ParCompMark::Buffer Class Reference
#include <PCMBuffer.h>
Collaboration diagram for ParCompMark::Buffer:
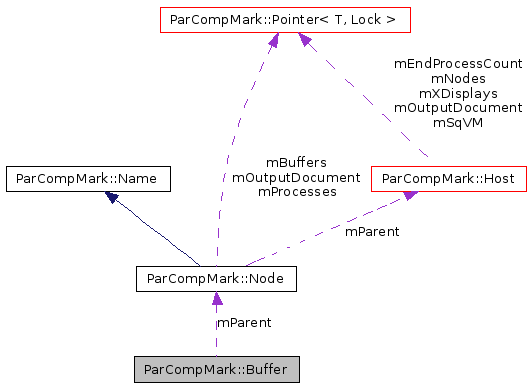
Detailed Description
Grapics memory buffer.Buffer object can store either colour and depth information of an image (PC result) or a fragment of an image (PC framelet).
Definition at line 67 of file PCMBuffer.h.
Methods | |
virtual void | initialize () |
Initialize the buffer. | |
virtual void | finalize () |
Finalize the buffer. | |
virtual void | saveToFile (const std::string &filename) |
Save the image in the buffer to the specified file. | |
virtual void | freeBuffers () |
Deallocate mColour and mDepth buffers. | |
Public Types | |
typedef Pointer< Buffer, Mutex > | Pointer |
Type for pointer on this class. | |
Public Member Functions | |
Constructors & destructor | |
Buffer () | |
Default constructor for Squirrel compatibility. | |
Buffer (const PCuint &left, const PCuint &top, const PCuint &width, const PCuint &height, const PCuint &depthFormat, Node *parent) | |
Create a buffer by initializing its parameters. | |
virtual | ~Buffer () |
The destructor. | |
Getters & setters | |
Node * | getParent () const |
Getter of mParent. | |
const PCuint & | getLeft () const |
Getter of mLeft. | |
void | setLeft (const PCuint left) |
Setter of mLeft. | |
const PCuint & | getTop () const |
Getter of mTop. | |
void | setTop (const PCuint top) |
Setter of mTop. | |
const PCuint & | getWidth () const |
Getter of mWidth. | |
void | setWidth (const PCuint width) |
Setter of mWidth. | |
const PCuint & | getHeight () const |
Getter of mHeight. | |
void | setHeight (const PCuint height) |
Setter of mHeight. | |
const bool & | getOwnPointers () const |
Getter of mOwnPointers. | |
PCuint * | getColour () |
Getter of mColour. | |
void | setColour (const PCuint *colour) |
Setter of mColour. | |
void * | getDepth () |
Getter of mDepth. | |
void | setDepth (const void *depth) |
Setter of mDepth. | |
const PCint & | getDepthFormat () const |
Getter of mDepthFormat. | |
void | setDepthFormat (const PCint depthformat) |
Setter of mDepthFormat. | |
const PCint & | getOutputRowPixel () const |
Getter of mOutputRowPixel. | |
void | setOutputRowPixel (const PCint &outputrowpixel) |
Setter of mOutputRowPixel. | |
const bool & | getInitialized () const |
Getter of mInitialized. | |
Static Public Member Functions | |
Scripting binding | |
static void | squirrelGlue () |
Static glue code method for Squirrel-C++ binding. | |
Protected Attributes | |
Variables | |
Node * | mParent |
Parent node of the buffer. | |
PCuint | mLeft |
PCuint | mTop |
PCuint | mWidth |
PCuint | mHeight |
bool | mOwnPointers |
The Buffer is responsible for deallocation of mColour and mDepth. | |
PCuint * | mColour |
Colour information buffer (RGBA32 or BGRA32 coded). | |
void * | mDepth |
Depth information buffer (the coding is unknown). | |
PCint | mDepthFormat |
Depth format. | |
PCint | mOutputRowPixel |
If the output is not the whole frame, this is the remainde pixels in a row. | |
bool | mInitialized |
The buffer is initialized. |
Member Typedef Documentation
typedef Pointer< Buffer, Mutex > ParCompMark::Buffer::Pointer |
Constructor & Destructor Documentation
ParCompMark::Buffer::Buffer | ( | ) |
Default constructor for Squirrel compatibility.
Calling this constructor always raises an exception.
Definition at line 49 of file PCMBuffer.cpp.
References Except.
ParCompMark::Buffer::Buffer | ( | const PCuint & | left, | |
const PCuint & | top, | |||
const PCuint & | width, | |||
const PCuint & | height, | |||
const PCuint & | depthFormat, | |||
Node * | parent | |||
) |
Create a buffer by initializing its parameters.
- Parameters:
-
[in] left X offset of the image (it is 0 for whole images). [in] top Y offset of the image (it is 0 for whole images). [in] width Width of the image. [in] height Height of the image. [in] depthFormat Depth format used by this image. [in] parent Parent node
Definition at line 69 of file PCMBuffer.cpp.
References ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mColour, mDepth, mDepthFormat, mHeight, mInitialized, mLeft, mOutputRowPixel, mOwnPointers, mParent, mTop, mWidth, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

ParCompMark::Buffer::~Buffer | ( | ) | [virtual] |
The destructor.
This class has virtual destructor.
Definition at line 103 of file PCMBuffer.cpp.
References finalize(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mInitialized, mParent, and ParCompMark::Logger::NOTICE.
Here is the call graph for this function:

Member Function Documentation
void ParCompMark::Buffer::finalize | ( | ) | [virtual] |
Finalize the buffer.
Definition at line 143 of file PCMBuffer.cpp.
References Assert, ParCompMark::Logger::DEBUG, freeBuffers(), ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mInitialized, and mParent.
Referenced by ~Buffer().
Here is the call graph for this function:

void ParCompMark::Buffer::freeBuffers | ( | ) | [protected, virtual] |
Deallocate mColour and mDepth buffers.
Definition at line 187 of file PCMBuffer.cpp.
References Except, ParCompMark::Name::getName(), mColour, mDepth, mDepthFormat, mOwnPointers, mParent, and ParCompMark::StringConverter::toString().
Referenced by finalize().
Here is the call graph for this function:

PCuint * ParCompMark::Buffer::getColour | ( | ) | [inline] |
Getter of mColour.
Returns value of mColour.
- Returns:
- The value of mColour
Definition at line 529 of file PCMBuffer.h.
void * ParCompMark::Buffer::getDepth | ( | ) | [inline] |
Getter of mDepth.
Returns value of mDepth.
- Returns:
- The value of mDepth
Definition at line 548 of file PCMBuffer.h.
const PCint & ParCompMark::Buffer::getDepthFormat | ( | ) | const [inline] |
Getter of mDepthFormat.
Returns value of mDepthFormat.
- Returns:
- The value of mDepthFormat
Definition at line 567 of file PCMBuffer.h.
Referenced by squirrelGlue().
const PCuint & ParCompMark::Buffer::getHeight | ( | ) | const [inline] |
Getter of mHeight.
Returns value of mHeight.
- Returns:
- The value of mHeight
Definition at line 506 of file PCMBuffer.h.
Referenced by squirrelGlue().
const bool & ParCompMark::Buffer::getInitialized | ( | ) | const [inline] |
Getter of mInitialized.
Returns value of mInitialized.
- Returns:
- The value of mInitialized
Definition at line 599 of file PCMBuffer.h.
const PCuint & ParCompMark::Buffer::getLeft | ( | ) | const [inline] |
Getter of mLeft.
Returns value of mLeft.
- Returns:
- The value of mLeft
Definition at line 458 of file PCMBuffer.h.
Referenced by squirrelGlue().
const PCint & ParCompMark::Buffer::getOutputRowPixel | ( | ) | const [inline] |
Getter of mOutputRowPixel.
Returns value of mOutputRowPixel.
- Returns:
- The value of mOutputRowPixel
Definition at line 583 of file PCMBuffer.h.
const bool & ParCompMark::Buffer::getOwnPointers | ( | ) | const [inline] |
Getter of mOwnPointers.
Returns value of mOwnPointers.
- Returns:
- The value of mOwnPointers
Definition at line 522 of file PCMBuffer.h.
Node * ParCompMark::Buffer::getParent | ( | ) | const [inline] |
Getter of mParent.
Returns value of mParent.
- Returns:
- The value of mParent
Definition at line 451 of file PCMBuffer.h.
const PCuint & ParCompMark::Buffer::getTop | ( | ) | const [inline] |
Getter of mTop.
Returns value of mTop.
- Returns:
- The value of mTop
Definition at line 474 of file PCMBuffer.h.
Referenced by squirrelGlue().
const PCuint & ParCompMark::Buffer::getWidth | ( | ) | const [inline] |
Getter of mWidth.
Returns value of mWidth.
- Returns:
- The value of mWidth
Definition at line 490 of file PCMBuffer.h.
Referenced by squirrelGlue().
void ParCompMark::Buffer::initialize | ( | ) | [virtual] |
Initialize the buffer.
Definition at line 117 of file PCMBuffer.cpp.
References ParCompMark::Logger::DEBUG, Except, ParCompMark::Singleton< T >::getInstance(), ParCompMark::Name::getName(), mColour, mDepth, mDepthFormat, mHeight, mInitialized, mParent, mWidth, and ParCompMark::StringConverter::toString().
Here is the call graph for this function:
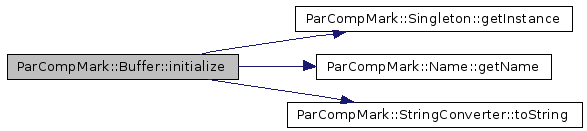
void ParCompMark::Buffer::saveToFile | ( | const std::string & | filename | ) | [virtual] |
void ParCompMark::Buffer::setColour | ( | const PCuint * | colour | ) | [inline] |
Setter of mColour.
Sets value of mColour.
- Parameters:
-
[in] colour The value of mColour
Definition at line 536 of file PCMBuffer.h.
void ParCompMark::Buffer::setDepth | ( | const void * | depth | ) | [inline] |
Setter of mDepth.
Sets value of mDepth.
- Parameters:
-
[in] depth The value of mDepth
Definition at line 555 of file PCMBuffer.h.
void ParCompMark::Buffer::setDepthFormat | ( | const PCint | depthformat | ) | [inline] |
Setter of mDepthFormat.
Sets value of mDepthFormat.
- Parameters:
-
[in] depthformat The value of mDepthFormat
Definition at line 574 of file PCMBuffer.h.
Referenced by squirrelGlue().
void ParCompMark::Buffer::setHeight | ( | const PCuint | height | ) | [inline] |
Setter of mHeight.
Sets value of mHeight.
- Parameters:
-
[in] height The value of mHeight
Definition at line 513 of file PCMBuffer.h.
Referenced by squirrelGlue().
void ParCompMark::Buffer::setLeft | ( | const PCuint | left | ) | [inline] |
Setter of mLeft.
Sets value of mLeft.
- Parameters:
-
[in] left The value of mLeft
Definition at line 465 of file PCMBuffer.h.
Referenced by squirrelGlue().
void ParCompMark::Buffer::setOutputRowPixel | ( | const PCint & | outputrowpixel | ) | [inline] |
Setter of mOutputRowPixel.
Sets value of mOutputRowPixel.
- Parameters:
-
[in] outputrowpixel The value of mOutputRowPixel
Definition at line 590 of file PCMBuffer.h.
void ParCompMark::Buffer::setTop | ( | const PCuint | top | ) | [inline] |
Setter of mTop.
Sets value of mTop.
- Parameters:
-
[in] top The value of mTop
Definition at line 481 of file PCMBuffer.h.
Referenced by squirrelGlue().
void ParCompMark::Buffer::setWidth | ( | const PCuint | width | ) | [inline] |
Setter of mWidth.
Sets value of mWidth.
- Parameters:
-
[in] width The value of mWidth
Definition at line 497 of file PCMBuffer.h.
Referenced by squirrelGlue().
static void ParCompMark::Buffer::squirrelGlue | ( | ) | [inline, static] |
Static glue code method for Squirrel-C++ binding.
This method should be call from each Squirrel virtual machines to generate the proper Squirrel glue code. To content of this method is fully autogenerated in the header implementation file, you should not modify it.
Definition at line 91 of file PCMBuffer.h.
References getDepthFormat(), getHeight(), getLeft(), getTop(), getWidth(), setDepthFormat(), setHeight(), setLeft(), setTop(), and setWidth().
Referenced by ParCompMark::squirrelClassBindings().
Here is the call graph for this function:
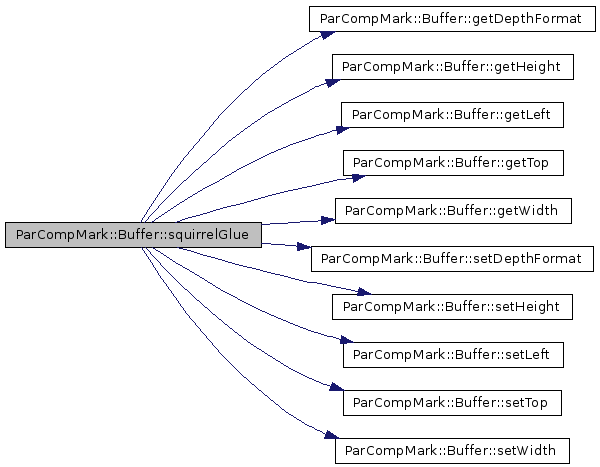
Member Data Documentation
PCuint* ParCompMark::Buffer::mColour [protected] |
Colour information buffer (RGBA32 or BGRA32 coded).
- Remarks:
- This is own attribute of this class.
Definition at line 171 of file PCMBuffer.h.
Referenced by Buffer(), freeBuffers(), initialize(), and saveToFile().
void* ParCompMark::Buffer::mDepth [protected] |
Depth information buffer (the coding is unknown).
- Remarks:
- This is own attribute of this class.
Definition at line 178 of file PCMBuffer.h.
Referenced by Buffer(), freeBuffers(), and initialize().
PCint ParCompMark::Buffer::mDepthFormat [protected] |
Depth format.
Needed for deallocating the buffer.
- Remarks:
- This is own attribute of this class.
Definition at line 185 of file PCMBuffer.h.
Referenced by Buffer(), freeBuffers(), and initialize().
PCuint ParCompMark::Buffer::mHeight [protected] |
- Remarks:
- This is own attribute of this class.
Definition at line 157 of file PCMBuffer.h.
Referenced by Buffer(), initialize(), and saveToFile().
bool ParCompMark::Buffer::mInitialized [protected] |
The buffer is initialized.
None of its attribute can be modified after the buffer is initialized except for mOutputRowPixel, mColour, and mDepth.
- Remarks:
- This is own attribute of this class.
Definition at line 199 of file PCMBuffer.h.
Referenced by Buffer(), finalize(), initialize(), and ~Buffer().
PCuint ParCompMark::Buffer::mLeft [protected] |
- Remarks:
- This is own attribute of this class.
Definition at line 136 of file PCMBuffer.h.
Referenced by Buffer().
PCint ParCompMark::Buffer::mOutputRowPixel [protected] |
If the output is not the whole frame, this is the remainde pixels in a row.
- Remarks:
- This is own attribute of this class.
Definition at line 192 of file PCMBuffer.h.
Referenced by Buffer().
bool ParCompMark::Buffer::mOwnPointers [protected] |
The Buffer is responsible for deallocation of mColour and mDepth.
- Remarks:
- This is own attribute of this class.
Definition at line 164 of file PCMBuffer.h.
Referenced by Buffer(), and freeBuffers().
Node* ParCompMark::Buffer::mParent [protected] |
Parent node of the buffer.
(Parent reference is standard pointer to avoid circular reference)
- Remarks:
- This attribute references an attribute.
Definition at line 129 of file PCMBuffer.h.
Referenced by Buffer(), finalize(), freeBuffers(), initialize(), and ~Buffer().
PCuint ParCompMark::Buffer::mTop [protected] |
- Remarks:
- This is own attribute of this class.
Definition at line 143 of file PCMBuffer.h.
Referenced by Buffer().
PCuint ParCompMark::Buffer::mWidth [protected] |
- Remarks:
- This is own attribute of this class.
Definition at line 150 of file PCMBuffer.h.
Referenced by Buffer(), initialize(), and saveToFile().
The documentation for this class was generated from the following files: