ParCompMark::CPU Class Reference
#include <PCMCPU.h>
Inheritance diagram for ParCompMark::CPU:
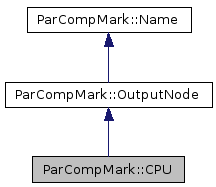
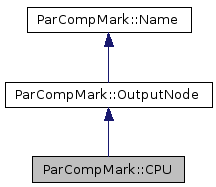
Detailed Description
Class contain CPU information.
Definition at line 60 of file PCMCPU.h.
Public Types | |
typedef ParCompMark::Pointer< CPU, Mutex > | Pointer |
Type for pointer on this class. | |
Public Member Functions | |
Constructors & destructor | |
CPU (const std::string &name, const OutputNode::NodeType &type) | |
Creates CPU node. | |
virtual | ~CPU () |
The destructor. | |
Getters & setters | |
const std::string & | getVendor () const |
Getter of mVendor. | |
void | setVendor (const std::string &vendor) |
Setter of mVendor. | |
const std::string & | getModel () const |
Getter of mModel. | |
void | setModel (const std::string &model) |
Setter of mModel. | |
const Real & | getClock () const |
Getter of mClock. | |
void | setClock (const Real &clock) |
Setter of mClock. | |
const u32 & | getCache () const |
Getter of mCache. | |
void | setCache (const u32 &cache) |
Setter of mCache. | |
const Real & | getBogomips () const |
Getter of mBogomips. | |
void | setBogomips (const Real &bogomips) |
Setter of mBogomips. | |
const std::string & | getFlags () const |
Getter of mFlags. | |
void | setFlags (const std::string &flags) |
Setter of mFlags. | |
Methods | |
virtual void | refreshData () |
Refresh CPU datas. | |
Static Public Member Functions | |
Class methods | |
static CPU::Pointer | parseXML (TiXmlElement *&tiCPU) |
Creates a CPU class instance based on xml. | |
Protected Attributes | |
Variables | |
std::string | mVendor |
Name of the vendor of the CPU. | |
std::string | mModel |
Name of the CPU model. | |
Real | mClock |
CPU clock in MHz. | |
u32 | mCache |
CPU cache in KB. | |
Real | mBogomips |
BogoMIPS value for the CPU. | |
std::string | mFlags |
CPU flags. |
Member Typedef Documentation
typedef ParCompMark::Pointer< CPU, Mutex > ParCompMark::CPU::Pointer |
Constructor & Destructor Documentation
ParCompMark::CPU::CPU | ( | const std::string & | name, | |
const OutputNode::NodeType & | type | |||
) |
ParCompMark::CPU::~CPU | ( | ) | [virtual] |
Member Function Documentation
const Real & ParCompMark::CPU::getBogomips | ( | ) | const [inline] |
const u32 & ParCompMark::CPU::getCache | ( | ) | const [inline] |
const Real & ParCompMark::CPU::getClock | ( | ) | const [inline] |
const std::string & ParCompMark::CPU::getFlags | ( | ) | const [inline] |
const std::string & ParCompMark::CPU::getModel | ( | ) | const [inline] |
const std::string & ParCompMark::CPU::getVendor | ( | ) | const [inline] |
CPU::Pointer ParCompMark::CPU::parseXML | ( | TiXmlElement *& | tiCPU | ) | [static] |
Creates a CPU class instance based on xml.
- Parameters:
-
[out] tiCPU Description of CPU.
- Returns:
- Returns the pointer of the CPU class instance created based on the xml
Definition at line 72 of file PCMCPU.cpp.
References CPU(), ParCompMark::OutputNode::DEFINITION, ParCompMark::OutputNode::INFORMATION, ParCompMark::OutputNode::REFERENCE, and ParCompMark::OutputNode::STATISTICS.
Referenced by ParCompMark::HostInfo::parseXML().
Here is the call graph for this function:

void ParCompMark::CPU::refreshData | ( | ) | [virtual] |
Refresh CPU datas.
Reimplemented from ParCompMark::OutputNode.
Definition at line 163 of file PCMCPU.cpp.
void ParCompMark::CPU::setBogomips | ( | const Real & | bogomips | ) | [inline] |
void ParCompMark::CPU::setCache | ( | const u32 & | cache | ) | [inline] |
void ParCompMark::CPU::setClock | ( | const Real & | clock | ) | [inline] |
void ParCompMark::CPU::setFlags | ( | const std::string & | flags | ) | [inline] |
void ParCompMark::CPU::setModel | ( | const std::string & | model | ) | [inline] |
void ParCompMark::CPU::setVendor | ( | const std::string & | vendor | ) | [inline] |
Member Data Documentation
Real ParCompMark::CPU::mBogomips [protected] |
u32 ParCompMark::CPU::mCache [protected] |
Real ParCompMark::CPU::mClock [protected] |
std::string ParCompMark::CPU::mFlags [protected] |
std::string ParCompMark::CPU::mModel [protected] |
std::string ParCompMark::CPU::mVendor [protected] |
The documentation for this class was generated from the following files: