file PCMThread.cpp.
References ParCompMark::Timer::sleep().
Here is the call graph for this function:

void ParCompMark::Thread::thread | ( | ) | [protected, virtual] |
This is the thread method containing one or more (or inifinite) iterations.
Definition at line 264 of file PCMThread.cpp.
References iteration(), mIterationNumber, threadFinalize(), threadInitialize(), and yield().
Referenced by entryPoint().
Here is the call graph for this function:
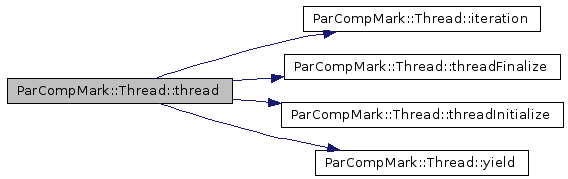
void ParCompMark::Thread::threadFinalize | ( | ) | [virtual] |
Some finitiate step as thread.
Reimplemented in ParCompMark::Process.
Definition at line 146 of file PCMThread.cpp.
Referenced by thread().
void ParCompMark::Thread::threadInitialize | ( | ) | [virtual] |
Some init step as thread.
Reimplemented in ParCompMark::Process.
Definition at line 140 of file PCMThread.cpp.
Referenced by thread().
void ParCompMark::Thread::wait | ( | ) | [protected, virtual] |
Wait for something.
Definition at line 305 of file PCMThread.cpp.
References Except, mCondition, and mConditionMutex.
Referenced by ParCompMark::NetServer::sendBroadcastMessage(), ParCompMark::Process::stop(), and ParCompMark::Process::task().
void ParCompMark::Thread::yield | ( | ) | [inline, static] |
Yield current thread.
Definition at line 495 of file PCMThread.h.
Referenced by thread(), and ParCompMark::Application::waitForOutput().
Member Data Documentation
pthread_cond_t ParCompMark::Thread::mCondition [protected] |
pthread_mutex_t ParCompMark::Thread::mConditionMutex [protected] |
u32 ParCompMark::Thread::mCurrentFPS [protected] |
The thread is running.
- Remarks:
- This is own attribute of this class.
Definition at line 157 of file PCMThread.h.
Referenced by Thread().
u32 ParCompMark::Thread::mExpectedFPS [protected] |
The thread is running.
Zero means no limit.
- Remarks:
- This is own attribute of this class.
Definition at line 164 of file PCMThread.h.
Referenced by initThread(), ParCompMarkTest::TestThread::test_initThread_cu32_cu32_cbool_cbool(), and Thread().
u32 ParCompMark::Thread::mIterationNumber [protected] |
Iteration number of task.
Zero means nan.
- Remarks:
- This is own attribute of this class.
Definition at line 171 of file PCMThread.h.
Referenced by initThread(), ParCompMarkTest::TestThread::test_initThread_cu32_cu32_cbool_cbool(), thread(), and Thread().
bool ParCompMark::Thread::mJoinable [protected] |
The thread is created as joinable or not.
- Remarks:
- This is own attribute of this class.
Definition at line 115 of file PCMThread.h.
Referenced by initThread(), joinThread(), startThread(), and Thread().
bool ParCompMark::Thread::mRunning [protected] |
The thread is running.
- Remarks:
- This is own attribute of this class.
Definition at line 150 of file PCMThread.h.
Referenced by entryPoint(), joinThread(), shutDownThread(), startThread(), Thread(), and ~Thread().
bool ParCompMark::Thread::mStopRequested [protected] |
The thread is requested to stop.
- Remarks:
- This is own attribute of this class.
Definition at line 108 of file PCMThread.h.
Referenced by shutDownThread(), stopThread(), and Thread().
pthread_t ParCompMark::Thread::mThread [protected] |
Thread handle.
- Remarks:
- This is own attribute of this class.
Definition at line 129 of file PCMThread.h.
Referenced by joinThread(), shutDownThread(), startThread(), and Thread().
std::string ParCompMark::Thread::mThreadName [protected] |
Name of the thread.
- Remarks:
- This is own attribute of this class.
Definition at line 94 of file PCMThread.h.
Referenced by entryPoint(), joinThread(), shutDownThread(), startThread(), stopThread(), Thread(), ParCompMark::Process::threadInitialize(), and ~Thread().
BoolPointer ParCompMark::Thread::mWait [protected] |
Indicates that thread waits or not.
- Remarks:
- This is own attribute of this class.
Definition at line 101 of file PCMThread.h.
Referenced by Thread().
bool ParCompMark::Thread::mWaitThread [protected] |
When class destructor call, wait thread or not.
- Remarks:
- This is own attribute of this class.
Definition at line 122 of file PCMThread.h.
Referenced by initThread(), and shutDownThread().
The documentation for this class was generated from the following files: