ParCompMark::Timer Class Reference
#include <PCMTimer.h>
Detailed Description
Collection of time handling methods.
Definition at line 61 of file PCMTimer.h.
Static Public Member Functions | |
Scripting binding | |
static void | squirrelGlue () |
Static glue code method for Squirrel-C++ binding. | |
Getters & setters | |
static const Real & | getTimeCorrection () |
Getter of mTimeCorrection. | |
static void | setTimeCorrection (const Real &timecorrection) |
Setter of mTimeCorrection. | |
static const Real & | getStartTime () |
Getter of mStartTime. | |
Class methods | |
static Real | getSystemTime () |
Return corrected system time in seconds. | |
static Real | getApplicationTime () |
Return (uncorrected) application time (getUncorrectedSystemTime() - mStartTime) in seconds. | |
static Real | getUncorrectedSystemTime () |
Return uncorrected system time in seconds. | |
static std::string | getTimeString (const bool µseconds=true, const bool &seconds=true, const bool &minutes=true, const bool &hours=true, const Real &correction=0.0) |
Return current time as a string. | |
static std::string | getDateString (const bool &days=true, const bool &months=true, const bool &years=true, const Real &correction=0.0) |
Return current date as a string. | |
static std::string | getTimeDateString (const bool µseconds=true, const bool &seconds=true, const bool &minutes=true, const bool &hours=true, const bool &days=true, const bool &months=true, const bool &years=true, const Real &correction=0.0) |
Return current time and date as a string. | |
static void | sleep (const Real time) |
Sleep process for the specified seconds. | |
Static Public Attributes | |
Class constants | |
static const Real | EPSILONDELAY = 0.000001 |
Constant for minimal time delay (1us). | |
Static Protected Attributes | |
Class variables | |
static Real | mTimeCorrection = 0.0 |
Time correction for this application according to the host of the commander mode application. | |
static Real | mStartTime = 0.0 |
(Uncorrected) time of the last write access to mTimeCorrection variable. |
Member Function Documentation
Real ParCompMark::Timer::getApplicationTime | ( | ) | [inline, static] |
Return (uncorrected) application time (getUncorrectedSystemTime() - mStartTime) in seconds.
- Returns:
- Corrected application time in seconds
Definition at line 316 of file PCMTimer.h.
Referenced by squirrelGlue().
std::string ParCompMark::Timer::getDateString | ( | const bool & | days = true , |
|
const bool & | months = true , |
|||
const bool & | years = true , |
|||
const Real & | correction = 0.0 | |||
) | [static] |
Return current date as a string.
- Parameters:
-
[in] days Flag indicates days to display in output [in] months Flag indicates months to display in output [in] years Flag indicates years to display in output [in] correction Time correction in seconds
- Returns:
- Current time in string format
Definition at line 62 of file PCMTimer.cpp.
References getTimeDateString().
Here is the call graph for this function:

const Real & ParCompMark::Timer::getStartTime | ( | ) | [inline, static] |
Getter of mStartTime.
Returns value of mStartTime.
- Returns:
- The value of mStartTime
Definition at line 298 of file PCMTimer.h.
Real ParCompMark::Timer::getSystemTime | ( | ) | [inline, static] |
Return corrected system time in seconds.
- Returns:
- Corrected time in seconds
Definition at line 309 of file PCMTimer.h.
Referenced by ParCompMark::OpenGLRenderingEngine::generateRandomTriangles(), getTimeDateString(), ParCompMark::Context::initialize(), ParCompMark::Process::Process(), ParCompMark::GLXRenderWindow::resetStatistics(), ParCompMark::Process::runningProcess(), squirrelGlue(), and ParCompMark::GLXRenderWindow::updateStatistics().
const Real & ParCompMark::Timer::getTimeCorrection | ( | ) | [inline, static] |
Getter of mTimeCorrection.
Returns value of mTimeCorrection.
- Returns:
- The value of mTimeCorrection
Definition at line 283 of file PCMTimer.h.
std::string ParCompMark::Timer::getTimeDateString | ( | const bool & | microseconds = true , |
|
const bool & | seconds = true , |
|||
const bool & | minutes = true , |
|||
const bool & | hours = true , |
|||
const bool & | days = true , |
|||
const bool & | months = true , |
|||
const bool & | years = true , |
|||
const Real & | correction = 0.0 | |||
) | [static] |
Return current time and date as a string.
- Parameters:
-
[in] microseconds Flag indicates microseconds to display in output [in] seconds Flag indicates seconds to display in output [in] minutes Flag indicates minutes to display in output [in] hours Flag indicates hours to display in output [in] days Flag indicates days to display in output [in] months Flag indicates months to display in output [in] years Flag indicates years to display in output [in] correction Time correction in seconds
- Returns:
- Current time in string format
Definition at line 70 of file PCMTimer.cpp.
References getSystemTime(), and ParCompMark::StringConverter::toString().
Referenced by getDateString(), getTimeString(), ParCompMark::Logger::initialize(), and ParCompMark::ConfigOptions::saveToIniFile().
Here is the call graph for this function:

std::string ParCompMark::Timer::getTimeString | ( | const bool & | microseconds = true , |
|
const bool & | seconds = true , |
|||
const bool & | minutes = true , |
|||
const bool & | hours = true , |
|||
const Real & | correction = 0.0 | |||
) | [static] |
Return current time as a string.
- Parameters:
-
[in] microseconds Flag indicates microseconds to display in output [in] seconds Flag indicates seconds to display in output [in] minutes Flag indicates minutes to display in output [in] hours Flag indicates hours to display in output [in] correction Time correction in seconds
- Returns:
- Current time in string format
Definition at line 53 of file PCMTimer.cpp.
References getTimeDateString().
Here is the call graph for this function:

Real ParCompMark::Timer::getUncorrectedSystemTime | ( | ) | [inline, static] |
Return uncorrected system time in seconds.
- Returns:
- Uncorrected time in seconds
Definition at line 323 of file PCMTimer.h.
Referenced by ParCompMark::Application::_start(), and ParCompMark::Client::handleMessage().
void ParCompMark::Timer::setTimeCorrection | ( | const Real & | timecorrection | ) | [inline, static] |
Setter of mTimeCorrection.
Sets value of mTimeCorrection.
- Parameters:
-
[in] timecorrection The value of mTimeCorrection
Definition at line 290 of file PCMTimer.h.
Referenced by ParCompMark::Host::initialize(), and ParCompMark::Application::initialize().
void ParCompMark::Timer::sleep | ( | const Real | time | ) | [inline, static] |
Sleep process for the specified seconds.
- Parameters:
-
[in] time Time to sleep
Definition at line 334 of file PCMTimer.h.
Referenced by ParCompMark::NetServer::buildCluster(), ParCompMark::Application::noUserInterface(), ParCompMark::Process::openRenderWindow(), ParCompMark::Application::processSquirrelCommandList(), squirrelGlue(), ParCompMark::Thread::task(), and ParCompMark::Application::waitForOutput().
static void ParCompMark::Timer::squirrelGlue | ( | ) | [inline, static] |
Static glue code method for Squirrel-C++ binding.
This method should be call from each Squirrel virtual machines to generate the proper Squirrel glue code. To content of this method is fully autogenerated in the header implementation file, you should not modify it.
Definition at line 85 of file PCMTimer.h.
References getApplicationTime(), getSystemTime(), and sleep().
Referenced by ParCompMark::squirrelClassBindings().
Here is the call graph for this function:
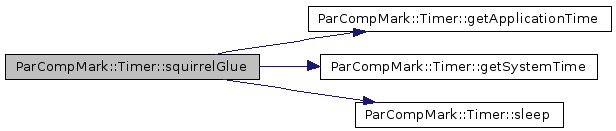
Member Data Documentation
const Real ParCompMark::Timer::EPSILONDELAY = 0.000001 [static] |
Constant for minimal time delay (1us).
- Remarks:
- This is own attribute of this class.
Definition at line 108 of file PCMTimer.h.
Referenced by ParCompMark::GLXRenderWindow::updateStatistics().
Real ParCompMark::Timer::mStartTime = 0.0 [static, protected] |
(Uncorrected) time of the last write access to mTimeCorrection variable.
- Remarks:
- This is own attribute of this class.
Definition at line 133 of file PCMTimer.h.
Real ParCompMark::Timer::mTimeCorrection = 0.0 [static, protected] |
Time correction for this application according to the host of the commander mode application.
- Remarks:
- This is own attribute of this class.
Definition at line 126 of file PCMTimer.h.
The documentation for this class was generated from the following files: